var_dump() PHP Built-In Function
-
Use the
var_dump()
to Retrieve the Information of an Integer -
Use the
var_dump()
to Retrieve the Information of a String -
Use the
var_dump()
to Retrieve the Information of an Array and an Object -
Use the
var_dump()
to Retrieve the Information of Multiple Variables
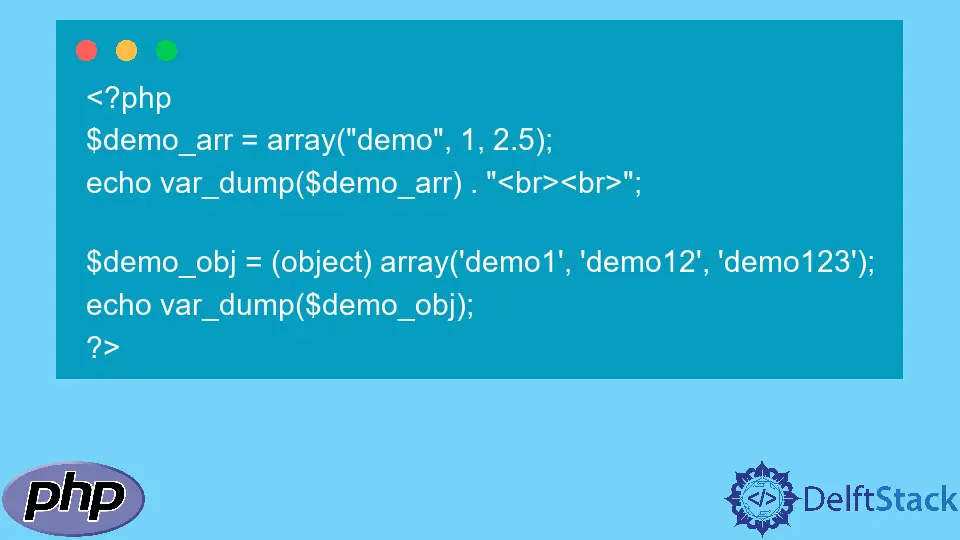
The built-in PHP function var_dump()
can retrieve the information of both scalar and compound variables.
For each variable, it will show the data type and value of the variable.
For a string variable, the var_dump()
will also retrieve the length or size of the string, and if the variable is an array or an object, the information will be shown recursively.
This tutorial demonstrates how PHP var_dump()
can retrieve all the structural information of different data types.
Use the var_dump()
to Retrieve the Information of an Integer
The var_dump takes the variable as a parameter. Let’s see the example for an integer.
<?php
$demo = 10;
echo var_dump($demo);
?>
Output:
int(10)
The output shows the value in parenthesis with the data type int. It will also show output for float and boolean data types similarly.
Use the var_dump()
to Retrieve the Information of a String
As mentioned above, the var_dump()
will also retrieve the length or size of the string. See example:
<?php
$demo= "This is php var_dump test";
echo var_dump($demo);
?>
Output:
string(25) "This is php var_dump test"
The output is the data type, length in parenthesis, and the string value for the string.
Use the var_dump()
to Retrieve the Information of an Array and an Object
For an array or an object, var_dump()
will retrieve the information about each element. See example:
<?php
$demo_arr = array("demo", 1, 2.5);
echo var_dump($demo_arr) . "<br><br>";
$demo_obj = (object) array('demo1', 'demo12', 'demo123');
echo var_dump($demo_obj);
?>
Output:
array(3) { [0]=> string(4) "demo" [1]=> int(1) [2]=> float(2.5) }
object(stdClass)#1 (3) { [0]=> string(5) "demo1" [1]=> string(6) "demo12" [2]=> string(7) "demo123" }
For an array, the var_dump()
shows the type and length of the array itself and each element’s type, value, and length. For object, it also shows the stdClass, an empty class used for casting other types to an object; other than that, the output is similar to the array.
Use the var_dump()
to Retrieve the Information of Multiple Variables
The var_dump()
can dump the information of multiple variables at once by just putting multiple parameters. See example:
<?php
$demo1 = true;
$demo2 = 10;
echo var_dump($demo1, $demo2);
?>
Output:
bool(true) int(10)
As we see for multiple variables, the var_dump()
shows each variable’s type and value in one line divided by space.
The var_dump()
doesn’t have any return type, and it is supported by all PHP 4.0 and above versions.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook