PHP Session Encode Decode
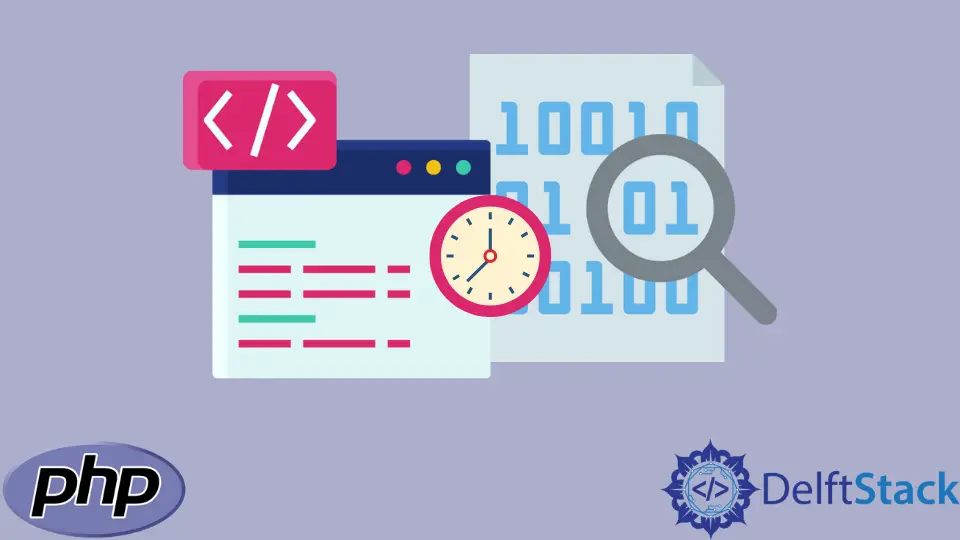
In PHP, the session is a way to handle data across web pages. The session encodes and decodes operations are the requirements while storing or reading data of the session.
Demonstrate the Use of session_encode()
and session_decode
in PHP
The built-in function session_encode()
serialize the $_SESSION
array data into an string and session_decode()
again convert the session data into the real format.
We insert the data through a form and then store it into a session:
test.php
:
<html>
<head>
<title> Demonstration of Session </title>
</head>
<body>
<form action="action.php" method="post" >
<div style="border: 4px solid;padding:10px; width:40%">
Employee Name:<input type="text" name="employee">
ID:<input type="text" name="id">
<input type="submit" value="SUBMIT" name="submit">
</div>
</form>
</body>
</html>
The session works like cookies; once we start the session and start storing the data, we can use it until the session is destroyed.
Output:
The encoded session string contains all the session elements separated by ;
. It should be mentioned here that this serialization is different from PHP serialize()
.
action.php
:
<?php
if (isset($_POST['submit']))
{
// Start the Session
session_start();
//Form Data
$employee=$_POST['employee'];
$id=$_POST['id'];
//store the form data into session
$_SESSION['employee']=$employee;
$_SESSION['id']=$id;
echo "According to the data from session: <br>";
echo "Hello ". $employee. "! your ID is ".$id."<br><br>";
echo"The encoded Session Data is: <br>";
//encode the session
$session_econded= session_encode();
echo $session_econded."<br><br>";
//decode session
session_decode($session_econded);
echo "Session data after decode: ";
print_r( $_SESSION);
//Destroy the Session
session_destroy();
}
?>
Output:
According to the data from session:
Hello Jack! your ID is 1234
The encoded Session Data is:
employee|s:4:"Jack";id|s:4:"1234";
Session data after decode: Array ( [employee] => Jack [id] => 1234 )
The encoded data is in the string form similar to PHP serialize()
.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook