How to Get String Length of a Variable in PowerShell
-
Use
GetType()
to Check Data Type of a Variable in PowerShell -
Use
$string.Length
to Get the String Length of a Variable in PowerShell -
Use
Measure-Object
to Get the String Length of a Variable in PowerShell
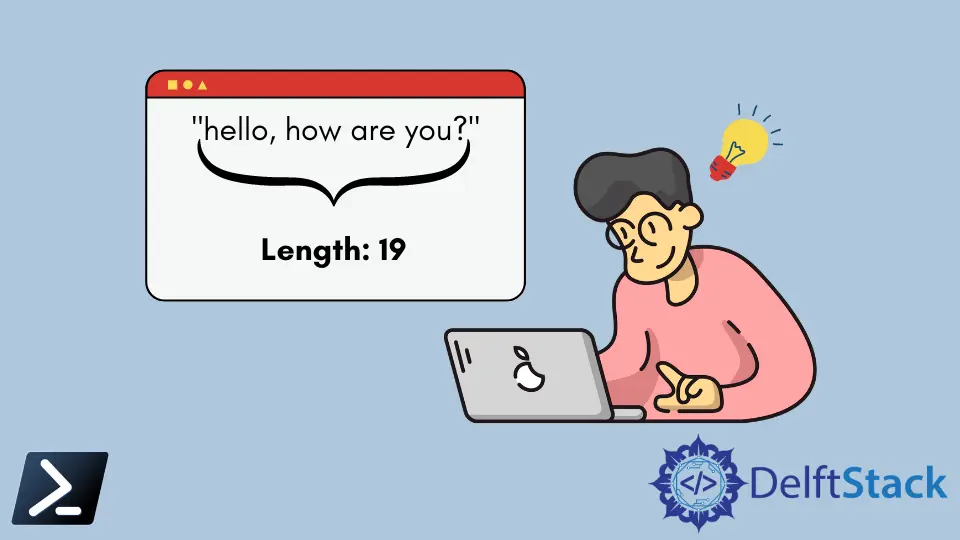
The string is one of the most common data types used in PowerShell; it contains the sequence of characters or texts. You can define a string by using single or double-quotes.
The PowerShell string has the System.String
object type. This tutorial will teach you to get the string length of variables in PowerShell.
Use GetType()
to Check Data Type of a Variable in PowerShell
We have created a string variable $text
as shown below.
$text = "hello, how are you?"
You can check the data type of a variable using GetType()
method.
$text.GetType()
Output:
IsPublic IsSerial Name BaseType
-------- -------- ---- --------
True True String System.Object
Use $string.Length
to Get the String Length of a Variable in PowerShell
The $string.Length
is the most straightforward method to check a string length of a variable in PowerShell.
$text.Length
Output:
19
You can test if a variable is more than eight characters using the following command.
if ($text.Length -gt 8) {
Write-Output "True"
}
It returns True
if the variable string length is more than eight characters and prints nothing if it is not.
Output:
True
Instead of the if
condition, you can also use the ternary operator ?:
. The ternary operator is available only from PowerShell 7.0.
($text.Length -gt 8) ? "True" : "False"
Output:
True
-gt
is the comparison operator in the PowerShell and indicates the greater than
. The comparison operators are used to compare the values and test conditions in the PowerShell.
Other useful comparison operators are as follows:
-eq: equals
-ne: not equals
-ge: greater than or equal
-lt: less than
-le: less than or equal
Use Measure-Object
to Get the String Length of a Variable in PowerShell
The Measure-Object
cmdlet calculates the numeric properties of certain types of objects in the PowerShell. It counts the number of string objects’ words, lines, and characters.
You can get the string length of a variable using the command below.
$text | Measure-Object -Character
The total number of characters is the total length of a string.
Output:
Lines Words Characters Property
----- ----- ---------- --------
19
You can check the number of lines and words using the parameter -Line
and -Word
, respectively.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell