How to Check if a File Exists in Windows PowerShell
-
Use
Test-Path
to Check if a File Exists in PowerShell -
Use
[System.IO.File]::Exists()
to Check if a File Exists in PowerShell -
Use
Get-Item
to Check if a File Exists in PowerShell -
Use
Get-ChildItem
to Check if a File Exists in PowerShell
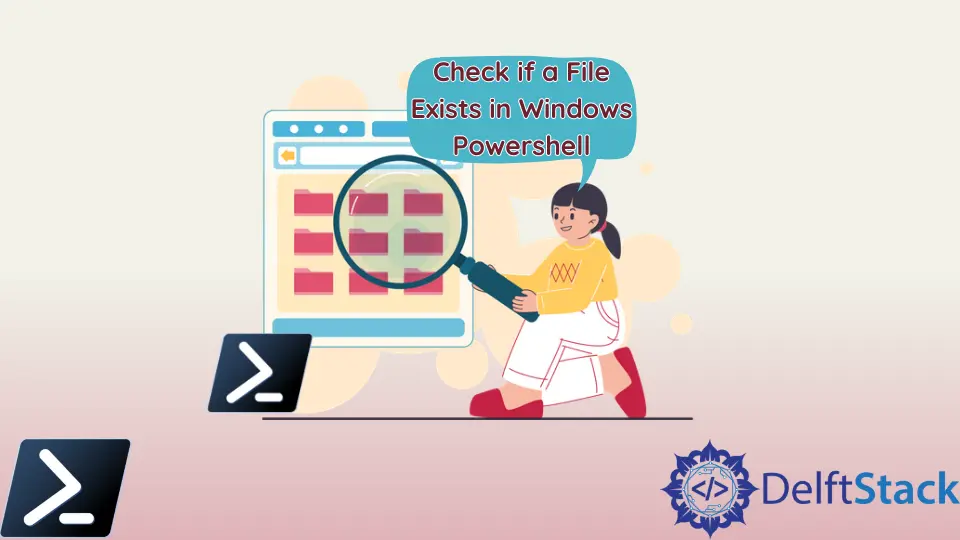
Sometimes, you get an error message saying the file does not exist in PowerShell.
This tutorial will introduce four methods to check if a file exists in PowerShell.
Use Test-Path
to Check if a File Exists in PowerShell
The first method is the Test-Path
cmdlet. It determines whether the complete path exists. It returns $True
if the path exists and $False
if any element is missing. The -PathType Leaf
parameter checks for a file and not a directory.
Test-Path -Path "C:/New/test.txt" -PathType Leaf
Output:
True
If there is no file named file.txt
in the directory New
, it returns $False
.
Test-Path -Path "C:/New/file.txt" -PathType Leaf
Output:
False
Use [System.IO.File]::Exists()
to Check if a File Exists in PowerShell
Another method to check if a file exists is [System.IO.File]::Exists()
. It provides a Boolean result, True
if the file exists or False
if the file does not exist.
[System.IO.File]::Exists("C:/New/test.txt")
Output:
True
Use Get-Item
to Check if a File Exists in PowerShell
The Get-Item
cmdlet is used to get the item at the specified path. You can use it to check if a file exists by specifying the file’s path. It prints the mode (attributes), last write time, length, and name of a file if it exists. It displays an error message if a file does not exist.
Get-Item C:/New/test.txt
Output:
Directory: C:\New
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 12/11/2021 2:59 PM 5 test.txt
Below is the output when the file doesn’t exist.
Get-Item : Cannot find path 'C:\New\test10.txt' because it does not exist.
At line:1 char:1
+ Get-Item C:/test/test10.txt
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : ObjectNotFound: (C:\New\test10.txt:String) [Get-Item], ItemNotFoundException
+ FullyQualifiedErrorId : PathNotFound,Microsoft.PowerShell.Commands.GetItemCommand
Use Get-ChildItem
to Check if a File Exists in PowerShell
The last method is to use Get-ChildItem
cmdlet. It gets the items and child items in one or more specified paths. It displays the file details if the file exists in the specified path.
Get-ChildItem -Path C:/New/test.txt
Output:
Directory: C:\New
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 12/11/2021 2:59 PM 5 test.txt
It prints an error message saying Cannot find path '$path' because it does not exist.
when a file is not found.
Get-ChildItem -Path C:/New/test
Output:
Get-ChildItem : Cannot find path 'C:\New\test' because it does not exist.
At line:1 char:1
+ Get-ChildItem -Path C:/New/test
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : ObjectNotFound: (C:\New\test:String) [Get-ChildItem], ItemNotFoundException
+ FullyQualifiedErrorId : PathNotFound,Microsoft.PowerShell.Commands.GetChildItemCommand