How to Autoload Classes in PHP
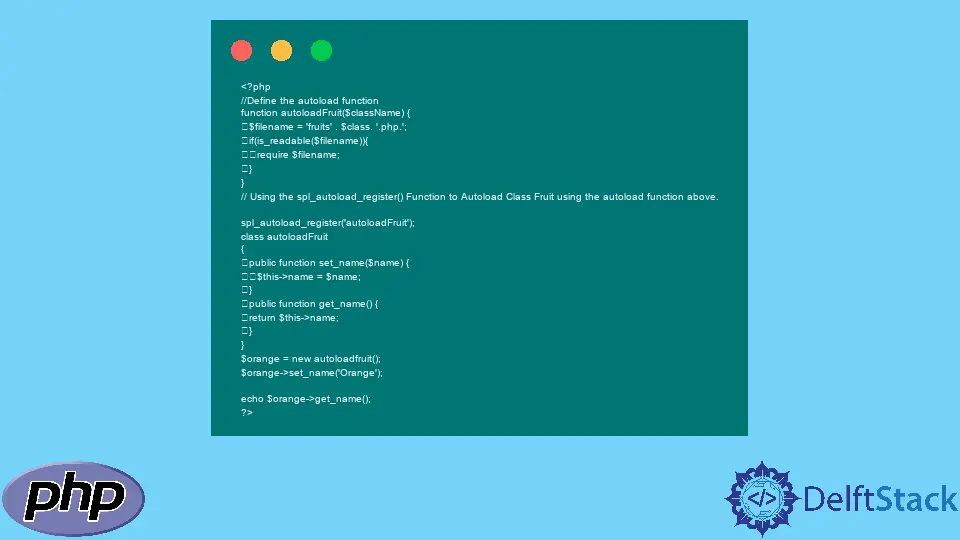
In this tutorial, we will introduce the spl_autoload_register()
function. We will see how you can load classes not defined in your PHP script.
Use the spl_autoload_register()
Function to Autoload Classes in PHP
First, we will create a PHP class. We will call this class fruit
and save the PHP script in a folder fruits
in our local server’s document root.
We will also create the object apple
in the class.
It is important to name your PHP script file as your class name. In our case, we will name our PHP script fruit.php
.
<?php
// Creating a Fruit class
Class fruit {
public $name;
public $color;
public function set_name($name) {
$this->name = $name;
}
public function get_name() {
return $this->name;
}
}
$apple = new fruit();
$apple->set_name('Apple');
echo $apple->get_name();
?>
Output:
Apple
Let’s create an object in such a class on a new script. We will use the spl_autoload_register()
function to instantiate the class on our new PHP script and create Orange
.
The code below illustrates how you can create a custom autoload function, in our case, the autoload fruits()
. We will refer to our custom autoload function when using the spl_autoload_register()
function.
<?php
//Define the autoload function
function autoloadFruit($className) {
$filename = 'fruits' . $class. '.php.';
if(is_readable($filename)){
require $filename;
}
}
// Using the spl_autoload_register() Function to Autoload Class Fruit using the autoload function above.
spl_autoload_register('autoloadFruit');
class autoloadFruit
{
public function set_name($name) {
$this->name = $name;
}
public function get_name() {
return $this->name;
}
}
$orange = new autoloadfruit();
$orange->set_name('Orange');
echo $orange->get_name();
?>
Output:
Orange
You can add multiple autoload functions when you have many scripts to refer to. You only need to create a custom autoload function for each class.
Alternatively you can use the include_once()
function.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn