How to Add a Unique Constraint After Creating a Table in PostgreSQL
- Understanding Unique Constraints
- Adding a Unique Constraint Using SQL
- Checking for Duplicate Values
- Resolving Duplicates
- Finalizing the Unique Constraint
- Conclusion
- FAQ
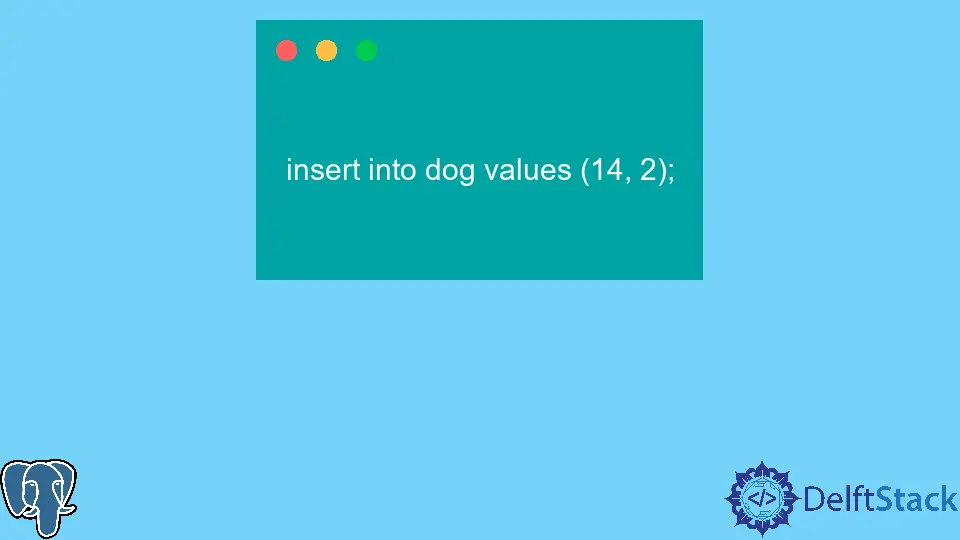
Adding a unique constraint to an existing table in PostgreSQL is a common requirement for database administrators and developers. Whether you’re looking to enforce data integrity, prevent duplicate entries, or simply improve your database design, understanding how to do this effectively is crucial.
In this article, we’ll explore the step-by-step process to add a unique constraint after a table has already been created. We’ll cover various methods, including SQL commands and best practices to ensure seamless implementation. By the end of this guide, you’ll be equipped with the knowledge to enhance your PostgreSQL tables efficiently.
Understanding Unique Constraints
Before diving into the methods, it’s essential to grasp what a unique constraint is. A unique constraint ensures that all values in a column are distinct from one another. This means no two rows can have the same value in the specified column. When you add a unique constraint to an existing table, PostgreSQL will check the current data and enforce this rule moving forward. If there are duplicates already present, the operation will fail unless you resolve those conflicts first.
Adding a Unique Constraint Using SQL
The most straightforward way to add a unique constraint to an existing table in PostgreSQL is through SQL commands. Here’s how you can do it:
ALTER TABLE your_table_name
ADD CONSTRAINT your_constraint_name UNIQUE (your_column_name);
In this command, replace your_table_name
with the name of your table, your_constraint_name
with a name you want to give the constraint, and your_column_name
with the name of the column you want to enforce uniqueness on.
Output:
ALTER TABLE your_table_name
ADD CONSTRAINT your_constraint_name UNIQUE (your_column_name);
This command modifies the existing table by adding a unique constraint. If the column already contains duplicate values, PostgreSQL will return an error. Therefore, it’s a good practice to check for duplicates before executing this command. You can do this by running a query that counts the occurrences of each value in the column.
Checking for Duplicate Values
Before adding the unique constraint, it’s crucial to ensure that there are no duplicate values in the column you want to constrain. You can easily check for duplicates using the following SQL query:
SELECT your_column_name, COUNT(*)
FROM your_table_name
GROUP BY your_column_name
HAVING COUNT(*) > 1;
Replace your_column_name
and your_table_name
accordingly. This query will return any values that occur more than once in the specified column.
Output:
your_column_name | count
------------------|-------
duplicate_value1 | 2
duplicate_value2 | 3
If this query returns any rows, you need to resolve these duplicates before you can successfully add the unique constraint. You can either delete or update the duplicate rows based on your data requirements.
Resolving Duplicates
Once you identify the duplicates, you’ll need to decide how to handle them. You have several options, such as deleting duplicates or updating them to ensure uniqueness. Here’s how you can delete duplicates while keeping one instance of each value:
DELETE FROM your_table_name
WHERE ctid NOT IN (
SELECT MIN(ctid)
FROM your_table_name
GROUP BY your_column_name
);
This command deletes all duplicate rows while retaining the first occurrence of each unique value. The ctid
is a system column in PostgreSQL that uniquely identifies rows in a table.
Output:
DELETE FROM your_table_name
WHERE ctid NOT IN (
SELECT MIN(ctid)
FROM your_table_name
GROUP BY your_column_name
);
After executing this command, you can re-run the duplicate-check query to ensure all duplicates are resolved. Once confirmed, you can proceed to add your unique constraint.
Finalizing the Unique Constraint
Now that you’ve resolved any duplicates, you can safely add the unique constraint to your table. Use the SQL command provided earlier to complete this step.
ALTER TABLE your_table_name
ADD CONSTRAINT your_constraint_name UNIQUE (your_column_name);
Output:
ALTER TABLE your_table_name
ADD CONSTRAINT your_constraint_name UNIQUE (your_column_name);
This command will now execute successfully, and your specified column will enforce uniqueness moving forward. You can verify that the constraint has been added by querying the information_schema
:
SELECT *
FROM information_schema.table_constraints
WHERE table_name = 'your_table_name';
Output:
constraint_name | constraint_type
----------------|-----------------
your_constraint_name | UNIQUE
This query will show all constraints associated with your table, confirming that your unique constraint is in place.
Conclusion
Adding a unique constraint after creating a table in PostgreSQL is a manageable task if approached systematically. By ensuring there are no duplicate values beforehand and using SQL commands effectively, you can enhance your database integrity. Remember to regularly check your data for duplicates to maintain the quality of your database. With these steps, you can confidently manage your PostgreSQL tables and enforce data uniqueness.
FAQ
- What happens if I try to add a unique constraint with existing duplicates?
You will receive an error message indicating that the constraint cannot be added due to duplicate values in the specified column.
-
Can I add a unique constraint to multiple columns?
Yes, you can create a composite unique constraint by specifying multiple columns in the UNIQUE clause. -
How do I remove a unique constraint in PostgreSQL?
You can remove a unique constraint using the command:ALTER TABLE your_table_name DROP CONSTRAINT your_constraint_name;
. -
Is it possible to add a unique constraint to a column that already has NULL values?
Yes, unique constraints allow multiple NULL values, as they are considered distinct. -
Can I name the unique constraint anything I want?
Yes, you can choose a name for your unique constraint, but it should be unique within the database schema.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub