How to Check if a String Contains a Substring in PHP
-
Use the
strpos()
Function to Check if a String Contains a Substring in PHP -
Use the
preg_match()
Function to Check if a String Contains a Substring in PHP
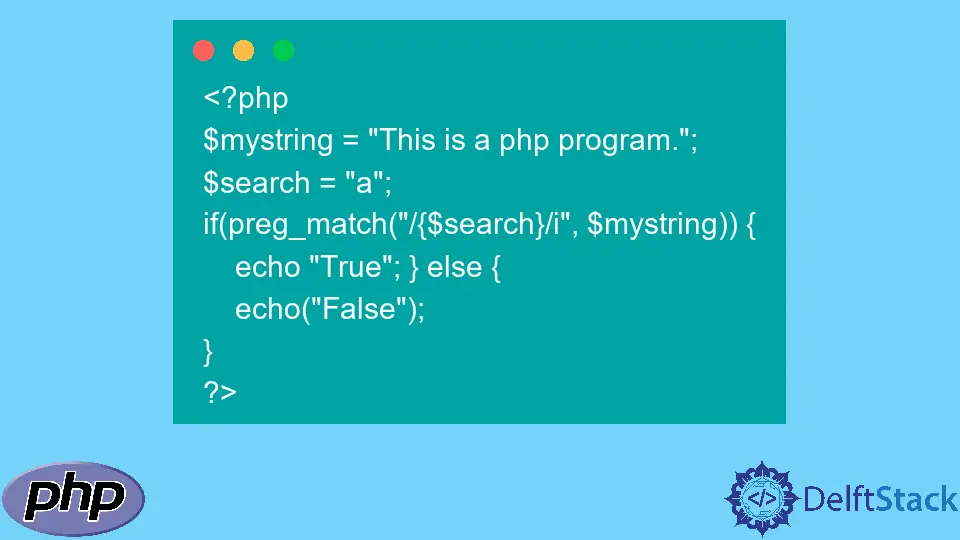
This article will introduce different methods to check if a string contains a substring in PHP using the strpos()
and preg_match()
functions.
Use the strpos()
Function to Check if a String Contains a Substring in PHP
We will use the built-in function strpos()
to check if a string contains a substring. This command checks the occurrence of a passed substring; the correct syntax to execute this is as follows:
strpos($originalString, $checkString, $offset);
The built-in function strpos()
has three parameters, which are discussed below:
Parameters | Description | |
---|---|---|
$originalString |
mandatory | It’s the string that we want to check for the substring. |
$checkString |
mandatory | It’s the string that we want to check in the $checkString . |
$offset |
optional | It’s the start position of the search process; if the offset is negative, the search process will start from the end. |
This function returns the position of the substring relative to the position of the original string. The program below shows how we can use the strpos()
function to check if a string contains a substring.
<?php
$mystring = "This is a PHP program.";
if (strpos($mystring, "program.") !== false) {
echo("True");
}
?>
Output:
True
If we pass the $offset
parameter, the function will start the search from the specified location.
<?php
$mystring = "This is a PHP program.";
if (strpos($mystring, "PHP", 13) !== false) {
echo("True");
} else {
echo("False");
}
?>
Output:
False
The output has shown False
because the string that we searched was before the offset.
Use the preg_match()
Function to Check if a String Contains a Substring in PHP
In PHP, we can also use the preg_match()
function to check if a string contains a specific word. This function uses regular expressions to match patterns. The correct syntax to use this function is as follows:
preg_match($pattern, $inputString, $matches, $flag, $offset);
The function preg_match()
accepts five parameters. The detail of its parameters is as follows
Parameters | Description | |
---|---|---|
$regexPattern |
mandatory | It is the pattern which we will search in the original string. |
$string |
mandatory | It is the string that we will search in the original string. |
$matches |
optional | This parameter stores the result of the matching process in it. |
$flag |
optional | This parameter specifies the flags. We have two flags for this parameter: PREG_OFFSET_CAPTURE and PREG_UNMATCHED_AS_NULL . |
$offset |
optional | This parameter tells about the start position of the matching process. |
We will use the pattern /{$search}/i
to check for a specific word. The program that checks if a string contains a specific word is as follows:
<?php
$mystring = "This is a php program.";
$search = "a";
if(preg_match("/{$search}/i", $mystring)) {
echo "True"; } else {
echo("False");
}
?>
Output:
True