How to Push Both Value and Key Into PHP Array
- Push Key and Value to PHP Array Using Square Bracket Array Initialization Method
-
Push Key and Value to PHP Array Using Array
object
-
Push Key and Value to PHP Array Using
array_merge
Method - Push Key and Value to PHP Array Using Compound Assignment Operators
-
Push Key and Value to PHP Array Using
parse_str
Method -
Push Key and Value to PHP Array Using
array_push
Method
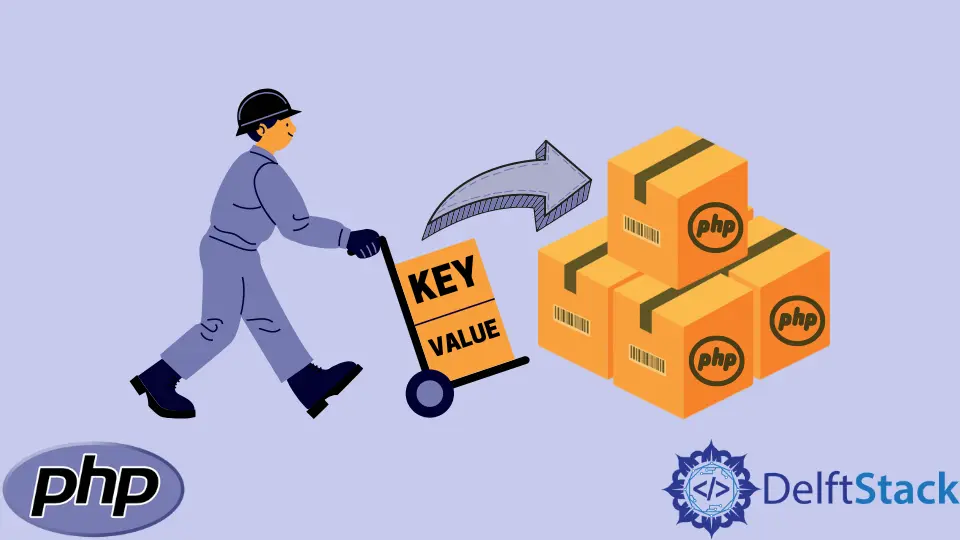
We will look at the method to push a key and corresponding value to a PHP array using the square bracket array initialization method.
We will look at different ways to push a key and corresponding value to a PHP array using the array_merge
method, the array object
, the compound assignment operators, the parse_str
method and the array_push
method.
Push Key and Value to PHP Array Using Square Bracket Array Initialization Method
We will initialize an empty array then add a key-value pair to the square brackets initialization.
<?php
$array_test = [];
$array_test['name'] = "Kevin Amayi";
print_r($array_test);
?>
Output:
Array ( [name] => Kevin Amayi )
Push Key and Value to PHP Array Using Array object
We will initialize an array with the corresponding key and value using the array object
.
<?php
$array_test = array("firstname" => "Kevin","lastname" => "Amayi");
print_r($array_test);
?>
Output:
Array ( [firstname] => Kevin [lastname] => Amayi )
Push Key and Value to PHP Array Using array_merge
Method
We will directly initialize an array with two values then use the array_merge
method to add a new value with the corresponding key.
<?php
$array_test = array("firstname" => "Kevin","lastname" => "Amayi");
$array_test = array_merge($array_test, ['occupation1' => "blogger"]);
$array_test = array_merge($array_test, ['occupation2' => "programmer"]);
print_r($array_test);
?>
Output:
Array ( [firstname] => Kevin [lastname] => Amayi [occupation1] => blogger [occupation2] => programmer )
Push Key and Value to PHP Array Using Compound Assignment Operators
We will initialize an empty array then use compound assignment to add new key-value pair in the array.
<?php
$profile = [];
$profile += [ "name" => "Kevin" ];
$profile += [ "Age" => 23 ];
$profile += [ "Hobby" => "Football" ];
print_r($profile);
?>
Output:
Array ( [name] => Kevin [Age] => 23 [Hobby] => Football )
Push Key and Value to PHP Array Using parse_str
Method
We will initialize an empty array then use the parse_str
method to add new key-value pair in the array.
<?php
$profile = [];
parse_str("name=Kevin&age=23",$profile);
print_r($profile);
?>
Output:
Array ( [name] => Kevin [age] => 23 )
Push Key and Value to PHP Array Using array_push
Method
We will directly initialize an array with two values then use the array_push
method to add a new value with the corresponding key.
<?php
$array_test = array("Kevin", "Amayi");
array_push($array_test, ["occupation1" => "Blogger"],["occupation2" => "Programmer"]);
print_r($array_test);
?>
Output:
Array ( [0] => Kevin [1] => Amayi [2] => Array ( [occupation1] => Blogger ) [3] => Array ( [occupation2] => Programmer) )