How to Get the File System Location of a PowerShell Script
- Using the Automatic Variable $PSScriptRoot
- Using the $MyInvocation Variable
- Combining Methods for Enhanced Functionality
- Conclusion
- FAQ
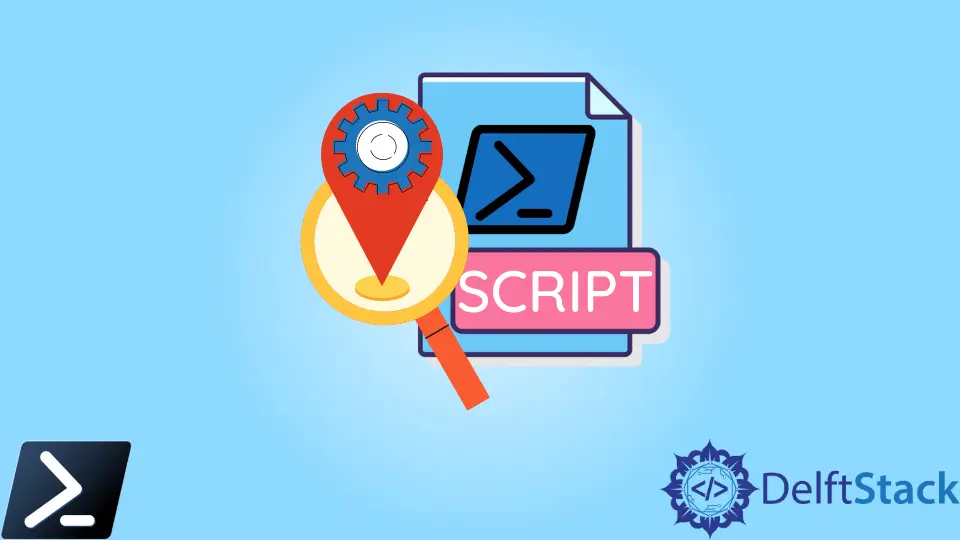
In the world of scripting, knowing the file system location of your PowerShell script can be incredibly useful. Whether you’re debugging, logging, or simply trying to understand the environment your script is running in, this information can help streamline your workflow.
In this tutorial, we’ll explore how to retrieve the file system location of a running PowerShell script. By the end of this guide, you’ll have a solid understanding of the methods available to achieve this, along with practical examples to help you along the way. So, let’s dive right in!
Using the Automatic Variable $PSScriptRoot
One of the simplest and most effective ways to get the file system location of a running PowerShell script is by using the automatic variable $PSScriptRoot
. This variable contains the directory from which the script is being executed, making it an invaluable tool for any PowerShell developer.
Here’s how you can use it in your script:
$scriptLocation = $PSScriptRoot
Write-Output "The script is located at: $scriptLocation"
Output:
The script is located at: C:\Path\To\Your\Script
In this example, $PSScriptRoot
automatically retrieves the directory of the script when it runs. This means you don’t have to hard-code paths or worry about where the script is located. It’s particularly useful when your script interacts with other files in the same directory, as you can easily reference them relative to the script’s location.
Moreover, $PSScriptRoot
is especially handy for scripts that are part of larger projects or repositories. It ensures that your scripts are portable and can be executed from different environments without modification. By using this variable, you can maintain a clean and efficient codebase, allowing for easier collaboration and version control.
Using the $MyInvocation Variable
Another powerful method to obtain the file system location of a PowerShell script is by leveraging the $MyInvocation
automatic variable. This variable provides information about the current command that is being executed, including the path of the script.
Here’s how to use it:
$scriptPath = $MyInvocation.MyCommand.Path
Write-Output "The script is located at: $scriptPath"
Output:
The script is located at: C:\Path\To\Your\Script\YourScript.ps1
The $MyInvocation.MyCommand.Path
retrieves the complete path of the script, including the script name itself. This can be particularly useful when you need not just the directory but the full path for logging or error handling purposes.
Using $MyInvocation
can also help in scenarios where you need to extract the script name or manipulate the path for further processing. For example, if you want to extract just the file name, you can use the Split-Path
cmdlet:
$scriptName = Split-Path -Path $scriptPath -Leaf
Write-Output "The script name is: $scriptName"
Output:
The script name is: YourScript.ps1
This flexibility allows for more dynamic scripting capabilities, making it easier to adapt your scripts to different contexts or environments.
Combining Methods for Enhanced Functionality
While the methods above are effective on their own, combining them can yield even more powerful outcomes. For instance, you might want to log both the directory and the script name for better traceability. This approach can be particularly useful for larger projects where tracking script execution is crucial.
Here’s a code snippet that combines both methods:
$scriptLocation = $PSScriptRoot
$scriptName = Split-Path -Path $MyInvocation.MyCommand.Path -Leaf
Write-Output "The script is located at: $scriptLocation"
Write-Output "The script name is: $scriptName"
Output:
The script is located at: C:\Path\To\Your\Script
The script name is: YourScript.ps1
In this example, you get both the directory and the script name, allowing for comprehensive logging or processing. This can be particularly helpful for scripts that need to reference other files in the same directory, as you can easily build paths based on the script’s location.
Additionally, combining these methods can help you create a more robust error handling mechanism. For instance, if your script encounters an error, you can log the location and the name of the script, making it easier to debug issues later on.
Conclusion
In summary, knowing how to get the file system location of a PowerShell script is essential for efficient scripting and development. By utilizing the automatic variables $PSScriptRoot
and $MyInvocation
, you can easily retrieve the script’s directory and name, allowing for better organization and error handling. Whether you’re working on a small project or a large-scale application, these techniques will enhance your scripting capabilities and streamline your workflow. So, next time you write a PowerShell script, remember these handy methods!
FAQ
-
How can I get just the directory of the script without the file name?
You can use the$PSScriptRoot
variable to get only the directory path of the running script. -
What if my script is called from another location?
The variables$PSScriptRoot
and$MyInvocation
will still provide the correct path based on where the script is located, not where it was called from. -
Can I use these methods in a function within my script?
Yes, both$PSScriptRoot
and$MyInvocation
work within functions, allowing you to get the script location regardless of where the function is called. -
Are these methods available in all versions of PowerShell?
Yes, both methods are available in PowerShell 3.0 and later versions. -
How can I use the script location in a logging mechanism?
You can concatenate the script location with your log file name to create a full path for your logs, ensuring they are saved in the same directory as your script.