How to Write to an Error Log File in PHP
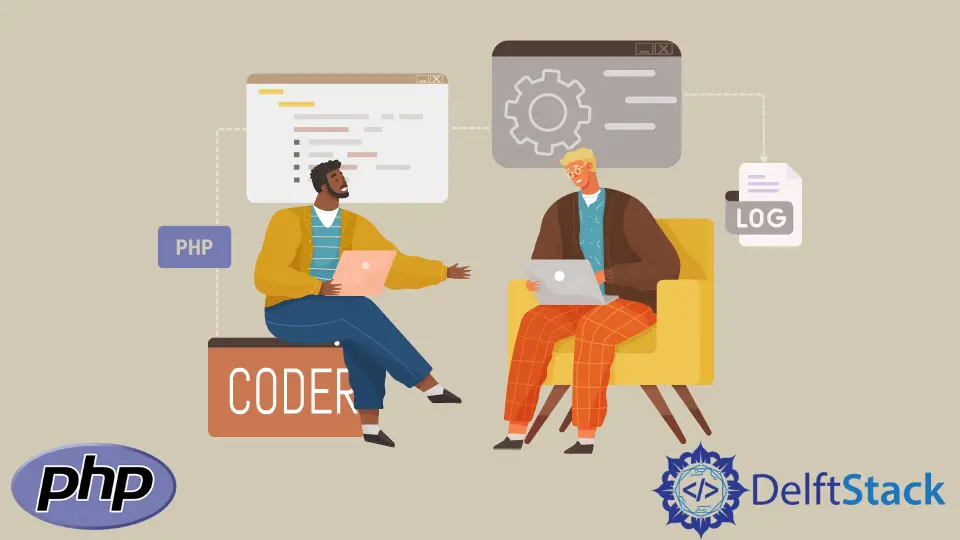
When we have a large-scale web application running on production mode, we need to monitor all the errors or potential errors that a user can face. The best way to keep a close eye on all the possible errors is by logging them into a file whenever there is an error.
This article will introduce the built-in function error_log()
and write error logs in a file in PHP.
Write to an Error Log File in PHP
PHP has a built-in function error_log()
that sends an error message to the defined error handling. We can easily log errors and warning into a PHP script file and change the configuration from PHP’s php.ini
file.
The error_log()
function takes in 3 arguments.
The first argument is the error we want to save into a file, and the second is the error message type. We usually use 3
as a message type to save the error messages to a file.
The third argument is the file’s location in which we want to save the error logs.
So, let’s go through an example. We’ll create an error and save it into a file using the error_log()
function.
# php
$error = "A new error is occured";
$path = "application-errors.log";
error_log($error, 3, $path);
Output:
From the above example, the error has been saved into a file. By using error_log()
in every function, we can log the errors in a file and ensure that the code works perfectly.
In addition, we can also send the time of the error to make sure when a specific error has occurred.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn