The PHP shell_exec() and exec() Functions
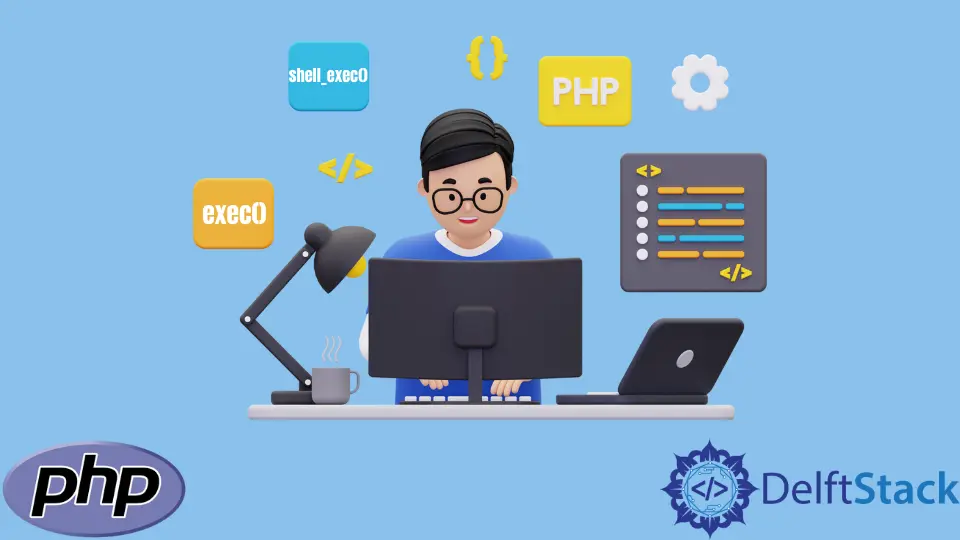
This article will discuss the PHP shell_exec()
and exec()
functions. While differentiating the two functions, we will see how each function performs and its practical use.
the PHP shell_exec()
Function
We use the shell_exec()
function to execute commands in the shell and return the output as a string. The shell_exec
is the alias for the backtick operator, *nix
.
Syntax:
string shell_exec( $cmd )
This function accepts one parameter, $cmd
. It contains the command to be executed.
In case of errors, the function returns NULL
.
It is important to note that the function does not run when PHP is in safe mode.
Example:
<?php
// Use ls command
$output = shell_exec('ls');
//List all files and directories
echo "<pre>$output</pre>";
?>
Output:
Insert.php
index.html
delft.php
the PHP exec()
Function
The exec()
function executes external programs and returns the last line of the output. If the command fails, it returns NULL
.
Syntax:
string exec( $command, $output, $return_var )
Parameters:
$command
contains the command to be executed.$output
specifies the array to be filled.$return_var
comes with theoutput
argument.
Example:
<?php
echo exec('iamexecfunction');
?>
Output:
delft.php
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn