How to Create a Signature From Hash_hmac() and Sha256 in PHP
-
the
hash_hmac()
Function in PHP -
Use
hash_hmac()
Withsha256
in PHP -
Use
hash_hmac()
Withbase64_encode()
-
Use
hash_hmac()
Withsha256
and Applyhex2bin
/bin2hex
Conversions in PHP
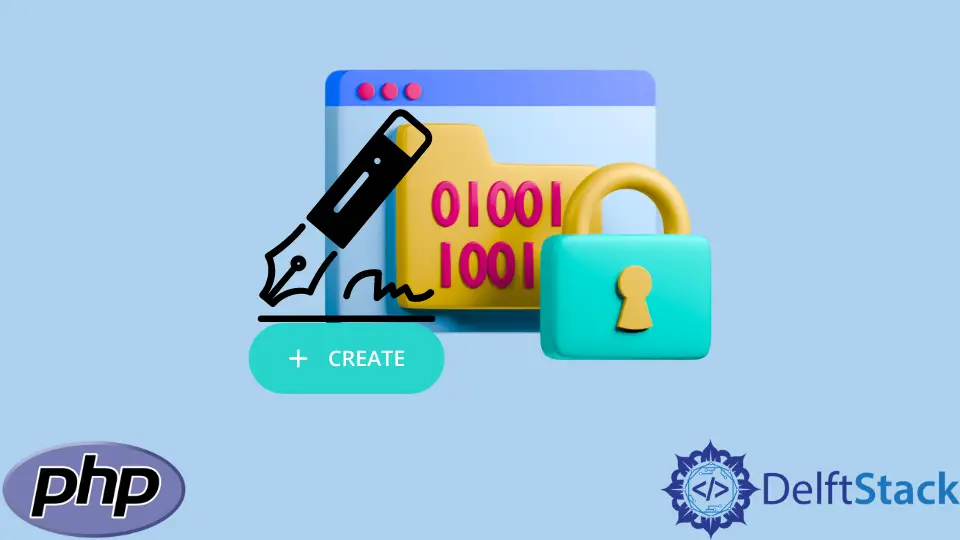
PHP has one of the best encryption functions used for data security. The Hash_hmac()
encryption function is one of the best-known encrypters.
We will show you how to use hash_hmac
with the sha256
encrypter to create a secure signature
, a secret key that you can store in the database or use in your login forms.
the hash_hmac()
Function in PHP
The hash_hmac()
creates an alphanumeric keyed secret hash value. It utilizes the cryptographic authentication technique known as the HMAC
method.
Syntax:
hash_hmac($algo, $data, $key, $binary = false);
- The
$algo
is one of the hash_hmac parameters, used as a signature secret keyed hash value (String
). - The
$data
, a hashed value (usually users’ passwords). It can be alphanumeric. - The
$key
is generated from theHMAC
method, a secret key, which you get from the function, and other programming uses. - The final parameter
$binary
is the binary value.
It returns raw data when TRUE
and throws lowercase hexits when FALSE
.
Use hash_hmac()
With sha256
in PHP
Example:
<?php
//sha256 is a $algo
$Password= 'ThisIS123PasswORD' ; //data
$secret ="ABCabc"; //$key
//false is bool value to check whether the method works or not
$hash_it = hash_hmac("sha256", utf8_encode($Password), $secret, false); //all four parameters
if(!$hash_it){ //checks true or false
echo "Password was not encrypted!";
}
echo "Your encrypted signature is:<b> '.$hash_it.' </b>";
?>
Output:
Your encrypted signature is: '.46e9757dc98f478c28f035cfa871dbd19b5a2bf8273225191bcca78801304813
Use hash_hmac()
With base64_encode()
If you want your encrypted signature to be more secure, you can also do the following code snippet.
Example:
<?php
$my_sign = "abc123";
$key = TRUE;
$base64_encode = base64_encode(hash_hmac('sha256', $my_sign, $key, true));
if(!$base64_encode){
echo "Your signature with the base64_decode(hash_hmac()); failed";
}
else{
echo "Your base64_decode(hash_hmac()); encrypted signature is is:<b> $base64_encode.' </b>";
}
?>
Output:
Your base64_decode(hash_hmac()); encrypted signature is is: '.00g0QmqlnluXxijqot60WZf6InDi07b/Mb5lL7eVZ34
Use hash_hmac()
With sha256
and Apply hex2bin
/bin2hex
Conversions in PHP
hex2bin()
- This function decodes a binary string that has been encoded in hexadecimal.bin2hex()
- A string of ASCII characters is converted to hexadecimal values using thebin2hex()
method.
Suppose you have critical financial data that you want to encrypt and create a secret key for the user.
Example:
<?php
$createhashmackey = "2974924872949278487322348237749823";
$bank_acc = "029480247299262947328749287";
$current_bal = $sum * 10000 / 450;
$bank_locker_no = 'AC54-A76X';
$last_deposit = $when;
$se = hex2bin($createhashmackey);
$create_his_signature = $bank_acc . $current_bal. $bank_locker_no . $last_deposit;
$enigma = bin2hex(hash_hmac('sha256', $create_his_signature, $se));
echo "The secret signature is.$enigma.";
Output:
The secret signature is.33633066323533383537663538323937373536393764396530343661663336666438636435363631383934363864383966316133633433633965343234336233.
Here is the output of all the code examples in this tutorial.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn