How to Create a Zip File in PHP
- Creating a Zip File in PHP
- Adding Files from a Folder to a Zip File
- Unzipping a Zip File in PHP
- Conclusion
- FAQ
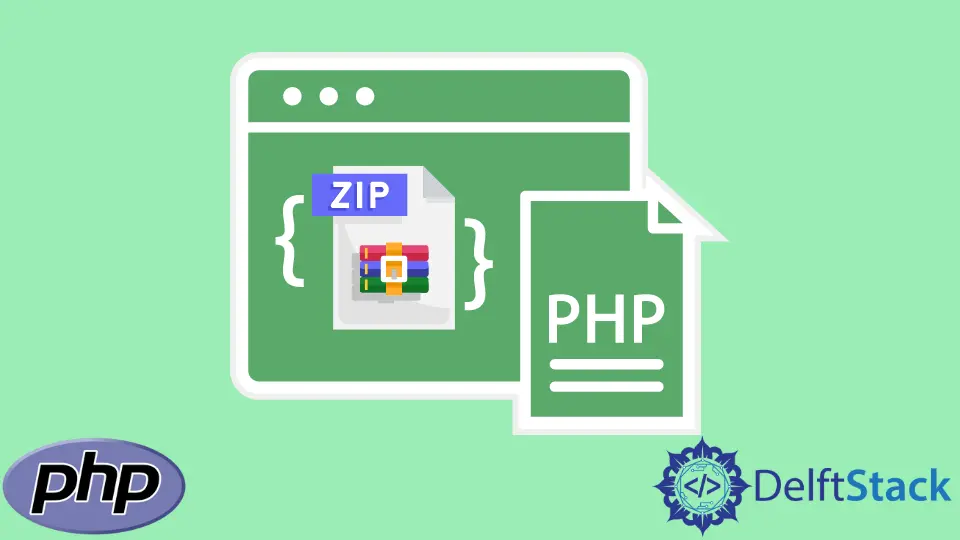
Creating a zip file in PHP can be a straightforward process, and it’s incredibly useful for developers looking to manage files more efficiently. Whether you’re compressing multiple files to save space or bundling resources for download, PHP provides robust tools to handle zip file creation and extraction.
In this tutorial, we’ll walk through the steps to create a zip file, add files from a folder, and unzip that file using PHP. By the end of this guide, you’ll have a solid understanding of how to work with zip files in your PHP projects. Let’s dive in!
Creating a Zip File in PHP
To create a zip file in PHP, you can utilize the built-in ZipArchive
class. This class allows you to open, create, and manipulate zip files easily. The first step involves creating an instance of the ZipArchive
class and then using its methods to add files or directories to the zip file.
Here’s a simple example of how to create a zip file and add files to it:
<?php
$zip = new ZipArchive();
$filename = "example.zip";
if ($zip->open($filename, ZipArchive::CREATE) !== TRUE) {
exit("Cannot open <$filename>\n");
}
$zip->addFile("file1.txt");
$zip->addFile("file2.txt");
$zip->addFile("folder/file3.txt");
$zip->close();
echo "Zip file created successfully.";
?>
Output:
Zip file created successfully.
In this code snippet, we first create a new instance of ZipArchive
. We then attempt to open a zip file named example.zip
for creation. If the file cannot be opened, we exit with an error message. After that, we use the addFile
method to include specific files in the zip archive. Finally, we close the zip file using the close
method, and a success message is displayed.
This method is perfect for situations where you want to bundle multiple files into a single zip file for easier management or download.
Adding Files from a Folder to a Zip File
If you want to add all files from a directory into a zip file, you can use a loop to iterate through the directory’s contents. This is a great way to compress entire folders without having to manually specify each file.
Here’s how you can do it:
<?php
$zip = new ZipArchive();
$filename = "folder_example.zip";
if ($zip->open($filename, ZipArchive::CREATE) !== TRUE) {
exit("Cannot open <$filename>\n");
}
$folderPath = 'my_folder/';
$files = scandir($folderPath);
foreach ($files as $file) {
if ($file != '.' && $file != '..') {
$zip->addFile($folderPath . $file);
}
}
$zip->close();
echo "All files from the folder added to zip successfully.";
?>
Output:
All files from the folder added to zip successfully.
In this example, we again create an instance of ZipArchive
and attempt to open a new zip file named folder_example.zip
. We use scandir
to get all files in the specified directory, my_folder
. A loop iterates through the files, and we check to ensure that we don’t attempt to add the .
and ..
entries, which represent the current and parent directories. Each valid file is added to the zip archive. Finally, we close the zip file and print a success message.
This method is particularly useful for developers who need to compress an entire folder quickly.
Unzipping a Zip File in PHP
Unzipping or extracting files from a zip archive is just as easy with PHP’s ZipArchive
class. You can specify a target directory where the contents of the zip file will be extracted. Here’s how to do it:
<?php
$zip = new ZipArchive();
$filename = "example.zip";
if ($zip->open($filename) === TRUE) {
$zip->extractTo('extracted_files/');
$zip->close();
echo "Files extracted successfully.";
} else {
exit("Failed to open <$filename>\n");
}
?>
Output:
Files extracted successfully.
In this code snippet, we again create an instance of ZipArchive
and attempt to open the existing zip file, example.zip
. If the file opens successfully, we use the extractTo
method to specify a directory (extracted_files/
) where the contents will be extracted. After closing the zip file, we display a success message. If the zip file cannot be opened, an error message is displayed instead.
This method is essential for scenarios where users need to access the contents of a zip file after downloading it.
Conclusion
Creating and managing zip files in PHP is a powerful feature that can enhance your web applications. By using the ZipArchive
class, you can easily create zip files, add multiple files or entire directories, and extract files as needed. This tutorial has provided you with the foundational knowledge to implement zip file functionality in your PHP projects. Whether you’re compressing files for download or organizing resources efficiently, mastering zip file manipulation will undoubtedly improve your development skills.
FAQ
-
How do I create a zip file in PHP?
You can create a zip file in PHP using the ZipArchive class. Initialize an instance, open a zip file, and use the addFile method to include files. -
Can I add an entire folder to a zip file in PHP?
Yes, you can use a loop to iterate through the files in a folder and add them to a zip file using the addFile method. -
How do I unzip a file in PHP?
To unzip a file in PHP, use the ZipArchive class to open the zip file and the extractTo method to specify where to extract the files.
-
What happens if the zip file cannot be opened?
If the zip file cannot be opened, you can handle it by checking the return value of the open method and displaying an error message. -
Is there a limit to the size of files I can add to a zip file in PHP?
While there is no strict limit imposed by PHP, you may encounter server limitations based on memory or file size restrictions. Always check your server configuration.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn