How to Set End of Line in PHP With PHP_EOL
Kevin Amayi
Feb 02, 2024
-
Set End of Line Breakpoints Using
PHP_EOL
Constant in Array Data -
Set End of Line Breakpoints Using
PHP_EOL
Constant in File Data
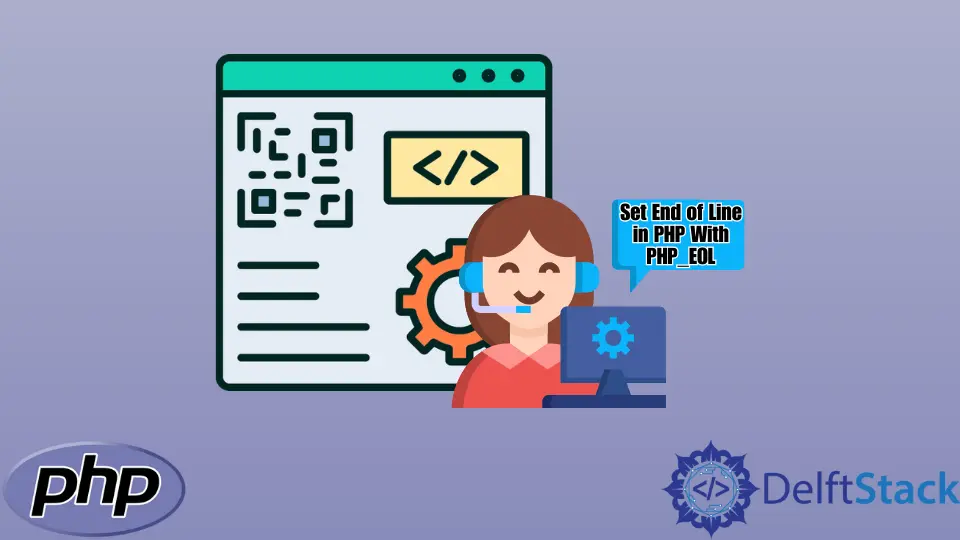
We will look at how to use PHP_EOL
to insert the correct end of line symbol for the different platforms on Windows.
PHP_EOL
will return \r\n
, and on a Unix system, it will return \n
. We will look at how to use PHP_EOL
to insert the correct end of line symbol for the different platforms in a file
.
Set End of Line Breakpoints Using PHP_EOL
Constant in Array Data
We will create an array and then loop through the array and display each item in a new line by applying the PHP_EOL
to insert a new line character at the end of the line.
<?php
$profile = array('Name'=>'Kevin', 'Occupation'=>'Programmer', 'Hobby'=>'Football');
// Multiple lines by PHP_EOL
reset( $profile );
while ( list($key, $value) = each( $profile ) ){
echo ("$key => $value" . PHP_EOL);
}
?>
Output:
Name => Kevin
Occupation => Programmer
Hobby => Football
Set End of Line Breakpoints Using PHP_EOL
Constant in File Data
We will create two-string data and then use the PHP_EOL
constant to insert a new line character at each string’s end before outputting the lines in a file.
<?php
$text = 'Test one' . PHP_EOL;
$text .= 'Test two' . PHP_EOL;
$file = fopen('test_file.txt', 'w');
fputs($file, $text);
fclose($file);echo $text;
?>
Output:
Test one
Test two