How to Import Certificates in PowerShell
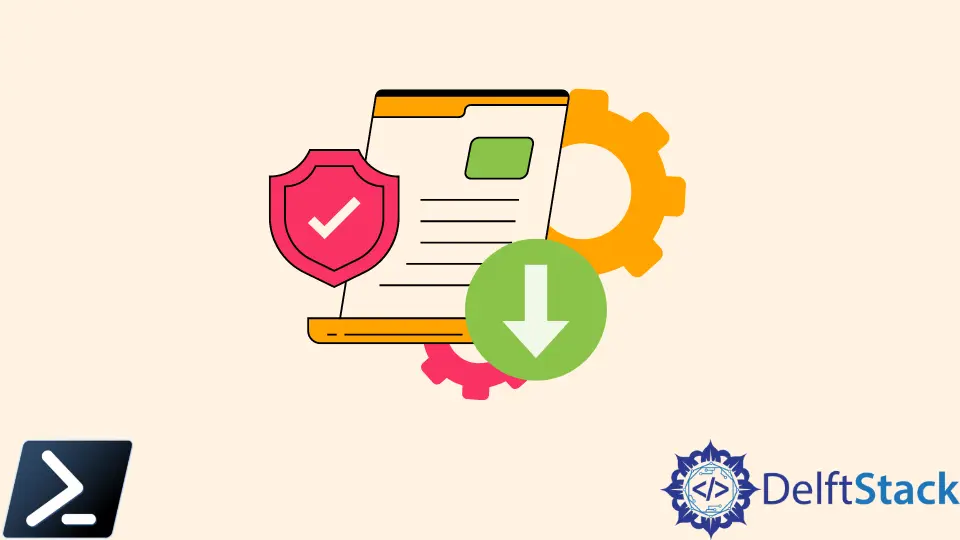
In today’s digital landscape, managing certificates is a crucial task for maintaining security and trust in various applications. Whether you’re a system administrator or a developer, knowing how to import certificates using PowerShell can save you time and effort.
This tutorial will guide you through the process of importing certificates via PowerShell scripts, ensuring you have the necessary skills to manage your certificates effectively. We’ll cover the steps, provide clear examples, and explain everything in a straightforward manner. By the end of this article, you’ll be well-equipped to handle certificate imports seamlessly.
Understanding Certificates and PowerShell
Before diving into the practical aspects, it’s essential to understand what certificates are and why they matter. Certificates are digital documents used to prove the ownership of a public key, ensuring secure communications over networks. PowerShell, a powerful scripting language and command-line shell, allows you to automate various tasks, including certificate management.
Using PowerShell to import certificates simplifies the process, especially when dealing with multiple certificates or automating deployments. It can save you from the tedious manual process of importing certificates through a graphical interface.
Importing Certificates Using PowerShell
The process of importing certificates in PowerShell involves a few straightforward steps. Below, we will explore how to import a certificate from a file, which is one of the most common methods.
Method 1: Importing a Certificate from a File
To import a certificate from a file, you can use the Import-PfxCertificate
cmdlet. This cmdlet allows you to specify the path of the certificate file and the store location where you want to import it.
Here’s a simple example:
$certPath = "C:\path\to\your\certificate.pfx"
$certPassword = ConvertTo-SecureString "your_password" -AsPlainText -Force
Import-PfxCertificate -FilePath $certPath -CertStoreLocation Cert:\LocalMachine\My -Password $certPassword
Output:
Certificate imported successfully.
In this example, we first define the path to the certificate file and the password associated with it. The ConvertTo-SecureString
cmdlet is used to convert the plain text password into a secure string, which is a requirement for the Import-PfxCertificate
cmdlet. Finally, the certificate is imported into the specified certificate store location, which in this case is the local machine’s personal store.
This method is particularly useful for administrators who need to deploy certificates across multiple machines or environments. By scripting this process, you can ensure consistency and reduce the risk of human error.
Method 2: Importing a Certificate from a Base64 Encoded String
Another common scenario is importing a certificate that is stored as a Base64 encoded string. This method is especially useful when you need to handle certificates programmatically.
Here’s how you can do it:
$base64Cert = "MIIDdzCCAl+gAwIBAgIEU..."
$certBytes = [System.Convert]::FromBase64String($base64Cert)
$cert = New-Object System.Security.Cryptography.X509Certificates.X509Certificate2
$cert.Import($certBytes)
$store = New-Object System.Security.Cryptography.X509Certificates.X509Store("My", "LocalMachine")
$store.Open("ReadWrite")
$store.Add($cert)
$store.Close()
Output:
Certificate imported successfully from Base64 string.
In this example, we start by defining a Base64 encoded certificate string. The FromBase64String
method converts the string into a byte array, which is then imported into a new X509Certificate2
object. After that, we create an instance of X509Store
, specifying the store name and location. We open the store in read/write mode, add the certificate, and finally close the store. This method is particularly effective for automation tasks where certificates are dynamically generated or retrieved.
Method 3: Importing a Certificate from a Remote Location
Sometimes, you may need to import a certificate from a remote location, such as a web server. PowerShell makes this process easy with the Invoke-WebRequest
cmdlet, allowing you to download the certificate before importing it.
Here’s how you can do it:
$remoteCertUrl = "https://example.com/certificate.cer"
$localCertPath = "C:\temp\downloaded_certificate.cer"
Invoke-WebRequest -Uri $remoteCertUrl -OutFile $localCertPath
Import-Certificate -FilePath $localCertPath -CertStoreLocation Cert:\LocalMachine\My
Output:
Certificate downloaded and imported successfully.
In this example, we specify the URL of the remote certificate and the local path where we want to save it. The Invoke-WebRequest
cmdlet downloads the certificate file, which is then imported using the Import-Certificate
cmdlet. This method is particularly useful for scenarios where certificates are frequently updated or managed through a centralized server.
Conclusion
Importing certificates using PowerShell is a valuable skill that can significantly streamline your workflow. Whether you’re working with local files, Base64 encoded strings, or remote locations, PowerShell provides a robust set of tools to manage certificates efficiently. By mastering these techniques, you can enhance your system’s security and ensure that your applications communicate securely. Remember to follow best practices for managing certificates, including regular updates and secure storage.
FAQ
-
How do I check if a certificate is installed?
You can use theGet-ChildItem Cert:\LocalMachine\My
command to list all certificates in the local machine’s personal store. -
Can I automate certificate imports?
Yes, PowerShell scripts can be scheduled to run automatically, allowing for seamless certificate management. -
What file formats can I import?
PowerShell can import several formats, including .pfx, .cer, and .pem. -
Is it safe to store passwords in scripts?
It is generally not recommended to store plain text passwords in scripts. Use secure strings or other secure methods for handling sensitive information. -
What should I do if the import fails?
Check the file path, ensure the certificate is valid, and verify that you have the necessary permissions to access the certificate store.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn