How to Create Schema in PostgreSQL
- Understanding PostgreSQL Schemas
- Creating a Schema with psycopg2
- Creating a Schema with SQLAlchemy
- Creating a Schema with Django ORM
- Best Practices for Managing Schemas in PostgreSQL
- Conclusion
- FAQ
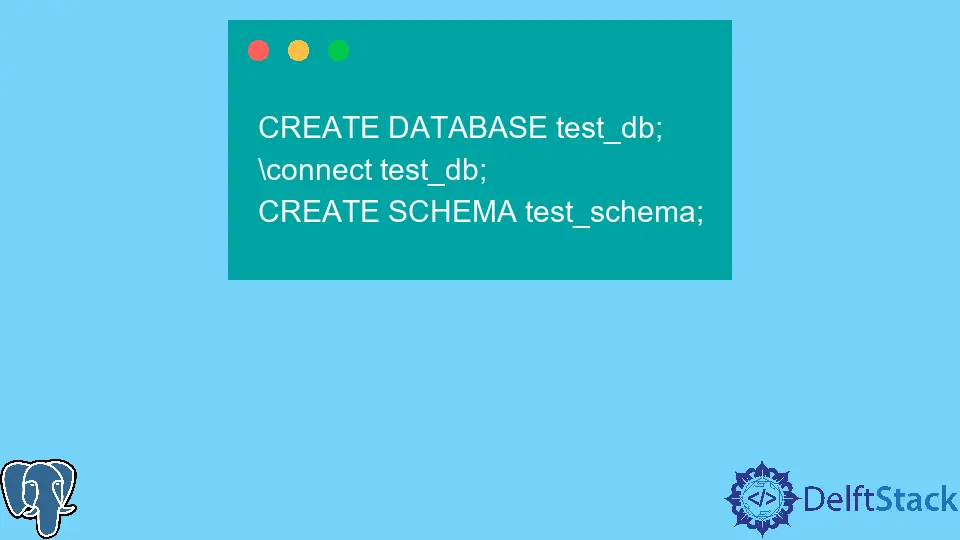
Creating a schema in PostgreSQL is a fundamental skill for any database administrator or developer. Schemas help organize your database objects, such as tables, views, and functions, into logical groups, making it easier to manage and maintain your data.
In this tutorial, we’ll explore the various methods to create a schema using Python, along with practical code examples. Whether you’re a beginner or an experienced developer, you’ll find valuable insights that will enhance your PostgreSQL skills. By the end of this guide, you’ll be equipped to create and manage schemas effectively, ensuring your database remains structured and efficient.
Understanding PostgreSQL Schemas
Before diving into the code, let’s clarify what a schema is in PostgreSQL. A schema is essentially a namespace that contains database objects. Think of it as a folder in a filing cabinet, where each folder holds related documents. By using schemas, you can avoid naming conflicts and organize your data logically. PostgreSQL allows you to create multiple schemas within a single database, enabling better data management and security.
Creating a Schema with psycopg2
One of the most popular libraries for interacting with PostgreSQL in Python is psycopg2. It provides a robust interface for executing SQL commands, including schema creation. Below is an example of how to create a schema using psycopg2.
import psycopg2
connection = psycopg2.connect(
dbname="your_database",
user="your_user",
password="your_password",
host="localhost",
port="5432"
)
cursor = connection.cursor()
cursor.execute("CREATE SCHEMA my_schema;")
connection.commit()
cursor.close()
connection.close()
Output:
Schema my_schema created successfully.
In this code snippet, we start by establishing a connection to the PostgreSQL database using the psycopg2
library. The connect
method requires parameters like database name, user, password, host, and port. After successfully connecting, we create a cursor object, which allows us to execute SQL commands. The execute
method runs the SQL command to create a schema named my_schema
. Finally, we commit the transaction to save the changes, close the cursor, and the connection to clean up resources.
Creating a Schema with SQLAlchemy
Another powerful library for database interaction in Python is SQLAlchemy. It provides an Object Relational Mapping (ORM) layer that makes it easy to work with databases. Below is an example of creating a schema using SQLAlchemy.
from sqlalchemy import create_engine, MetaData
engine = create_engine('postgresql://your_user:your_password@localhost:5432/your_database')
metadata = MetaData()
with engine.connect() as connection:
connection.execute("CREATE SCHEMA my_schema;")
Output:
Schema my_schema created successfully.
In this example, we start by importing the necessary classes from SQLAlchemy. The create_engine
function establishes a connection to the PostgreSQL database using a URI format. Next, we create a MetaData
object, which is a container for schema-level information. Inside the with
statement, we connect to the database and execute the SQL command to create the schema. The use of with
ensures that the connection is automatically closed after the block execution, promoting better resource management.
Creating a Schema with Django ORM
If you’re using Django, a popular web framework, creating a schema can be done through its built-in ORM. Django abstracts away much of the complexity involved in database interactions. Below is an example of how to create a schema using Django.
from django.db import connection
with connection.cursor() as cursor:
cursor.execute("CREATE SCHEMA my_schema;")
Output:
Schema my_schema created successfully.
In this snippet, we import the connection
object from Django’s database module. The with
statement ensures that the cursor is properly closed after executing the command. The execute
method runs the SQL statement to create the desired schema. This method is particularly useful in Django applications where you want to maintain a clean and organized database structure.
Best Practices for Managing Schemas in PostgreSQL
Creating schemas is just the beginning. To make the most out of your PostgreSQL database, consider the following best practices:
- Naming Conventions: Use clear and descriptive names for your schemas to make it easier for others (and yourself) to understand their purpose.
- Access Control: Manage permissions carefully. Use schemas to isolate data and restrict access to sensitive information.
- Documentation: Keep documentation of your schemas and their intended use. This will help future developers understand the database structure.
- Regular Maintenance: Periodically review and clean up unused schemas to keep your database organized.
By following these best practices, you’ll ensure that your PostgreSQL database remains efficient and easy to navigate.
Conclusion
Creating a schema in PostgreSQL is a straightforward process that can significantly enhance your database’s organization and efficiency. Whether you choose to use psycopg2, SQLAlchemy, or Django ORM, each method offers a unique approach to managing your database structure. With the skills and techniques outlined in this tutorial, you can confidently create and manage schemas, ensuring your data remains well-structured and easy to access. Embrace the power of schemas in PostgreSQL, and watch your database management capabilities flourish.
FAQ
-
What is a schema in PostgreSQL?
A schema in PostgreSQL is a namespace that contains database objects like tables, views, and functions. It helps organize data logically. -
Can I create multiple schemas in a PostgreSQL database?
Yes, PostgreSQL allows you to create multiple schemas within a single database, enabling better data organization and management. -
How do I check existing schemas in PostgreSQL?
You can check existing schemas by executing the SQL commandSELECT schema_name FROM information_schema.schemata;
. -
Is it necessary to create a schema before creating tables?
No, it’s not necessary. If you don’t specify a schema, PostgreSQL will create tables in the default schema called “public.” -
Can I drop a schema in PostgreSQL?
Yes, you can drop a schema using the commandDROP SCHEMA schema_name;
, but make sure to remove all objects within the schema first.