PHP Upload Image
-
Enable File Upload From
php.ini
File to Upload an Image in PHP - Use File Upload Operations to Upload an Image in PHP
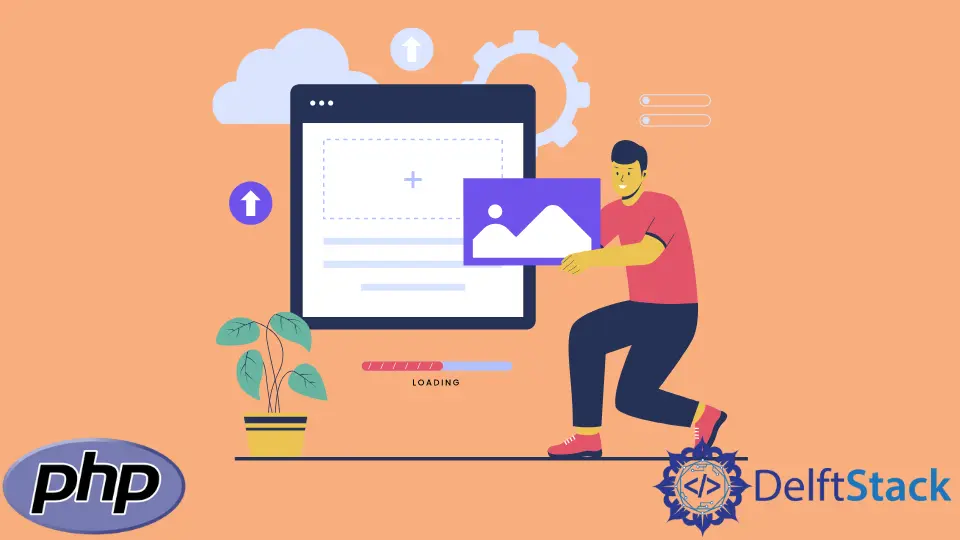
We can upload images in PHP using simple file upload operations, but first, file uploads should be enabled from the php.ini
file. This tutorial demonstrates how to upload an image in PHP.
Enable File Upload From php.ini
File to Upload an Image in PHP
For newer versions of PHP, the file upload default is on
. You can also edit file upload configuration from the php.ini
file.
Below is how configurations are set.
Allow HTTP file uploads.
file_uploads = On
Set the maximum allowed size for uploaded files.
upload_max_filesize = 2M
Set the maximum number of files uploaded via a single request.
max_file_uploads = 20
Use File Upload Operations to Upload an Image in PHP
The image size should be under 2 megabytes per the above configurations. The code below has a validation to check if the selected file is an image.
<form action="" method="post" enctype="multipart/form-data">
<input type="file" name="image" >
<input type="submit" value="upload" name="upload">
</form>
<?php
if(isset($_POST['upload'])){
$input_image=$_FILES['image']['name'];
$image_info = @getimagesize($input_image);
if($image_info == false){
echo "The selected file is not image.";
}
else{
$image_array=explode('.',$input_image);
$rand=rand(10000,99999);
$image_new_name=$image_array[0].$rand.'.'.$image_array[1];
$image_upload_path="uploads/".$image_new_name;
$is_uploaded=move_uploaded_file($_FILES["image"]["tmp_name"],$image_upload_path);
if($is_uploaded){
echo 'Image Successfully Uploaded';
}
else{
echo 'Something Went Wrong!';
}
}
}
?>
The code above uploads a file through a form on the same page. First, it validates if the selected file is an image and then uploads it.
Output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook