PHP Shortcode
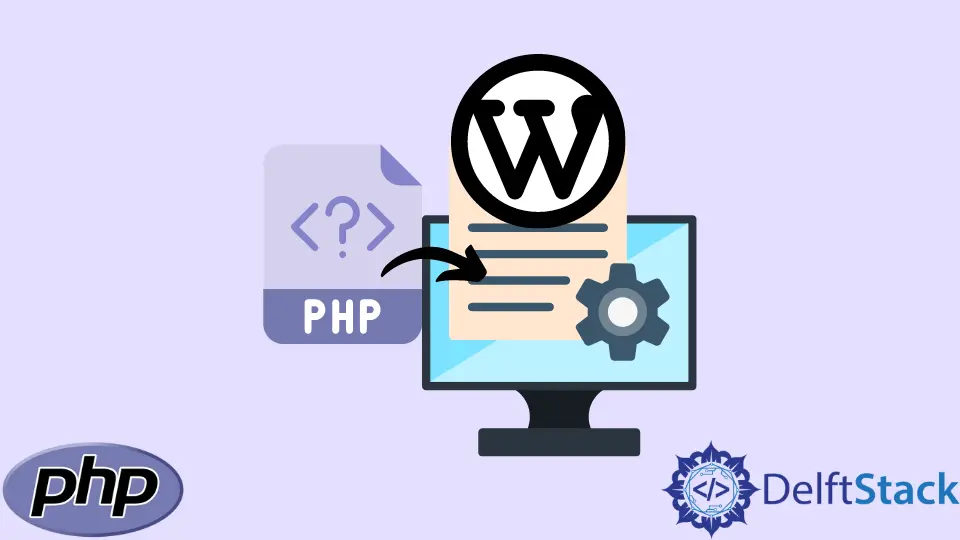
The shortcodes
are used to extend the site easily on WordPress. This tutorial demonstrates how to use shortcode
in PHP.
PHP Shortcode
The shortcodes
are used to use a component or functionality which is already developed. We can use that component of functionality anywhere we want in our PHP code in WordPress.
The syntax for applying the shortcode
in PHP is:
<?php echo do_shortcode( '[shortcode]' ); ?>
The shortcode
above will be the shortcode
of the component you want to include in the code. Let’s try to add a short code using WordPress and PHP.
Follow the steps below.
-
First of all, log in to WordPress or signup.
-
The next step is to install the plugin, for example, the
MetaSlider
. -
Once the plugin is installed, we can open the plugin from the right menu and find the
shortcode
underHow to Use
on the left side. Theshortcode
for this plugin is:[metaslider id = "9"]
-
Now, we can use this
shortcode
in the methoddo_shortcode()
to use the slider anywhere, mostly used in the headers.<?php echo do_shortcode( '[metaslider id = "9"]' ); ?>
-
The code above will show the slider on the position where it is written. The output for this slider is:
There are countless plugins out there, and many of them provide shortcodes
. We can use these shortcodes
in our codes to eliminate the large complex code structure.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook