True and False in PHP
-
Use
true
andfalse
as Boolean Logical Values in PHP -
Use the
var_dump()
Function to Determine Bool Values in PHP
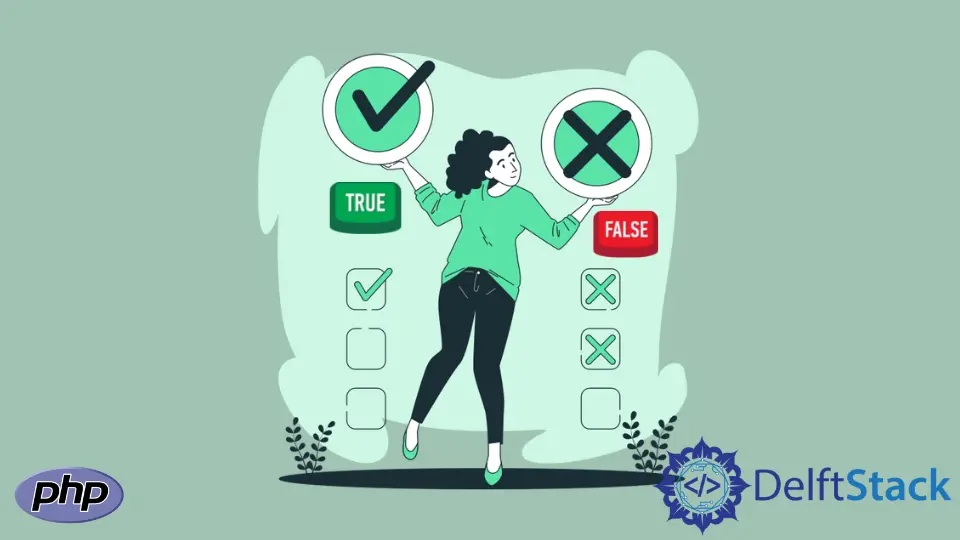
The boolean
values are called true
and false
in php. In the case of true
, the output is 1
.
While with the false
, it does not show any output. It is worth noting that the browser always renders these values in strings.
Use true
and false
as Boolean Logical Values in PHP
The booleans are logical values. They can either be true
or false
.
Both are case sensitive in php and are also simple data types in php.
$true = True; // returns 1
$false = False; // return nothing
echo $true.PHP_EOL;
echo $false;
//using comparision operator to check boolean values
if ($a == "abc") {
echo "return boolean value"; //returns nothing since $a is not equal to abc thus it is a false
}
if (TRUE) {
//because true means true
echo "Your condition is executed!".PHP_EOL;
}
if (false) {
echo "It will print nothing"; //false returns nothing
}
//Determine integer comparisions with boolean values
echo 5<10; //returns 1 since 5 is actually less ten (condition true)
echo 10 != 5; //returns 1 (true)
echo 5 == 4; //false
Output:
1
Your condition is executed!
11
Use the var_dump()
Function to Determine Bool Values in PHP
We can also convert bool values in php. Let us first understand some predefined value assignments to these parameters.
If we convert the following values to the boolean, they will be considered false
:
False
isfalse
.0
is alsofalse
.- Floats such as
0.0
and-0.0
are alsofalse
. - An array that has
0
elements isfalse
. NULL
is afalse
.
On the contrary, all other values are considered true
.
We can determine bool values with the help of the var_dump()
function that dumps information regarding a bool value.
Let’s check above mentioned false types with var_dump
:
<?php
var_dump((bool) false); //(false)
var_dump((bool) "0"); //(false)
var_dump((bool) 0.0); //(false)
var_dump((bool) -0.0); //(false)
var_dump((bool) NULL); //(false)
var_dump((bool) array()); //(false)
//true bools (a few examples)
var_dump((bool)true); //true
var_dump((bool)1); //true
var_dump((bool)100); //true
var_dump((bool) array(65)); //true
?>
Output:
bool(false)
bool(false)
bool(true)
bool(false)
bool(false)
bool(false)
bool(false)
bool(true)
bool(true)
bool(true)
bool(true)
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn