How to Get Registry on a Remote Computer in PowerShell
-
The
Invoke-Expression
in PowerShell -
Use
Get-ItemProperty
to Get Registry in PowerShell -
Use Invoke-Expression and
Get-ItemProperty
to Get Registry on a Remote Computer in PowerShell
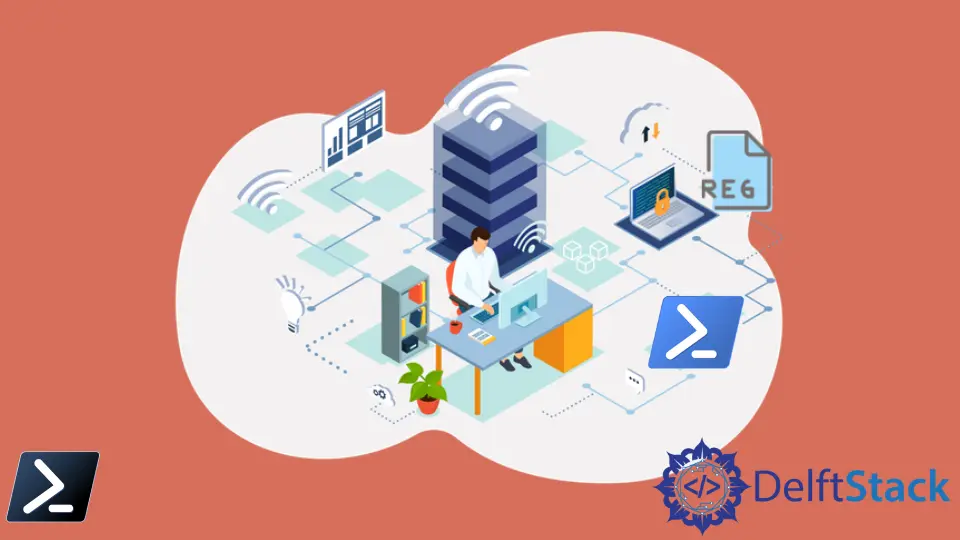
In this article, we will tackle how we can invoke expressions on a remote computer, get registry values, and how we can combine them both to get registry values on a remote computer.
The Invoke-Expression
in PowerShell
According to the official Microsoft description, the Invoke-Expression
command runs or evaluates a specific string as a command and exports the results of the command or expression.
In other words, it can help call code within a script or build commands to be executed later. However, we can also use it cautiously with user-provided input.
The most basic syntax of using Invoke-Expression
is defining a script and passing that string to the Command
parameter. Invoke-Expression
then executes that string.
$Command = 'Get-Process'
Invoke-Expression -Command $Command
#Execute a script via Invoke-Expression
$MyScript = '.\MyScript.ps1'
Invoke-Expression -Command $MyScript
However, Invoke-Expression
will execute the script as expected if we enclose the path item in single or double quotation marks. Consider this as a best practice as some paths have spaces in them.
$MyScript = "C:\'Folder Path'\MyScript.ps1"
Invoke-Expression $MyScript
The only parameter the Invoke-Expression
command has is the -Command
parameter. There is no other traditional way to pass parameters with Invoke-Expression
.
However, you can include them in the string you give to the Command
parameter.
Perhaps we have a script with two parameters called Path
and Force
. Instead of using a specific parameter via Invoke-Expression
, you can pass parameters to that script by giving them as you typically would via the console.
$scriptPath = 'C:\Scripts\MyScript.ps1'
$params = '-Path "C:\file.txt" -Force'
Invoke-Expression "$scriptPath $params"
# or
$string = 'C:\Scripts\MyScript.ps1 -Path "C:\file.txt" -Force'
Invoke-Expression $string
Use Get-ItemProperty
to Get Registry in PowerShell
The Get-ItemProperty
is a PowerShell command used to export registry entries and values in a more readable format. We can also get the value of a specific registry key using the Get-ItemProperty
cmdlet.
Example Code:
Get-ItemProperty -Path Registry::HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion
Output:
ProgramFilesDir
CommonFilesDir
ProgramFilesDir (x86)
CommonFilesDir (x86)
CommonW6432Dir
DevicePath
MediaPathUnexpanded
ProgramFilesPath
ProgramW6432Dir
SM_ConfigureProgramsName
SM_GamesName
Use Invoke-Expression and Get-ItemProperty
to Get Registry on a Remote Computer in PowerShell
Now, suppose we combine both concepts of invoking commands on a remote computer and getting the value of a registry key. In that case, we can now create a snippet of command that will get registry values in a remote computer.
Example Code:
Invoke-Command -Computer RemoteComputer01 {
Get-ItemProperty HKLM:\SOFTWARE\Microsoft\Windows\CurrentVersion\run
}
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn