How to Accessing the $args Array in PowerShell
- What is the $args Array?
- Accessing $args in a PowerShell Script
- Using $args with Functions
- Practical Applications of $args
- Conclusion
- FAQ
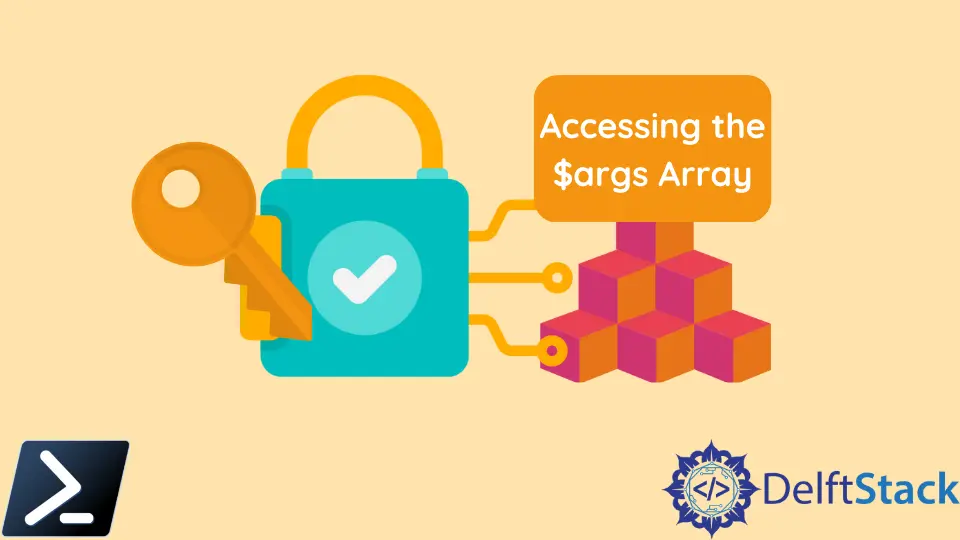
Understanding how to access the $args array in PowerShell is essential for anyone looking to streamline their scripting process. The $args array is a built-in feature that allows you to pass parameters to your PowerShell scripts or functions. This array collects all the arguments provided to a script or function, making it incredibly useful for handling input dynamically.
In this article, we will explore how to effectively use the $args array, providing you with practical examples and clear explanations. Whether you are a novice or an experienced scripter, mastering the $args array will enhance your PowerShell skills and improve your command line efficiency.
What is the $args Array?
The $args array in PowerShell is a special variable that holds all the arguments passed to a script or function. When you call a script or function with parameters, PowerShell automatically populates the $args array with these values. This makes it easy to access and manipulate input data without needing to define specific parameters in advance.
For example, if you run a script named example.ps1
with the command:
.\example.ps1 arg1 arg2 arg3
Inside the script, $args
will contain arg1
, arg2
, and arg3
. This capability allows for flexible scripting, as you can handle any number of arguments without explicitly defining them.
Accessing $args in a PowerShell Script
To access the $args array in a PowerShell script, you simply reference it by its name. Here’s a basic example of how to use it:
# example.ps1
foreach ($arg in $args) {
Write-Output "Argument: $arg"
}
When you execute this script with:
.\example.ps1 first second third
The output will be:
Argument: first
Argument: second
Argument: third
In this example, the foreach
loop iterates through each item in the $args array, allowing you to process each argument individually. This is particularly useful when you need to handle multiple inputs dynamically.
Using $args with Functions
You can also use the $args array within functions, which can enhance the modularity of your scripts. Here’s an example of how to define a function that utilizes $args:
function Show-Arguments {
foreach ($arg in $args) {
Write-Output "Received: $arg"
}
}
Show-Arguments "Hello" "World" "!"
When you run this code, the output will be:
Received: Hello
Received: World
Received: !
In this case, the Show-Arguments
function takes any number of arguments and processes them using the $args array. This approach allows you to create reusable functions that can accept varying input without hardcoding parameters.
Practical Applications of $args
The $args array can be incredibly useful in real-world scenarios, such as when creating scripts that automate tasks. For instance, if you are writing a script to back up files, you might want to allow users to specify the source and destination directories as arguments. Here’s how you could implement this:
# backup.ps1
$source = $args[0]
$destination = $args[1]
Copy-Item -Path $source -Destination $destination -Recurse
When executed like this:
.\backup.ps1 C:\Source D:\Backup
The script will copy all items from C:\Source
to D:\Backup
. This example illustrates how the $args array can make your scripts more flexible and user-friendly, enabling users to specify their own input without modifying the script’s code.
Conclusion
Accessing the $args array in PowerShell is a powerful technique that can significantly enhance your scripting capabilities. By understanding how to utilize this array, you can create dynamic scripts that accept a variety of inputs, making your work more efficient and effective. Whether you are automating tasks or developing complex scripts, mastering the $args array is a valuable skill that will serve you well in your PowerShell journey.
FAQ
-
What is the $args array in PowerShell?
The $args array is a built-in variable that stores all arguments passed to a script or function in PowerShell. -
How do I access the $args array?
You can access the $args array directly by referencing it in your script or function. -
Can I use $args in functions?
Yes, you can use the $args array within functions to handle dynamic input. -
How can I pass multiple arguments to a PowerShell script?
You can pass multiple arguments by simply listing them after the script name when executing it in the command line. -
What are some practical uses for the $args array?
The $args array can be used for tasks like file backups, data processing, and any scenario where dynamic input is required.