How to Print Variable in PostgreSQL
- Understanding RAISE NOTICE
- Printing a Simple Variable
- Printing Multiple Variables
- Printing Variables with Conditions
- Conclusion
- FAQ
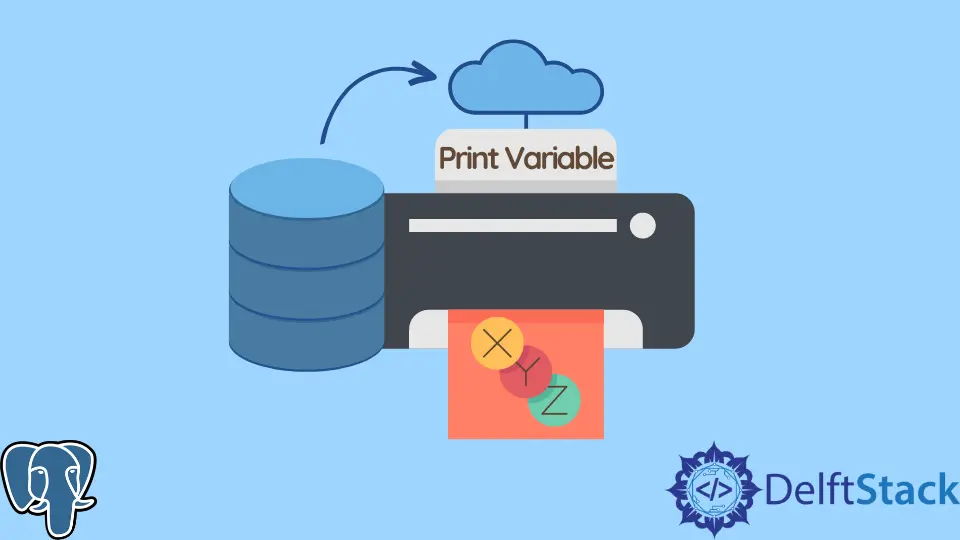
In the world of database management, PostgreSQL stands out as a powerful and versatile option. One of the essential functionalities that developers often seek is the ability to print variables for debugging or informational purposes. In PostgreSQL, this capability is achieved using the RAISE NOTICE
command. This command allows you to output messages, including variable values, directly to the console or logs. Understanding how to effectively print variables can significantly enhance your debugging process and improve the overall efficiency of your PL/pgSQL code.
In this article, we will explore various methods to print variables in PostgreSQL, ensuring you have the tools needed for effective database management.
Understanding RAISE NOTICE
When you want to print a variable in PostgreSQL, the RAISE NOTICE
command is your go-to solution. Unlike traditional print statements found in many programming languages, RAISE NOTICE
is specifically designed for PostgreSQL’s PL/pgSQL environment. It allows you to send messages to the client or log files, making it an invaluable tool for debugging and providing insights during code execution.
The syntax for using RAISE NOTICE
is straightforward:
RAISE NOTICE 'Your message here: %', your_variable;
In this syntax, you can include any message you want, and the %
acts as a placeholder for the variable you wish to print. This flexibility makes RAISE NOTICE
a powerful tool for monitoring the flow of your PL/pgSQL functions.
Printing a Simple Variable
Let’s start with a basic example of how to print a simple variable in PostgreSQL using RAISE NOTICE
. Suppose you want to print a variable that holds a user’s name.
DO $$
DECLARE
user_name TEXT := 'John Doe';
BEGIN
RAISE NOTICE 'User name is: %', user_name;
END $$;
Output:
User name is: John Doe
In this example, we define a variable user_name
and assign it the value ‘John Doe’. The RAISE NOTICE
command then outputs this value to the console. This method is particularly useful for quickly checking variable values during the execution of a PL/pgSQL block.
The DO
command allows you to execute an anonymous code block in PostgreSQL. By using DECLARE
, we can define variables that are only available within this block. The RAISE NOTICE
command effectively displays the content of the variable, making it easy to verify its value.
Printing Multiple Variables
Now, let’s explore how to print multiple variables in a single RAISE NOTICE
statement. This is particularly useful when you want to output several pieces of information at once.
DO $$
DECLARE
user_name TEXT := 'John Doe';
user_age INT := 30;
BEGIN
RAISE NOTICE 'User name is: %, User age is: %', user_name, user_age;
END $$;
Output:
User name is: John Doe, User age is: 30
In this example, we declare two variables: user_name
and user_age
. The RAISE NOTICE
command is then used to print both variables in one statement. By separating the placeholders with commas, we can easily format the output to include multiple variables.
This approach not only makes your code cleaner but also provides a comprehensive view of the variable states at any given moment. It’s an efficient way to debug and monitor the values of multiple variables simultaneously.
Printing Variables with Conditions
Sometimes, you may want to print variables based on certain conditions. This can be done using IF
statements within your PL/pgSQL code. Let’s look at an example where we print a variable only if a specific condition is met.
DO $$
DECLARE
user_score INT := 85;
BEGIN
IF user_score >= 75 THEN
RAISE NOTICE 'User has passed with a score of: %', user_score;
ELSE
RAISE NOTICE 'User has failed with a score of: %', user_score;
END IF;
END $$;
Output:
User has passed with a score of: 85
In this scenario, we declare a variable user_score
and use an IF
statement to check its value. If the score is 75 or higher, we print a message indicating that the user has passed. Otherwise, we print a failure message. This method allows for more dynamic output, depending on the conditions of your variables.
Using conditional statements with RAISE NOTICE
enhances your ability to control what information gets printed based on the state of your data. It adds a layer of logic to your debugging process, making it easier to track variable values under different circumstances.
Conclusion
Printing variables in PostgreSQL using the RAISE NOTICE
command is a powerful technique for debugging and monitoring your PL/pgSQL code. By understanding how to effectively utilize this command, you can gain valuable insights into your database operations. Whether you’re printing simple variables, multiple variables, or conditionally displaying information, mastering this skill will undoubtedly enhance your development process. With these techniques in your toolkit, you’ll be better equipped to manage your PostgreSQL databases efficiently.
FAQ
-
what is RAISE NOTICE in PostgreSQL?
RAISE NOTICE is a command in PostgreSQL used to print messages to the console or log files, primarily for debugging purposes. -
how do I print multiple variables at once in PostgreSQL?
You can print multiple variables using RAISE NOTICE by including multiple placeholders separated by commas in the message string. -
can I use conditions with RAISE NOTICE?
Yes, you can use conditional statements like IF to control when to print messages using RAISE NOTICE based on variable values. -
is RAISE NOTICE the only way to print in PostgreSQL?
While RAISE NOTICE is the most common method for printing messages, PostgreSQL also supports other logging levels such as RAISE WARNING and RAISE EXCEPTION for different purposes. -
how can I improve my debugging process in PostgreSQL?
Utilizing RAISE NOTICE effectively, along with structured logging and monitoring tools, can significantly enhance your debugging process in PostgreSQL.