How to Extract a Substring in PowerShell
-
Use the
Substring()
Method to Extract a Substring in PowerShell -
Dynamically Finding a Substring Using the
Length
Method in PowerShell
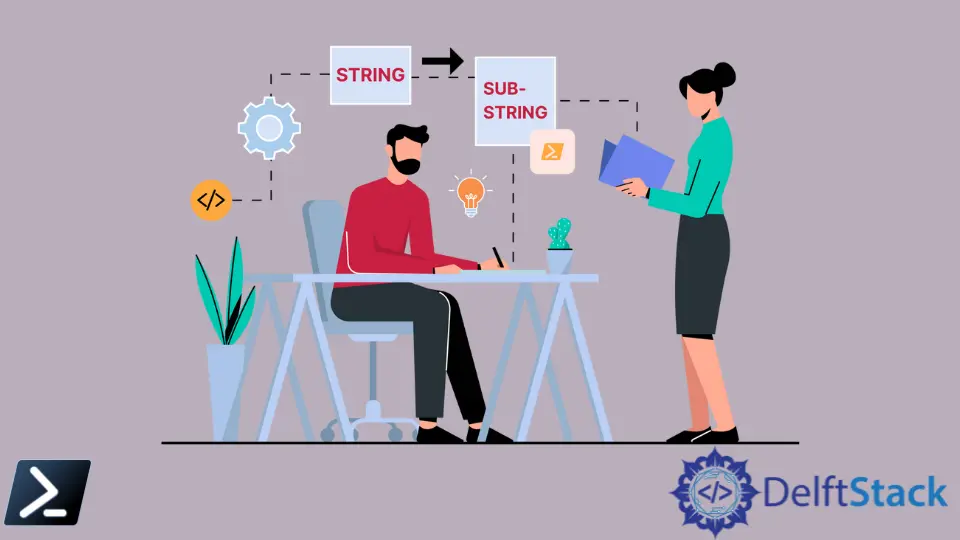
A typical scenario as a Windows administrator is to figure out a way to find a particular snippet of text inside a string called the substring. Windows PowerShell makes finding a substring easy.
The article will discuss efficiently extracting a substring in a string using PowerShell’s string library.
Use the Substring()
Method to Extract a Substring in PowerShell
We can use the Substring()
method to find a string inside a string. For example, perhaps we have a string like 'Hello World is in here Hello World!'
and you’d like to find the first four characters.
Example Code:
$sampleString = 'Hello World is in here Hello World!'
$sampleString.Substring(0, 5)
Output:
Hello
The first argument to pass in the Substring()
method is the position of the leftmost character, which is 'H'
. The second argument to pass is the farthest rightmost character position, which is the space character.
The Substring()
method returns all the characters preceding the character position in the second character.
Dynamically Finding a Substring Using the Length
Method in PowerShell
Using the string $sampleID = 'John_Marc_2021'
and you want to find the last four characters, instead of doing something like the example below.
Example Code:
$sampleID = 'John_Marc_2021'
$sampleID.SubString(0, 4)
Output:
John
We can replace 0
with $sampleID.Length - 4
argument, and we don’t need to provide the second argument. The Length
string method returns the position of the last character.
Using the Length
method of the string, which is the total number of characters in the given string, and deducting from that count, allows us to pick out substrings dynamically.
Example Code:
$sampleID = 'John_Marc_2021'
$sampleID.SubString($sampleID.Length - 4) # The - 4 in the expression will subtract the Length of the substring.
Output:
2021
The PowerShell substring method will always default to the last character position if we don’t specify the end position.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell