PHP Spaceship Operator
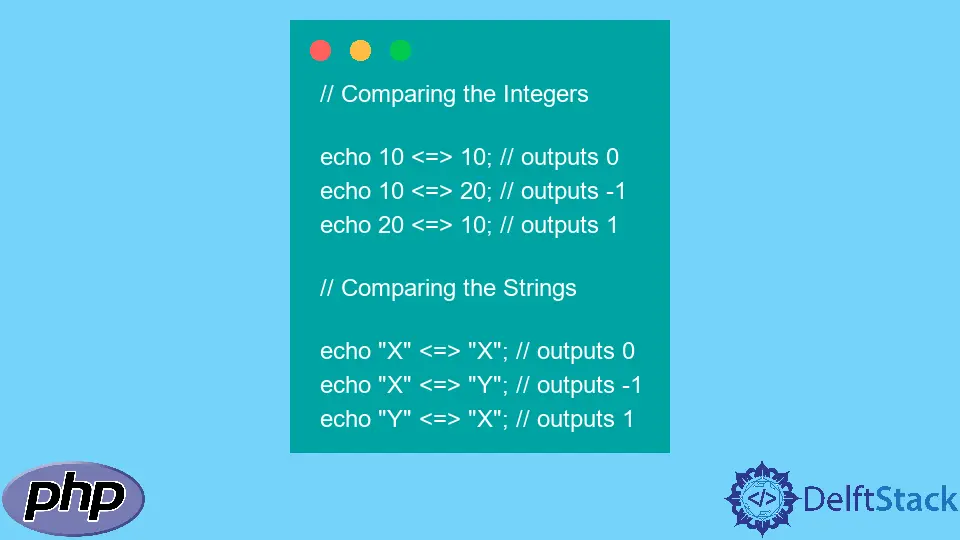
This tutorial demonstrates the spaceship operator in PHP.
PHP Spaceship Operator
The spaceship operator was introduced in PHP 7 and is denoted by the <=>
. The spaceship operator is considered a combined comparison operator.
The spaceship operator is a three-way operator used to perform a comparison based on greater than, less than, and equal to operations. The spaceship operator perform the same operations as the version_compare()
and strcmp()
methods.
The spaceship operator is used with the datatypes like integers, floats, strings, arrays, objects, etc. Here are some important points about the combined comparison of the <=>
operator.
- The spaceship operator will return
0
if the operands on both sides are equal. - The spaceship operator will return
1
if the operand on the left is a greater value. - The spaceship operator will return
-1
if the operand on the right is a greater value.
For example:
// Comparing the Integers
echo 10 <=> 10; // outputs 0
echo 10 <=> 20; // outputs -1
echo 20 <=> 10; // outputs 1
// Comparing the Strings
echo "X" <=> "X"; // outputs 0
echo "X" <=> "Y"; // outputs -1
echo "Y" <=> "X"; // outputs 1
Let’s try an example in PHP using the spaceship operator.
<?php
echo"The Spaceship Operator with Integer Values: <br>";
echo 10 <=> 10 ;
echo"<br>";
echo 10 <=> 20;
echo"<br>";
echo 20 <=> 10;
echo"<br>The Spaceship Operator with Float Values: <br>";
echo 10.5 <=> 10.5;
echo"<br>";
echo 10.5 <=> 20.5;
echo"<br>";
echo 20.5 <=> 10.5;
echo"<br>The Spaceship Operator with String Values: <br>";
echo "X" <=> "X" ;
echo"<br>";
echo "X" <=> "Y" ;
echo"<br>";
echo "Y" <=> "X" ;
echo"<br>The Spaceship Operator with Arrays: <br>";
echo [] <=> [];
echo"<br>";
echo [10, 20, 30] <=> [10, 20, 30];
echo"<br>";
echo [10, 20, 30, 40] <=> [10, 20, 30];
echo"<br>";
echo [10, 20, 30] <=> [40, 50, 60];
echo"<br>";
?>
The code above will use the spaceship operator on integers, floats, strings, and arrays to compare them. See the result:
The Spaceship Operator with Integer Values:
0
-1
1
The Spaceship Operator with Float Values:
0
-1
1
The Spaceship Operator with String Values:
0
-1
1
The Spaceship Operator with Arrays:
0
0
1
-1
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook