How to Get URL Data With QUERY_STRING in PHP
Kevin Amayi
Feb 02, 2024
-
Use
$_SERVER['QUERY_STRING']
to Get Data Passed as Query Parameters in the URL in PHP -
Use
$_SERVER['QUERY_STRING']
to Get URL Data and Convert the Data to Array Using the Explode Function in PHP -
Use
$_SERVER['QUERY_STRING']
to Get URL Data, Convert Data to Array, Get the Individual Array Elements in PHP
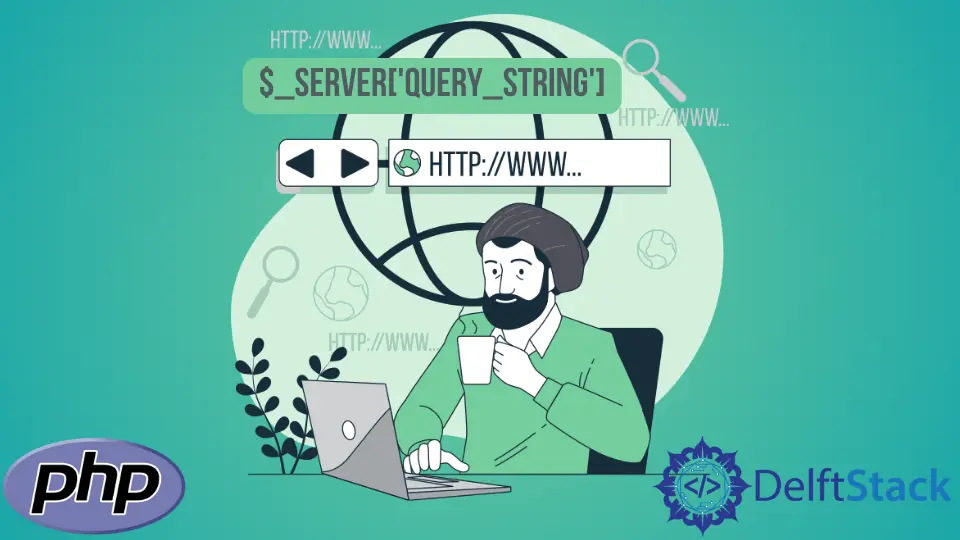
This article teaches how to use $_SERVER['QUERY_STRING']
to get data passed as query parameters in the URL, convert the data to an array, and get value at each index in PHP.
Use $_SERVER['QUERY_STRING']
to Get Data Passed as Query Parameters in the URL in PHP
We pass data as strings in the URL and capture the data using $_SERVER['QUERY_STRING']
and then print the strings.
<?php
$Q = $_SERVER['QUERY_STRING'];
var_dump($Q);
?>
URL:
http://localhost:2145/test2/hello.php?KevinAmayi/Programmer/Blogger/Athlete
Output:
string(37) "KevinAmayi/Programmer/Blogger/Athlete"
Use $_SERVER['QUERY_STRING']
to Get URL Data and Convert the Data to Array Using the Explode Function in PHP
We pass data as strings in the URL and capture the data using $_SERVER['QUERY_STRING']
, convert it to an array using the explode
function then print it.
<?php
$Q = explode("/", $_SERVER['QUERY_STRING']);
var_dump($Q);
?>
URL:
http://localhost:2145/test2/hello.php?KevinAmayi/Programmer/Blogger/Athlete
Output:
array(4) { [0]=> string(10) "KevinAmayi" [1]=> string(10) "Programmer" [2]=> string(7) "Blogger" [3]=> string(7) "Athlete" }
Use $_SERVER['QUERY_STRING']
to Get URL Data, Convert Data to Array, Get the Individual Array Elements in PHP
We pass data as strings in the URL and capture the data using $_SERVER['QUERY_STRING']
, convert it to an array using the explode
function then print the specific array elements.
<?php
$Q = explode('/',$_SERVER['QUERY_STRING']);
//get the first array element
echo $Q[0].'<br>';
//get the second array element
echo $Q[1].'<br>';
//get the third array element
echo $Q[2].'<br>';
echo $Q[3].'<br>';
?>
URL:
http://localhost:2145/test2/hello.php?KevinAmayi/Programmer/Blogger/Athlete
Output:
KevinAmayi
Programmer
Blogger
Athlete