How to Convert String to Integer in PowerShell
-
Use
[int]
to Convert String to Integer in PowerShell -
Use
[System.Int32]
to Convert String to Integer in PowerShell - Define the Data Type of a Variable to Convert String to Integer in PowerShell
- Conclusion
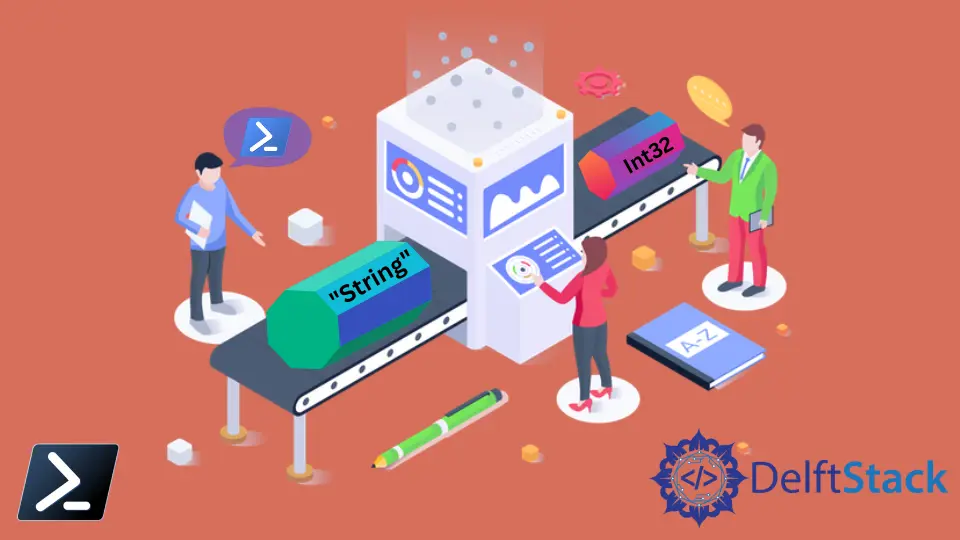
In PowerShell, knowing how to convert strings to integers is a valuable skill. This tutorial will teach you to convert strings to integers in PowerShell.
We will look into the technique of using [int]
and [System.Int32]
to perform this conversion, allowing you to easily modify your data.
Use [int]
to Convert String to Integer in PowerShell
In PowerShell, we can use [int]
to convert a string to an integer. This is called type casting or type conversion.
The following syntax is a simple assignment statement in PowerShell, where we are converting a string to an integer and assigning the result to the variable $integerVariable
.
Syntax:
$integerVariable = [int]$stringVariable
Parameters:
$integerVariable
: This is a variable that will hold the integer value after conversion.[int]
: This is used for type casting the value of$stringVariable
to an integer.$stringVariable
: This is another variable or expression containing a string that you want to convert to an integer.
Let us consider that we have a variable $a
as shown below.
$a = 123
The data type of $a
is an integer
.
$a.GetType().Name
Output:
Int32
But when we enclose the value with " "
, the data type will become string
.
$b = "123"
$b.GetType().Name
Output:
String
To convert the string ($b = "123"
) data type to an integer, we can use [int]
as shown below.
$b = $b -as [int]
$b.GetType().Name
In the code above, we start with the variable $b
. The current value and data type of $b
are unknown to us at this point, but we want to ensure it is treated as an integer.
Next, the $b -as [int]
, we use the -as
operator for safe type casting. It attempts to convert the value of $b
to the specified type [int]
.
If it can be converted, the result is assigned back to $b
. If not, $b
remains unchanged.
In the next line, we use $b.GetType().Name
to determine the data type of the variable $b
after the type casting operation.
Now, let’s go through what happens with a variable $b
having a specific value and type:
-
If
$b
initially contains an integer (e.g.,$b = 42
), the code will not change its type, and the output will beInt32
. -
If
$b
initially contains a string that can be successfully converted to an integer (e.g.,$b = "123"
), the code will change its type to an integer, and the output will beInt32
. -
If
$b
initially contains a string that cannot be converted to an integer (e.g.,$b = "hello"
), the code will not change its type, and the output will be the type of the original value, which, in this case, is a string so that the output would beString
.
Output:
Int32
Given the output Int32
, it implies that the initial value of the variable $b
(before this code snippet) was either an integer or a string that could be successfully converted to an integer.
Use [System.Int32]
to Convert String to Integer in PowerShell
The [System.Int32]
is a form of typecasting that converts a string to an integer in PowerShell, which explicitly tells PowerShell to treat a variable or expression as an Int32
data type.
In the syntax, we convert a string in $stringValue
to an integer, storing the result in $intValue
. This is done using the [System.Int32]
syntax to specify the desired data type, making $stringValue
ready for numeric operations.
Syntax:
$intValue = [System.Int32]$stringValue
Parameters:
$intValue
: This variable stores the integer value after the conversion.[System.Int32]
: This denotes the desired data type, specifying that the value should be treated as a 32-bit integer.$stringValue
: This contains the value to be converted, typically a string with a numeric value.
Let’s give an example.
$stringValue = "456"
$intValue = [System.Int32]$stringValue
$intValue.GetType().Name
In the code, we begin by setting a string variable named $stringValue
to the value "456"
. Then, we proceed to convert this string into an integer using [System.Int32]
and store the result in another variable, $intValue
.
After this conversion, we inquire about the data type of the variable $intValue
using $intValue.GetType().Name
.
Output:
Int32
The output tells us that the variable $intValue
contains an integer, specifically a 32-bit integer, as indicated by Int32
, which is the name of the data type.
Define the Data Type of a Variable to Convert String to Integer in PowerShell
PowerShell can detect the data type of a variable on its own.
We don’t need to be specific about the data type and declare a variable. But, we can define the data type of a variable before it so that we can force the data type.
Let’s give an example.
[int]$c = "456"
$c.GetType().Name
In the code above, we declare the variable and initialize it like this: [int]$c = "456"
. This line tells PowerShell to explicitly cast the string "456"
to an integer, and the result is then stored in the variable $c
.
Next, we want to know the data type of the variable $c
, so we use $c.GetType().Name
. This is our way of checking whether the typecasting was successful.
Output:
Int32
The output above is Int32
, indicating that the string "456"
has been converted into an integer. But, if you try to force an integer data type to a string value, you will get an error like in the following code example.
[int]$data = "storage"
$c.GetType().Name
In the code above, we start by declaring a variable named $data
and assigning the string value "storage"
to it. The next line, [int]$data
, represents an attempt to forcefully cast the string "storage"
to an integer data type.
Now, we’re going to check the data type of the variable $data
using $data.GetType().Name
.
Output:
Cannot convert value "storage" to type "System.Int32". Error: "Input string was not in a correct format."
At line:1 char:1
+ [int]$data = "storage"
+ ~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : MetadataError: (:) [], ArgumentTransformationMetadataException
+ FullyQualifiedErrorId : RuntimeException
We try to cast it as an integer using [int]
. However, since the string "storage"
cannot be converted to an integer, PowerShell does not change the type of the variable and returns an error message "Input string was not in a correct format."
.
Conclusion
In this article, we’ve learned to convert strings into integers using [int]
and [System.Int32]
. Now, we can easily transition between text to numbers in PowerShell.
By controlling variable types, you gain accuracy and versatility in your scripts.