The use Keyword in PHP
-
Introduction and Implementation of
namespace
in PHP -
Implement
use
Along Withnamespace
in PHP -
Group Multiple Class With
use
in PHP
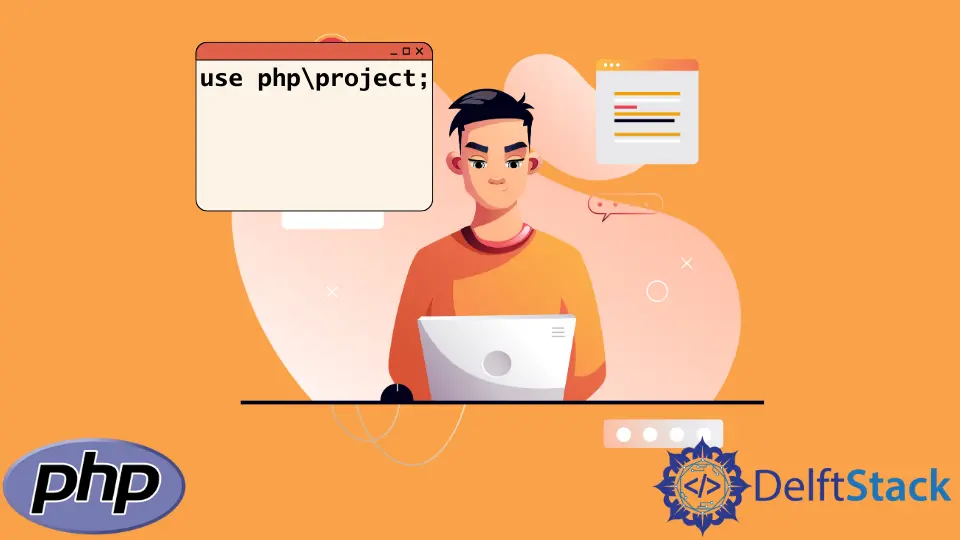
This article will introduce use
and namespace
in PHP. Then, we will walk through the implementation of these keywords by creating a small project.
Introduction and Implementation of namespace
in PHP
A namespace in PHP is a label that holds a block of code. We can use the namespace to access a particular code block from other locations in the project.
For example, a namespace can hold the block of codes like classes, functions, constants, etc.
Namespace mainly solves two problems. These are:
- Namespace avoids the name collision among the classes or the functions. For instance, there might be ambiguity when the user-defined function matches the name of the core PHP functions or the library functions.
- Namespace allows better communication and organization between the modules during the project. We can alias the components for better readability.
namespace
keyword indicates the namespace, and it should be the first line of code in the PHP file.Let’s see an example of how namespace works. Create a class Greetings
and write a constructor in it.
Display a message Hello everyone!
inside the constructor. Save the file as greetings.php
.
Next, create another file in the same directory as index.php
. Firstly, use the require
function to require the greetings.php
.
Then, create a variable $hello
and instantiate with the Greetings
class instance as $hello = new Greetings
.
When we serve the index.php
file, it gives an error, Class 'Greetings' not found
. To solve the problem, we can use the namespace.
For that, create a namespace subodh\project
in the greetings.php
file. Next, in the index.php
file, use the namespace subodh\project
ahead of the class Greetings
.
This time, the message Hello everyone!
is displayed. This is how namespace can be used to organize the components in a project.
We can also use namespace to organize the functions and variables similarly.
namespace subodh\project;
class Greetings{
public function __construct(){
print("Hello everyone!")."<br>";
}
}
function greet(){
print("Good Morning!")."<br>";
}
const greeting = "have a nice day"."<br>";
require 'greetings.php';
$hello = new subodh\project\Greetings;
subodh\project\greet();
echo subodh\project\greeting;
Output:
Hello everyone!
Good Morning!
have a nice day
Implement use
Along With namespace
in PHP
We can use the use
keyword in PHP to import and give an alias to namespace
in PHP. As a result, we can replace the long namespace with the short alias.
This improves the readability of the code. We can use the alias to indicate the namespace.
Firstly, we will use the use
keyword to create an alias of the namespace of the example code written above.
For example, in the index.php
file, write the use
keyword to import the namespace written in the greetings.php
file as use subodh\project
.
It means that now we can use project
to access the class, function and the constant, as in the example below.
require 'greetings.php';
use subodh\project;
$hello = new project\Greetings;
project\greet();
echo project\greeting;
We can also create a custom alias as follows.
require 'greetings.php';
use subodh\project as pr;
$hello = new pr\Greetings;
pr\greet();
echo pr\greeting;
We can also import the classes, functions and constants using the use
keyword. We can write the namespace after the use
keyword for class.
First, we should append the class name at the end of the namespace. Then, we can directly access the class.
In the case of functions and constant, we should write the keywords function
and constant
respectively after the use
keyword.
After that, we can write the namespace appending the name of the function and constant. The example is shown below.
use subodh\project\Greetings;
$hello = new Greetings;
use function subodh\project\greet;
greet();
use const subodh\project\greeting;
echo greeting;
The output of all the methods above is the same.
Output:
Hello everyone!
Good Morning!
have a nice day
Group Multiple Class With use
in PHP
As introduced in PHP7, we can group the classes, functions, and constants while using the use
keyword.
This feature prevents the multiple uses of the use
keywords and makes the code cleaner and easy to understand.
The line of the code is also decreased, and reusability is maintained. Let’s consider the following vehicle.php
file.
It contains two classes, Car
and Motorcycle
with constructors. In addition, we have created the namespace subodh\project
.
namespace subodh\project;
class Car{
public function __construct(){
print("This is Car class")."<br>";
}
}
class Motorcycle{
public function __construct(){
print("This is Motorcycle class")."<br>";
}
}
We can use the use
keyword once to import both classes as the same namespace. We can include the class names in the curly braces after the namespace.
We can even create an alias for the class. Commas separate the class names.
For example, write the namespace subodh\project\{}
after the use
keyword. Then, inside the curly brace, write the class Car
and write the class Motorcycle
after a comma.
Finally, write the alias bike
for the Motorcycle
class. Now, we can create the objects of these classes by instantiating Car
and bike
with the new
keyword.
require('vehicle.php');
use subodh\project\{Car, Motorcycle as bike};
$car = new Car;
$bike = new bike;
Output:
This is Car class
This is Motorcycle class
Thus, we can use the use
keyword to group the classes in a namespace in PHP. We also can similarly group the functions and constants.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn