How to Start and Stop a Timer in PHP
-
Understanding the
microtime()
Function - Starting a Timer
- Stopping a Timer
- Practical Applications of Timers in PHP
- Conclusion
- FAQ
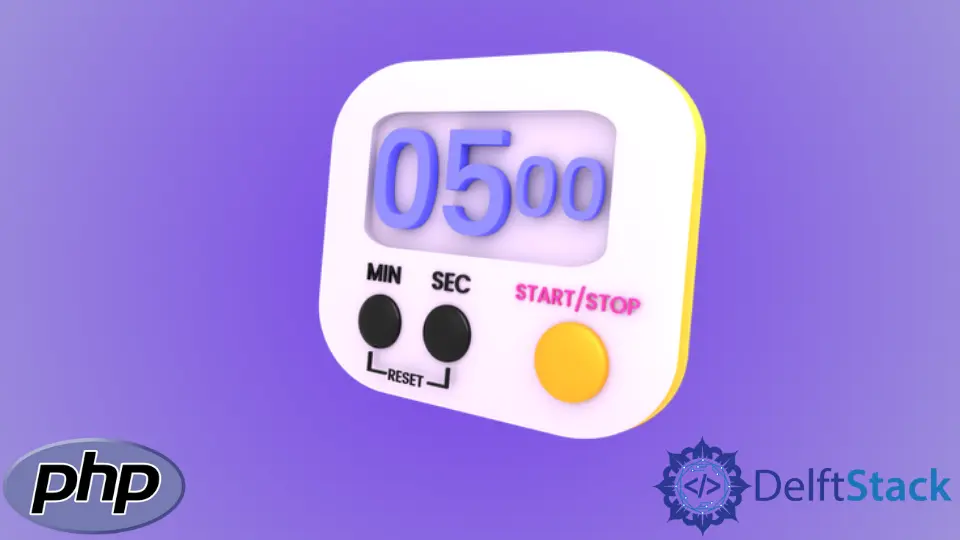
In the world of programming, timing can be crucial. Whether you’re measuring the performance of a script or simply tracking how long a process takes, knowing how to start and stop a timer in PHP can be incredibly useful.
This article will guide you through the process using PHP’s powerful microtime()
function. By the end, you’ll be able to implement timers in your own projects with ease. We’ll cover practical examples and provide clear explanations to help you grasp the concept fully. So, let’s dive in and learn how to effectively manage time in PHP!
Understanding the microtime()
Function
The microtime()
function in PHP is a handy tool for measuring time intervals. It returns the current Unix timestamp with microseconds, which allows for high-resolution timing. This is particularly useful when you need to measure the execution time of scripts or specific functions within your code.
To get started, you can call microtime()
with the optional parameter set to true
. This returns the current time as a float, making it easier to perform calculations. If you call it without parameters, it returns a string representation of the current time.
Let’s look at how to use this function to start and stop a timer.
Starting a Timer
To start a timer, you simply call microtime()
and store its result in a variable. This will mark the beginning of your timing. Here’s a simple example:
$startTime = microtime(true);
In this code, we are capturing the current time in seconds since the Unix epoch (January 1, 1970). The true
parameter allows us to get a float value, which is essential for precise calculations later.
Once you have the start time, you can execute the code block you want to measure. After the block is executed, you’ll stop the timer.
Stopping a Timer
Now that you’ve started a timer, the next step is to stop it. This is done in a similar way to starting it. You call microtime()
again and store the result in another variable. Here’s how to do it:
$endTime = microtime(true);
Now, you can calculate the elapsed time by subtracting the start time from the end time:
$elapsedTime = $endTime - $startTime;
This will give you the total time taken in seconds. To see the result, you can use the echo
statement:
echo "Elapsed time: " . $elapsedTime . " seconds";
Putting it all together, here’s a complete example that starts a timer, simulates a process, and then stops the timer:
$startTime = microtime(true);
// Simulated process
usleep(500000); // Sleep for half a second
$endTime = microtime(true);
$elapsedTime = $endTime - $startTime;
echo "Elapsed time: " . $elapsedTime . " seconds";
Output:
Elapsed time: 0.5 seconds
In this example, we used usleep()
to simulate a process that takes time. The timer starts before the sleep call and stops right after. The elapsed time is then printed out. This approach can be applied to any block of code where you want to measure execution time.
Practical Applications of Timers in PHP
Using timers in PHP can have various applications. For instance, if you’re developing a web application and want to optimize performance, measuring how long certain queries take can help you identify bottlenecks.
You might also want to time how long it takes for users to complete a specific action on your website. This could be useful for analytics or improving user experience.
Another example is in background tasks or cron jobs, where you may need to log how long a task takes to execute for monitoring purposes. By implementing timers, you can gather valuable data that can lead to better performance and user satisfaction.
Here’s a more detailed example that incorporates error handling and logging:
$startTime = microtime(true);
try {
// Simulated process
if (rand(1, 10) > 5) {
throw new Exception("An error occurred");
}
usleep(300000); // Simulate a task
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
$endTime = microtime(true);
$elapsedTime = $endTime - $startTime;
echo "Elapsed time: " . $elapsedTime . " seconds";
Output:
Elapsed time: 0.3 seconds
In this example, we simulate a process that could potentially throw an error. The timer still tracks the execution time, even if an error occurs. This is a great way to ensure that you have performance metrics regardless of the outcome of your process.
Conclusion
Starting and stopping a timer in PHP using the microtime()
function is straightforward and incredibly useful for various applications. Whether you’re measuring script performance, tracking user actions, or logging the execution time of background tasks, understanding how to implement timers can greatly enhance your PHP projects. By following the examples provided in this article, you can easily integrate timing functionality into your applications. So, get started today and see how timing can improve your PHP development!
FAQ
-
How accurate is the microtime() function in PHP?
The microtime() function provides high-resolution time measurements, accurate to microseconds, making it suitable for performance testing. -
Can I use microtime() to measure time in a web application?
Yes, microtime() is ideal for measuring execution time in web applications, such as tracking how long a page takes to load.
-
What happens if I forget to stop the timer?
If you forget to stop the timer, you won’t be able to measure the elapsed time accurately, which defeats the purpose of timing. -
Is there a built-in PHP function for profiling scripts?
PHP does not have a built-in profiling function, but you can use microtime() to create your own profiling tool. -
Can I use microtime() with other timing functions in PHP?
Yes, you can combine microtime() with other timing functions for more complex timing requirements.