How to Store Div Id in a PHP Variable and Pass It to JavaScript
-
What Is the
div id
in HTML -
Store the
div id
in a PHP Variable - Pass the PHP Variable to a JavaScript Code
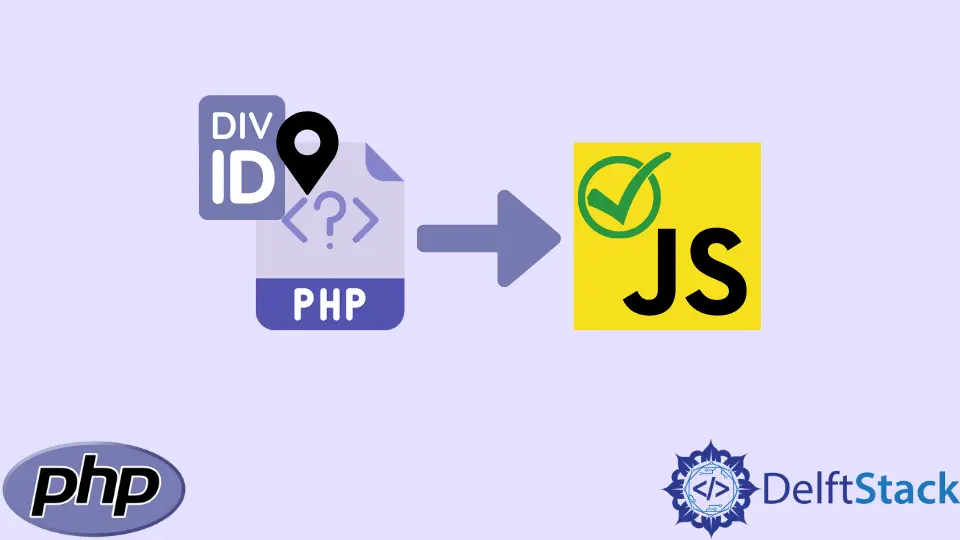
This tutorial will cover how to store div id
in a PHP variable and pass it to a JavaScript code.
We will answer the following questions.
- What is the
div id
? - How do you store the
div id
in a PHP variable? - How do you pass the variable to a JavaScript code?
Let’s jump right in.
What Is the div id
in HTML
The div id
is part of HTML. We use it to identify a unique HTML element and apply CSS or JavaScript to style the element.
Example:
Store the div id
in a PHP Variable
It is possible to store a div id
in a PHP variable, as shown below.
<?php
$divId = "Delft Stack Tutorials"
?>
<div id="<?php echo $divId;?>">
In the above code, our HTML element is Delft Stack Tutorials
, which is also our div id
. We use the variable $divId
to store our div id
.
Output:
<div id="Delft Stack Tutorials">
Pass the PHP Variable to a JavaScript Code
Below, we will pass the variable $divId
to a JavaScript code.
<!DOCTYPE html>
<html>
<head>
<title>
Storing div id in a PHP variable
</title>
</head>
<body style="text-align:center;">
<h1 style="color:blue;">DelftStack</h1>
<h2 style="color:black">
Storing div id in a PHP variable
</h2>
<head>
<style>
.custmDiv {
border: 5px outset red;
background-color: lightblue;
text-align: center;
}
</style>
</head>
<?php
$divId = "Delft Stack Tutorials"
?>
<div class="custmDiv"
<div id="text/javascript">
<h3> <?php echo"$divId";?> <h3>
</div>
</body>
Output:
We create an HTML page in the above code and pass the variable $divId
as our div id
.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn