The utf8_encode Function in PHP
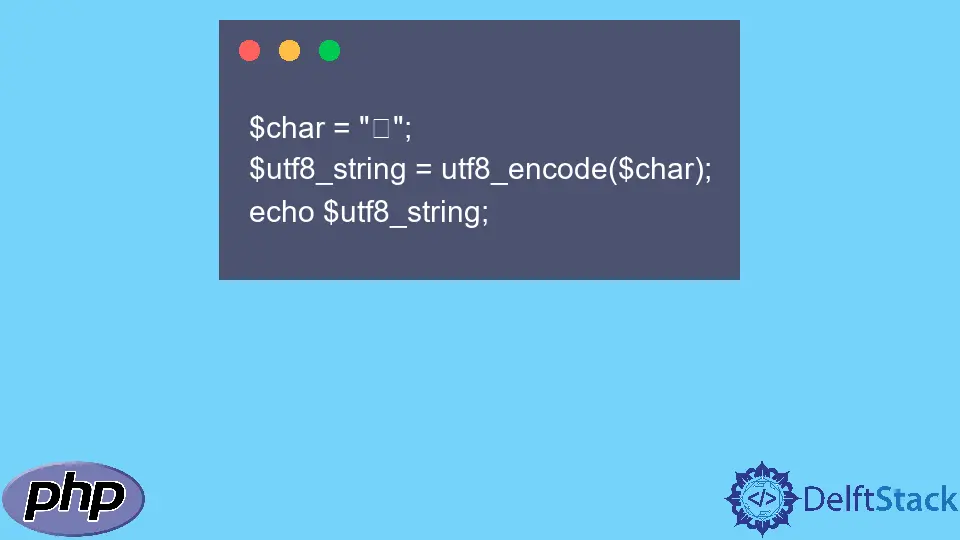
In the world of web development, encoding and decoding data correctly is crucial for ensuring that applications function seamlessly, especially when dealing with different character sets. One such function that comes in handy in PHP is utf8_encode
. This function is designed to convert ISO-8859-1 encoded strings to UTF-8, making it easier to handle multilingual content and special characters. Whether you’re a seasoned developer or a beginner, understanding how to use utf8_encode
can significantly enhance your web applications.
In this article, we will delve into the purpose of the utf8_encode
function, how it works, and provide practical examples to illustrate its usage.
Understanding the utf8_encode Function
The utf8_encode
function in PHP is specifically designed to convert a string encoded in ISO-8859-1 (also known as Latin-1) to UTF-8 encoding. UTF-8 is a more versatile encoding system that can represent any character in the Unicode standard, making it the preferred choice for modern web applications.
This function is particularly useful when dealing with databases or external data sources that may return strings in ISO-8859-1 format. By converting these strings to UTF-8, developers can ensure that their applications display characters correctly, avoiding issues like garbled text or question marks in place of special characters.
Basic Usage of utf8_encode
Using the utf8_encode
function is straightforward. You simply pass the ISO-8859-1 encoded string as an argument, and it returns the UTF-8 encoded version. Here’s a simple example to illustrate how it works:
$originalString = "Café";
$utf8String = utf8_encode($originalString);
echo $utf8String;
Output:
Café
In this example, the string “Café” is already in a format that can be encoded. The utf8_encode
function processes it and returns the same string in UTF-8 format. This is a basic demonstration, but it highlights the function’s utility in ensuring that special characters are preserved correctly.
Converting Strings with utf8_encode
When working with data from various sources, you may encounter strings that need conversion. The utf8_encode
function can simplify this process. Here’s a more detailed example that shows how to handle a string with multiple special characters:
$originalString = "São Paulo é uma cidade linda.";
$utf8String = utf8_encode($originalString);
echo $utf8String;
Output:
São Paulo é uma cidade linda.
In this case, the original string contains several special characters, including “ã,” “é,” and “ç.” By using utf8_encode
, these characters are correctly represented in UTF-8 format, ensuring that they display properly in web applications.
Handling Data from External Sources
Often, data is retrieved from databases or APIs, and it may not always be in the desired encoding. This is where utf8_encode
shines. Here’s an example of how you might use it when fetching data from a database:
$conn = new mysqli("localhost", "username", "password", "database");
$query = "SELECT name FROM users";
$result = $conn->query($query);
while ($row = $result->fetch_assoc()) {
$name = utf8_encode($row['name']);
echo $name . "<br>";
}
Output:
José
Ana
Müller
In this example, we connect to a MySQL database, fetch user names, and convert them to UTF-8 using utf8_encode
. This ensures that names with special characters, such as “é” and “ü,” are displayed correctly.
Conclusion
The utf8_encode
function in PHP is a powerful tool for developers dealing with character encoding issues. Its ability to convert ISO-8859-1 strings to UTF-8 ensures that web applications can handle multilingual content and special characters without any hiccups. By understanding how to use this function effectively, you can enhance your applications’ robustness and user experience.
In a world where content is global, having a solid grasp of encoding functions like utf8_encode
will undoubtedly make your development process smoother. Whether you’re working with databases, APIs, or user input, ensuring that your strings are correctly encoded is essential for delivering a seamless experience to users.
FAQ
-
What is the purpose of the utf8_encode function in PHP?
The utf8_encode function converts ISO-8859-1 encoded strings to UTF-8 encoding, ensuring proper representation of special characters. -
Can utf8_encode handle strings with special characters?
Yes, utf8_encode effectively handles strings with special characters, converting them to UTF-8 format for correct display.
-
Is utf8_encode the only way to handle string encoding in PHP?
No, there are other functions like utf8_decode and mb_convert_encoding that can also be used for various encoding tasks. -
When should I use utf8_encode?
Use utf8_encode when you need to convert ISO-8859-1 strings to UTF-8, especially when dealing with databases or external data sources. -
What happens if I use utf8_encode on a string that is already in UTF-8?
Using utf8_encode on an already UTF-8 encoded string can result in corrupted data, so it’s essential to ensure the string’s encoding before applying the function.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn