How to Generate QR Code in PHP
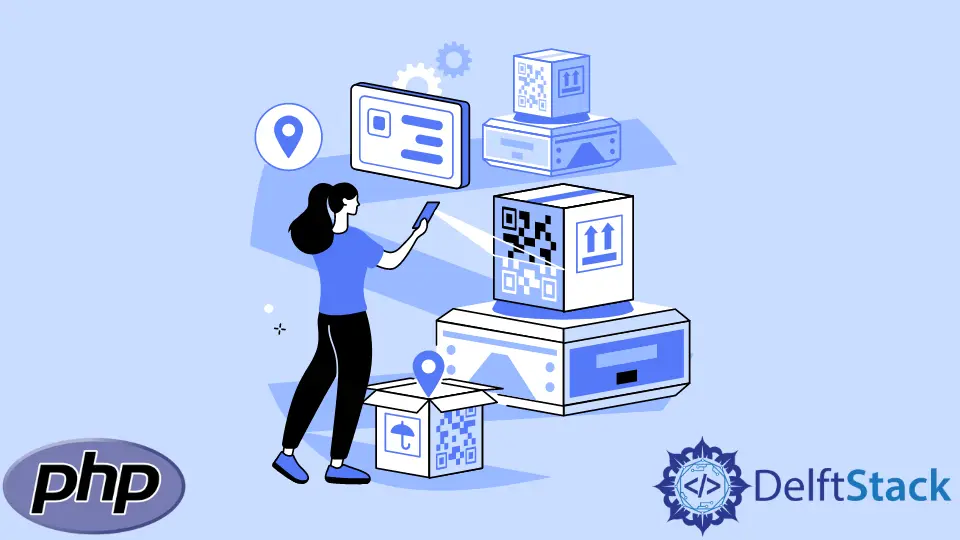
Creating QR codes in PHP can enhance user engagement and streamline processes in your applications. Whether you’re developing an e-commerce site, a mobile app, or simply want to share information quickly, QR codes are an excellent tool.
In this tutorial, we’ll explore how to generate QR codes using PHP with a straightforward approach. You’ll learn about various libraries and methods that can help you implement this functionality seamlessly. Let’s dive into the world of QR codes and see how you can integrate them into your PHP projects!
Understanding QR Codes
Before we jump into the code, let’s briefly understand what QR codes are. QR stands for Quick Response, and these codes can store URLs, text, and other types of data. Scanning a QR code with a smartphone camera or a QR code reader allows users to access the embedded information instantly. This makes them a popular choice for marketing materials, event tickets, and more.
Using the PHP QR Code Library
One of the most popular libraries for generating QR codes in PHP is the PHP QR Code library. It’s easy to use and provides various options for customization. Here’s how you can get started.
Step 1: Install the PHP QR Code Library
First, you need to download the library. You can do this using Composer, which is a dependency manager for PHP. If you don’t have Composer installed, you can download it from the official website.
Run the following command in your terminal:
composer require endroid/qr-code
This command will add the PHP QR Code library to your project.
Step 2: Generate a QR Code
Now that you have the library installed, let’s write some code to generate a QR code. Here’s a simple example:
<?php
require 'vendor/autoload.php';
use Endroid\QrCode\QrCode;
$qrCode = new QrCode('https://www.example.com');
$qrCode->setSize(300);
$qrCode->setMargin(10);
header('Content-Type: image/png');
echo $qrCode->writeString();
?>
Output:
A PNG image of the QR code linking to https://www.example.com
In this code, we first include the autoload file from Composer to load the library. We then create a new instance of the QrCode
class and pass the data we want to encode—in this case, a URL. The setSize
method allows you to define the dimensions of the QR code, while setMargin
sets the margin around the code. Finally, we set the content type to image/png
and output the QR code as a PNG image.
This method is straightforward and allows you to generate QR codes quickly. You can customize the size, margin, and even colors according to your needs.
Customizing QR Codes
One of the great features of the PHP QR Code library is its ability to customize QR codes. You can change colors, add logos, and modify the error correction level. Here’s how you can customize the QR code further.
Example of Customization
<?php
require 'vendor/autoload.php';
use Endroid\QrCode\QrCode;
use Endroid\QrCode\Writer\PngWriter;
$qrCode = new QrCode('https://www.example.com');
$qrCode->setSize(300);
$qrCode->setMargin(10);
$qrCode->setForegroundColor(['r' => 0, 'g' => 0, 'b' => 255]); // Blue color
$qrCode->setBackgroundColor(['r' => 255, 'g' => 255, 'b' => 255]); // White background
$writer = new PngWriter();
$writer->write($qrCode)->saveToFile('qrcode.png');
?>
Output:
A PNG image file named 'qrcode.png' with a blue QR code
In this code snippet, we set the foreground color of the QR code to blue and the background color to white using the setForegroundColor
and setBackgroundColor
methods. We then use the PngWriter
to save the QR code as a file named qrcode.png
. This customization allows you to create visually appealing QR codes that match your branding.
Conclusion
Generating QR codes in PHP is a straightforward process that can significantly enhance your application’s functionality. By using the PHP QR Code library, you can easily create, customize, and display QR codes for various purposes. Whether you choose to display them directly on your website or save them as images, the possibilities are endless. With this tutorial, you now have the knowledge to implement QR code generation in your PHP projects effectively.
FAQ
-
What is a QR code?
A QR code is a type of matrix barcode that can store information such as URLs, text, or other data, which can be scanned using a smartphone camera. -
Can I customize the appearance of my QR code?
Yes, you can customize the size, colors, and even add logos to your QR codes using the PHP QR Code library. -
Do I need to install any additional software to use the PHP QR Code library?
You need to have Composer installed to manage the library dependencies for your PHP project. -
Can QR codes be used for marketing purposes?
Absolutely! QR codes are widely used in marketing to link customers to websites, promotional content, and more. -
Is it possible to generate QR codes for different types of data?
Yes, you can generate QR codes for various data types, including URLs, text, contact information, and more.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn