Optional Arguments in PHP
-
Use
"NULL"
as an Optional Argument - Use a Specific Value as an Optional Argument
- Use an Empty String as an Optional Argument
-
Use the Splat Operator (
...
) to Define Optional Argument -
Use
func_get_args
Method to Set an Optional Argument in PHP
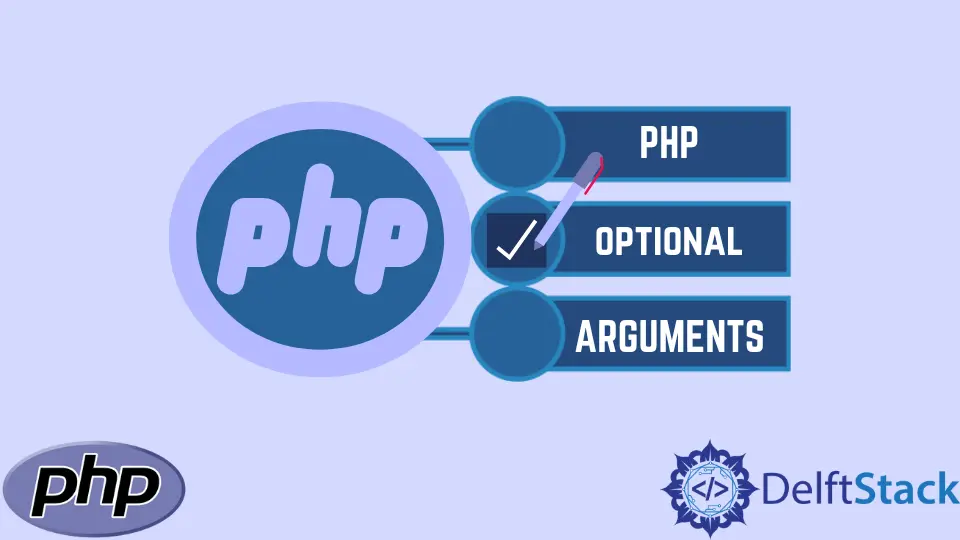
In PHP, optional arguments do not prevent the method from working even if no data is given. The article below shows examples of different ways of implementing optional arguments.
Use "NULL"
as an Optional Argument
We will create a function and pass a default argument
with its value set as "null"
. If we call the function without resetting the default argument value, then "null"
will be used in its place.
<?php
function fruits($bestfruit = "NULL")
{
return "I love enjoying $bestfruit" .'<br>';
}
echo fruits();
echo fruits('mango');
?>
Output:
I love enjoying NULL
I love enjoying mango
Use a Specific Value as an Optional Argument
We will create a function and pass a default argument with its value set as a string. If we call the function without resetting the default argument value, then the specified value will be used in its place.
<?php
function fruits($bestfruit = "Apple")
{
return "I love enjoying $bestfruit" .'<br>';
}
echo fruits();
echo fruits('mango');
?>
Output:
I love enjoying Apple
I love enjoying mango
Use an Empty String as an Optional Argument
Create a function and pass a default argument with its value set as an empty string.
<?php
function fruits($bestfruit = "")
{
return "I love enjoying $bestfruit" .'<br>';
}
echo fruits();
echo fruits('PineApples');
?>
Output:
I love enjoying
I love enjoying PineApples
Use the Splat Operator (...
) to Define Optional Argument
Here we don’t pass any default value. Instead, we will pass the splat operator (...
) that will default define an empty array when no argument has been passed to the function.
<?php
function fruits(...$bestfruit)
{
var_dump($bestfruit).'<br>';
}
echo fruits();
echo fruits('PineApples','test');
?>
Output:
array(0) { } array(2) { [0]=> string(10) "PineApples" [1]=> string(4) "test" }
Use func_get_args
Method to Set an Optional Argument in PHP
Same in using the splat operator (...
), we create a function without passing any default value. If we call the function without specifying a value, 0
will be the default value.
<?php
function summation() {
$numbers = func_get_args();
return array_sum($numbers);
}
echo summation().'<br>';
echo summation(1,2,3,4,5,6);
?>
Output:
0
21