How to Create a Popup Window in PHP
-
Use the
echo
Function to Display the JavaScript Popup in PHP -
Use the HTML Button and the JavaScript
onClick
Event to Display Popup in PHP
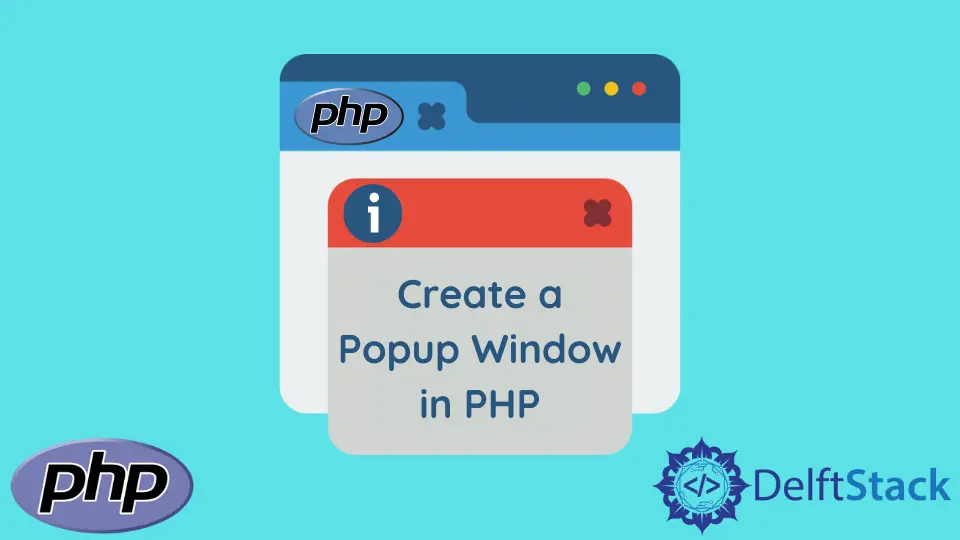
This article introduces ways to create a popup window in PHP with the help of JavaScript.
Use the echo
Function to Display the JavaScript Popup in PHP
In a PHP application, JavaScript comes in handy if we need to create a popup window. We can use the JavaScript alert()
method to achieve our goal.
The function creates an alert box on the webpage. We can write the JavaScript code in a PHP file inside the <script>
tag.
Finally, we can use the echo
statement to display the JavaScript function in PHP.
For example, create a PHP file and write the <script>
tag. Inside the <script>
tag, create a function displayMessage()
.
Next, use the alert()
method inside the displayMessage()
function and write some message in it.
In PHP, write the echo
function. Inside the quote of the echo
function, write the <script>
tag and invoke the displayMessage()
function.
As a result, an alert box will pop up when we run the PHP file.
JavaScript Code:
// index.php
<script>function displayMessage() {
alert('Hi, have a good day');
} < /script>
PHP Code:
//index.php
echo '<script>
displayMessage()
</script>
';
Output:
Use the HTML Button and the JavaScript onClick
Event to Display Popup in PHP
This method will introduce a way to create a popup window in PHP using the JavaScript onClick
. We can assign the onClick
event to an HTML button and invoke the alert()
method.
We can put all the code in a PHP file, host it on a server, and run it.
For example, create a PHP file index.php
and write the alert()
method with some message inside a JavaScript function displayMessage()
. Do not forget to wrap the JavaScript code inside the <script></script>
tags.
Next, create a button using the <input>
or the <button>
element. In the element, write the onClick
attribute and assign the displayMessage()
function to it.
After running the PHP file, a button will be displayed on the webpage that says Display alert box
.
When we click on the button, a window will pop up with a message.
JavaScript Code:
// index.php
<script>function displayMessage() {
alert('Hi, have a good day');
} < /script>
PHP Code:
//index.php
<input type="button" onclick="displayMessage()" value="Display alert box">
Output:
This way, we can create a popup window in PHP using the JavaScript alert()
method.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn