How to Create and Get the Path of tmpfile in PHP
-
Use the
stream_get_meta_data()
Function anduri
Index to Create and Get the Path oftmpfile
in PHP -
Use the
tempnam()
andsys_get_temp_dir()
Functions to Create and Get the Path oftmpfile
in PHP
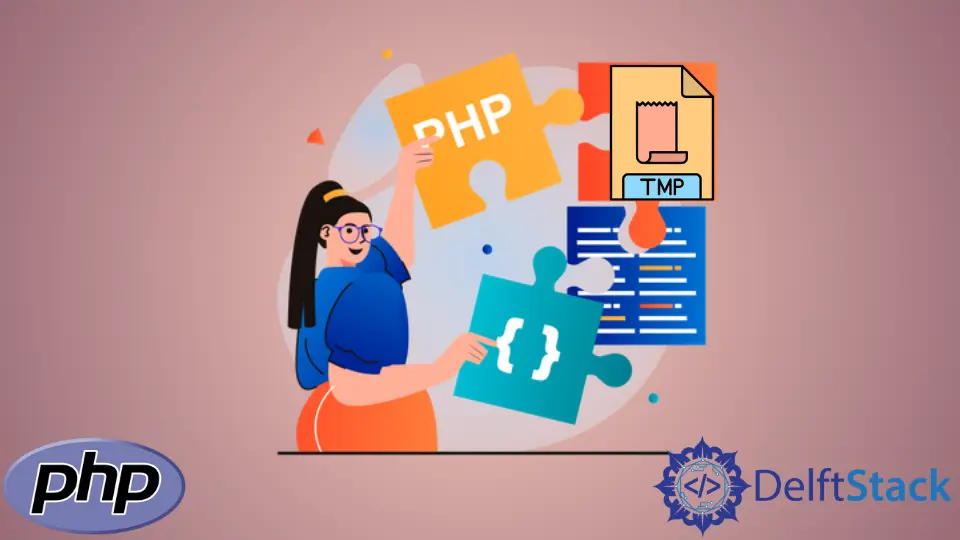
This article will introduce methods to create temporary files and get their full path in PHP.
Use the stream_get_meta_data()
Function and uri
Index to Create and Get the Path of tmpfile
in PHP
Temporary files are the files that hold the information temporarily while the program executes. Once the script or the program finishes its execution, the temporary file is deleted or transferred to a permanent file.
We can create temporary files in PHP with the tmpfile()
function.
The temporary file created by the function has the read/write(w+) mode. It returns false in case of failure to make a temporary file.
We can use stream_get_meta_data()
to get the path of the temporary file. The function takes a parameter that can be streams or file pointers.
It receives the header or metadata from the parameter and returns an array. We can use the file pointer of the temporary file created by the tmpfile()
function and the uri
index to return the temporary file path.
For example, create a temporay file with the tmpfile()
function and store it in the $file
variable. Next, use the stream_get_meta_data()
function with $file
as the parameter.
Assign it to the $temp_path
variable. Finally, display the variable using the uri
index.
As a result, we can see the temporary file’s location.
$file = tmpfile();
$temp_path = stream_get_meta_data($file);
echo $temp_path['uri'];
Output:
/tmp/phpQ8Y7V5
Use the tempnam()
and sys_get_temp_dir()
Functions to Create and Get the Path of tmpfile
in PHP
We can also use the tempnam()
function to create a temporary file in PHP. Using this function, we can give a unique name to the file.
The function takes two parameters. The first parameter specifies the directory where the temporary file is to be created, and the second is the filename prefix.
We can use the sys_get_temp_dir()
function as the first parameter to the tempnam()
function. The sys_get_temp_dir()
function returns the default directory where PHP stores the temporary file.
As a result, a temporary file will be created in the default directory. We can get the temporary file path by displaying the tempnam()
function with echo
.
For example, create a variable $temp_path
and assign it with the tempnam()
function. Write the sys_get_temp_dir()
function as the first parameter to the function and php
as the prefix.
Next, use the echo
function to print the $temp_path
variable.
Consequently, the path of the temporary file is printed.
$temp_path = tempnam(sys_get_temp_dir(), 'php');
echo $temp_path;
Output:
/tmp/phpujBAL3
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn