How to Create and Use Static Classes in PHP
- Understanding Static Classes
- Creating Static Methods
- Using Static Properties
- Best Practices for Static Classes
- Conclusion
- FAQ
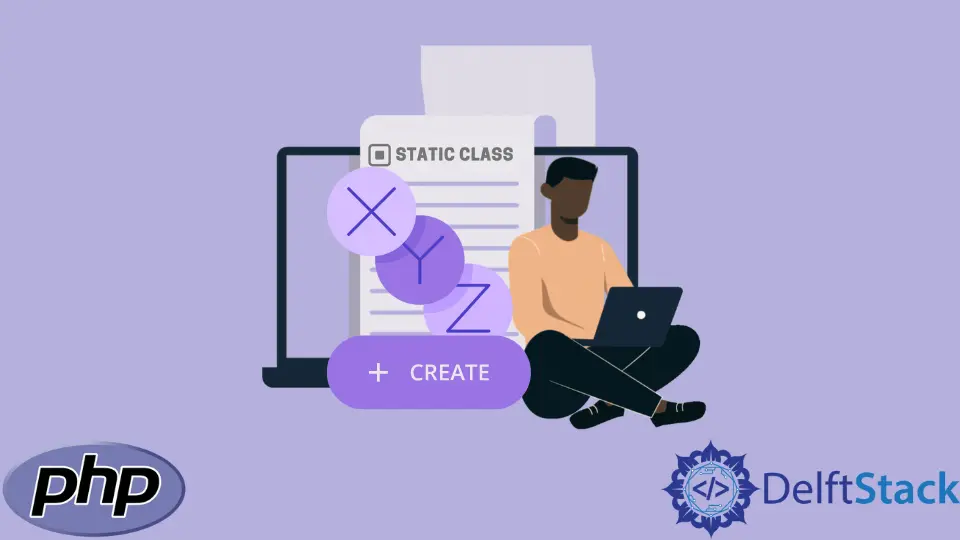
Creating and using static classes in PHP can be a powerful way to organize your code and manage shared resources. Static classes allow you to access methods and properties without needing to instantiate an object, leading to cleaner and more efficient code.
This article will guide you through the process of creating static classes, explaining their benefits and providing clear examples to illustrate their usage. Whether you’re a beginner or an experienced developer looking to refine your skills, this guide will help you understand how to leverage static classes effectively in PHP.
Understanding Static Classes
Static classes in PHP are classes that cannot be instantiated. Instead, all members (properties and methods) are accessed statically. This means you can call them directly on the class itself without needing to create an object. Static classes are particularly useful for utility functions or when you want to maintain state across different parts of your application without creating multiple instances.
To define a static class, you typically use the static
keyword. Here’s a simple example:
class MathUtilities {
public static function add($a, $b) {
return $a + $b;
}
}
In this example, the MathUtilities
class has a static method called add
. You can call this method without creating an instance of the class:
$result = MathUtilities::add(5, 10);
Output:
15
Static classes are great for grouping related functions together, making your code more organized. They also help in reducing memory usage since you don’t need to create an object for each method call. However, it’s essential to use them wisely, as overusing static methods can lead to code that is hard to test and maintain.
Creating Static Methods
Creating static methods in PHP is straightforward. You simply declare a method with the static
keyword. This allows you to call the method directly on the class without instantiation. Here’s how you can create and use static methods effectively:
class StringUtilities {
public static function toUpperCase($string) {
return strtoupper($string);
}
}
You can call the toUpperCase
method like this:
$upperString = StringUtilities::toUpperCase("hello world");
Output:
HELLO WORLD
In this example, the StringUtilities
class contains a static method that converts a string to uppercase. By using static methods, you can easily manipulate data without needing to create an object. This approach is particularly beneficial when you have utility functions that don’t require object state.
However, remember that static methods can make unit testing more challenging. They are tightly coupled to the class itself, making it harder to mock or replace them in tests. Therefore, use static methods judiciously, focusing on utility functions or shared resources.
Using Static Properties
Static properties in PHP can store values that are shared across all instances of a class. This can be particularly useful for maintaining state or configuration data. Here’s how to define and use static properties:
class Configuration {
public static $version = "1.0.0";
public static function getVersion() {
return self::$version;
}
}
You can access the static property and method like this:
$currentVersion = Configuration::getVersion();
Output:
1.0.0
In this example, the Configuration
class has a static property called $version
. The getVersion
method returns the value of this property. Since the property is static, it can be accessed without creating an instance of the class. This is particularly useful for configuration settings that should remain constant throughout your application.
Static properties also allow you to maintain state across different calls. For instance, you could modify the $version
property and have that change reflected across all calls to getVersion
. However, be cautious about modifying static properties, as they can lead to unexpected behavior if not managed properly.
Best Practices for Static Classes
When working with static classes in PHP, adhering to best practices can help you write cleaner, more maintainable code. Here are some tips to keep in mind:
-
Limit Usage: Use static classes for utility functions or shared resources. Avoid making everything static, as it can lead to tightly coupled code that is hard to test.
-
Naming Conventions: Name your static classes clearly to indicate their purpose. For example,
MathUtilities
orStringUtilities
are descriptive and convey their functionality. -
Avoid State: Try to avoid using static properties to maintain state unless necessary. If you need to maintain state, consider using singleton patterns or dependency injection instead.
-
Testing: Be aware that static methods can complicate testing. If you find yourself needing to mock static methods frequently, it might be a sign to refactor your code.
By following these best practices, you can make the most of static classes while minimizing potential pitfalls.
Conclusion
Static classes in PHP offer a powerful way to create organized, efficient code. By understanding how to create and use static methods and properties, you can streamline your development process and manage shared resources effectively. However, it’s essential to use static classes judiciously, keeping in mind the best practices to maintain code quality. As you continue to explore PHP, incorporating static classes into your toolkit will enhance your programming skills and help you write cleaner, more maintainable applications.
FAQ
-
What are static classes in PHP?
Static classes are classes that cannot be instantiated and have members accessed statically using the class name. -
How do you create a static method in PHP?
You create a static method by declaring it with thestatic
keyword, allowing it to be called without an instance of the class. -
Can you modify static properties in PHP?
Yes, you can modify static properties, but be cautious, as they maintain state across all calls to the class. -
What are the advantages of using static classes?
Static classes help organize utility functions and reduce memory usage since you don’t need to instantiate objects. -
Are static methods suitable for unit testing?
Static methods can complicate unit testing because they are tightly coupled to the class itself, making it harder to mock or replace them.