How to Edit a Text File on the Console Using PowerShell
- Use Notepad to Edit a Text File on the PowerShell Console
-
Use
nano
to Edit a Text File on the PowerShell Console -
Use
vim
to Edit a Text File on the PowerShell Console -
Use
emacs
to Edit a Text File on the PowerShell Console
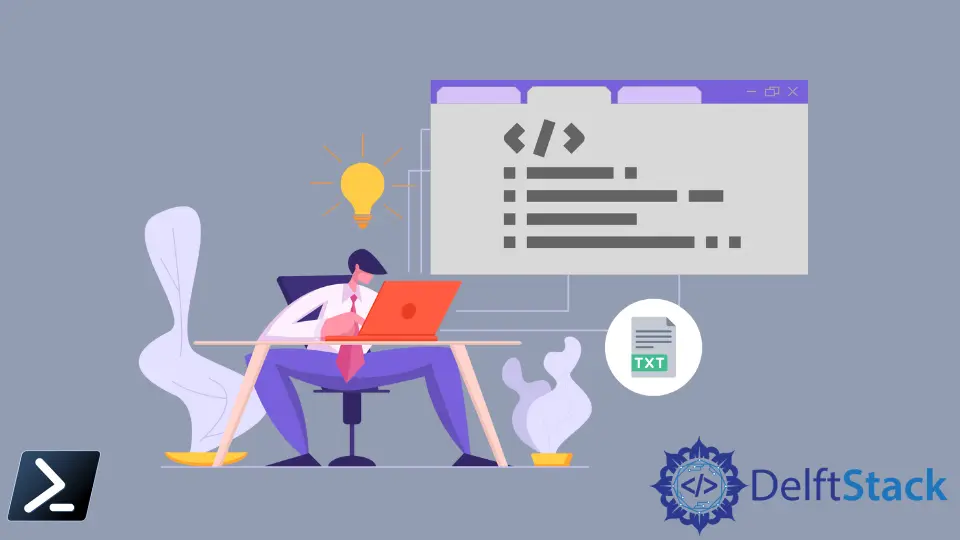
This tutorial will introduce multiple ways to edit a text file on the PowerShell console. You can add or remove contents in the text file without leaving the PowerShell console.
Use Notepad to Edit a Text File on the PowerShell Console
One of the easiest ways to edit a text file in PowerShell is a notepad. Notepad is a simple text editor for Windows to create and edit plain text documents.
notepad record.txt
When you use the notepad
command in PowerShell, it opens the text file in the notepad application. It is easier to edit in notepad as it is user-friendly.
Use nano
to Edit a Text File on the PowerShell Console
The nano
text editor is another way to edit a text file on the PowerShell console.
It is a small and friendly text editor for use in the terminal. You need to install nano
to use it on the PowerShell.
At first, you must install the chocolatey
package, which will help install nano
in the system. Ensure you open PowerShell as an administrator to install it.
Set-ExecutionPolicy Bypass -Scope Process -Force; iex ((New-Object System.Net.WebClient).DownloadString('https://community.chocolatey.org/install.ps1'))
After the chocolatey
package is installed, you can run this command to install the nano
editor.
choco install nano
You can use the nano
editor on the PowerShell console to edit a text file. It will open the GNU nano editor
to make changes to any text file.
nano record.txt
Use vim
to Edit a Text File on the PowerShell Console
Vim
is another text editor you can use on the PowerShell console. Firstly, open the PowerShell as an administrator and install vim
using the choco
.
choco install vim
After the installation, you can run vim
to edit a text file on the PowerShell console.
vim record.txt
Use emacs
to Edit a Text File on the PowerShell Console
You can also use emacs
text editor like other text editors.
choco install emacs
Then you can edit text files using emacs
on the PowerShell console.
emacs record.txt