Counter in PowerShell
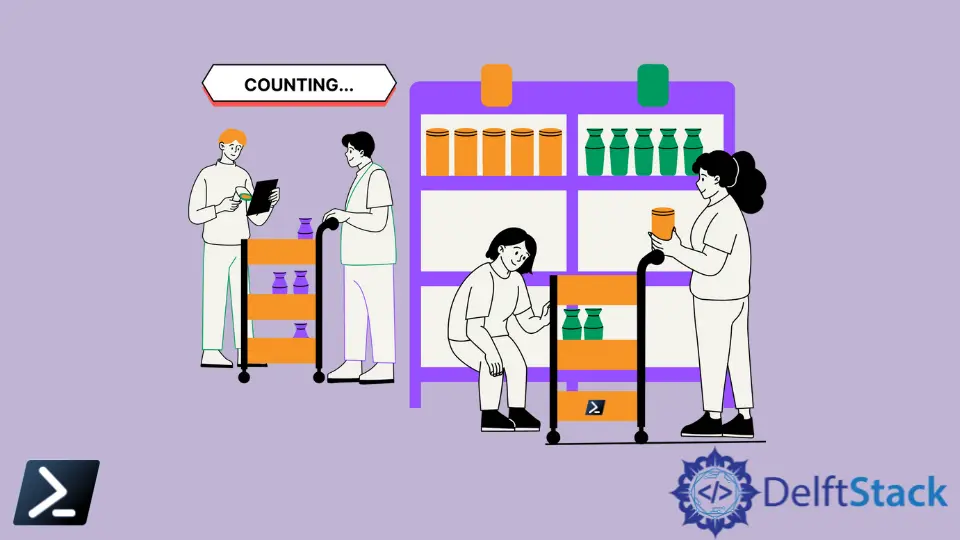
In programming, counters are used to track what is needed to be counted within your program. Usually, this is a numeric type variable that is initialized to 0
.
There are several approaches to increment a counter within a program. In this article, we will be focusing on different approaches to implementing counters in PowerShell.
Basic Counter in PowerShell
The basic way to implement a counter is by declaring a variable and initializing it to 0
, as shown in the following.
$counter = 0
Let’s print the $counter
variable value to the PowerShell command window.
Write-Host $counter
Output:
We can use two methods to increment the value of the $counter
variable. One traditional way is to add one to the $counter
and re-assign the result to the $counter
.
$counter = $counter + 1
Output:
Another approach is to use the increment (++
) operator.
$counter++
Output:
Next, we will use the post-increment to increment the value of the $countVar
.
$counterHolder = $countVar++
Write-Host $counterHolder
Output:
As you can see, the $counterHolder
variable holds 0
, which means the $countVar++
didn’t increment within that expression. But the actual value of the $countVar
should have been incremented. Let’s print the $countVar
variable value.
Pre-Increment Operator in PowerShell
The pre-increment operator is used to increment a variable’s value before using it in an expression. Let’s understand this with the following example.
First, we will declare a variable called $preIncrVar
and initialize it to 0
.
$preIncrVar = 0
Write-Host $preIncrVar
Let’s use the pre-increment operator as follows.
$preIncrVarHolder = ++$preIncrVar
Write-Host $preIncrVarHolder
Output:
As expected, the $preIncrVarHolder
variable’s value is 1
, which means the pre-increment operator increments the value of the $preIncrVar
variable within the expression that is being used. These approaches can be used in the for
,while
and do...while
loops in PowerShell.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.