PHP URL Decoding
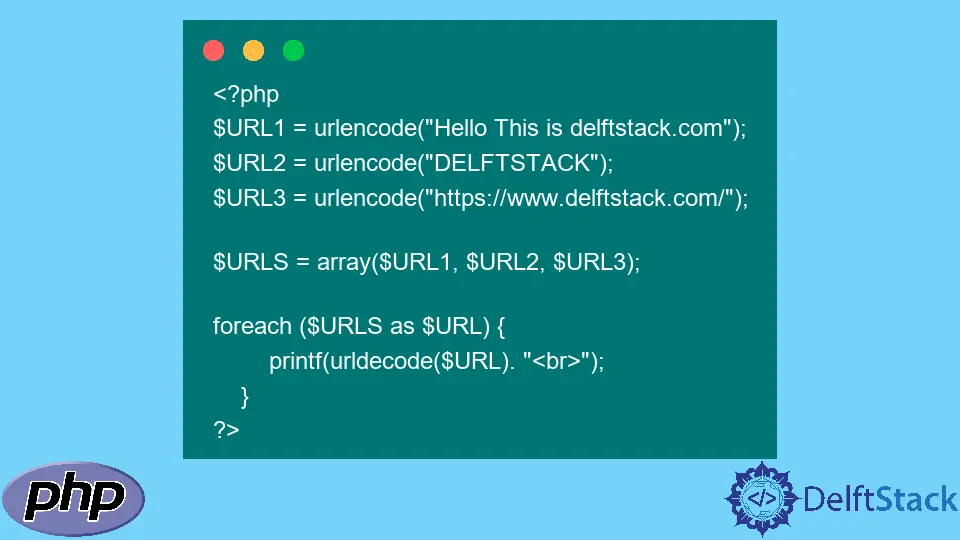
The method urldecode()
is used to decode an encoded string in PHP. This tutorial will demonstrate using PHP’s urldecode()
method.
PHP URL Decoding
The urldecode()
is a built-in method in PHP used to decode the encoded strings and URLs. The urldecode()
can only decode the encoded strings and URLs done by urlencode()
method.
The syntax for this method is:
string urldecode( URL )
Where URL
is the URL or string to be decoded, the return value for this method is a string. Let’s try an example for the urldecode()
method:
<?php
$URL1 = urlencode("Hello This is delftstack.com");
$URL2 = urlencode("DELFTSTACK");
$URL3 = urlencode("https://www.delftstack.com/");
echo $URL1. " <br>";
echo urldecode($URL1). "<br>";
echo $URL2. "<br>";
echo urldecode($URL2). "<br>";
echo $URL3. "<br>";
echo urldecode($URL3). "<br>";
?>
The code above first uses the urlencode()
method to encode the URLs and strings and then uses the urldecode()
method to decode them. See output:
Hello+This+is+delftstack.com
Hello This is delftstack.com
DELFTSTACK
DELFTSTACK
https%3A%2F%2Fwww.delftstack.com%2F
https://www.delftstack.com/
To decode multiple URLs, we can use an array and the foreach
loop on the urldecode()
method. See example:
<?php
$URL1 = urlencode("Hello This is delftstack.com");
$URL2 = urlencode("DELFTSTACK");
$URL3 = urlencode("https://www.delftstack.com/");
$URLS = array($URL1, $URL2, $URL3);
foreach ($URLS as $URL) {
printf(urldecode($URL). "<br>");
}
?>
The code above will decode multiple URLs using an array and the foreach
loop on the urldecode()
method. See output:
Hello This is delftstack.com
DELFTSTACK
https://www.delftstack.com/
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook