How to Get AdGroupMember From Multiple Groups in PowerShell
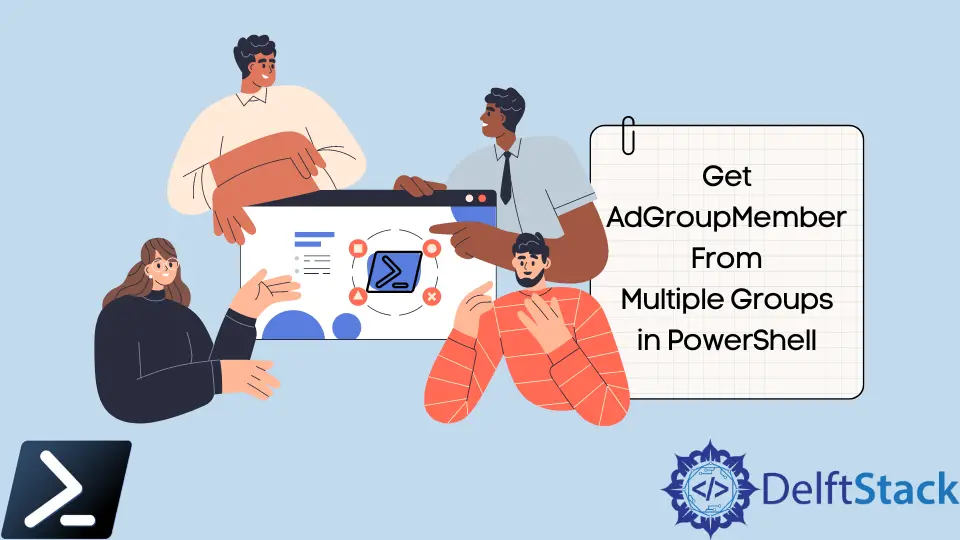
We can use the command Get-ADGroupMember
to get a list of members. These members are mainly from the ActiveDirectory
.
Now the ActiveDirectory
is a module containing a set of cmdlets mainly used to manage Active Directory members.
In this article, we are going to see how we can get the Group Members in PowerShell, and also we are going to discuss the topic by using examples and explanations to make the topic easier.
Get ADGroupMember
From Multiple Groups in PowerShell
Use the Get-ADGroupMember
cmdlet with the -Recursive
parameter to get adgroupmember
from multiple groups.
$groupNames = "Group1", "Group2", "Group3"
$results = @();
foreach ($group in $groupNames) {
$results += (Get-ADGroupMember -Identity $group -Recursive)
}
$results | Format-Table -AutoSize
After executing the above example code, you will get an output like the one below.
If you don’t have any output like the above, your environment may not contain the ActiveDirectory
. Use the steps below to fix this problem.
First of all, check whether you have ActiveDirectory
. To do this, follow the example below.
Get-Module -Name ActiveDirectory
If you have ActiveDirectory
, you will get an output like the one below.
ModuleType Version Name ExportedCommands
---------- ------- ---- ----------------
Manifest 1.0.1.0 ActiveDirectory {Add-ADCentralAccessPolicyMember, Add-ADComputerServiceAccount, Add-ADDomainControllerPasswordReplicationPolicy, Add-ADFineGrained...
If you don’t see any output like the above, just run the below command to install ActiveDirectory
.
Get-WindowsCapability -Name RSAT.ActiveDirectory* -Online | Add-WindowsCapability -Online
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn