PHP Variables Pass by Reference
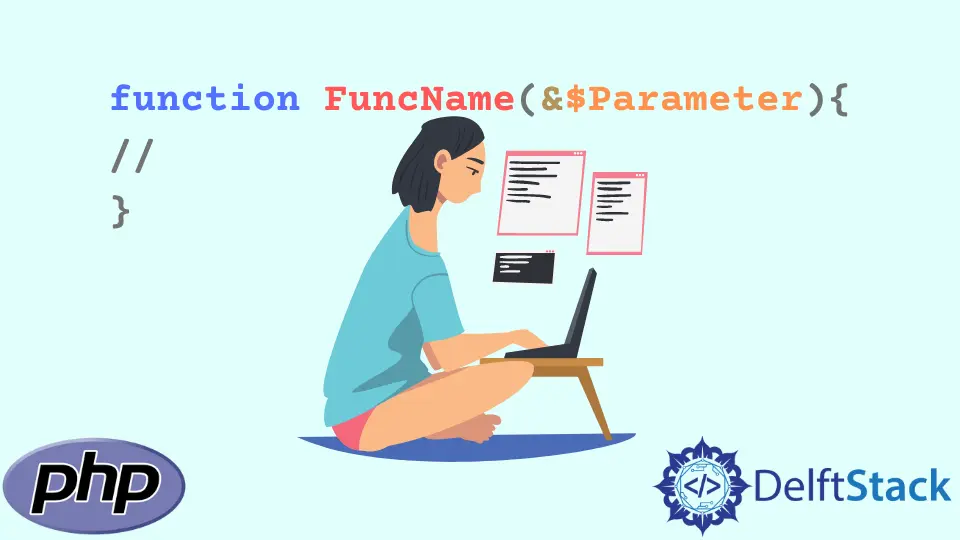
The variables are default passed to functions by value, but they can also be passed by reference in PHP. This tutorial demonstrates how to pass by references in PHP.
PHP Variables Pass by Reference
The ampersand symbol &
will be added to the beginning of the variable argument to pass the variables by reference in PHP. For example, function(&$a)
, where both global and function’s variable goal is to become global values because they are defined using the same reference concept.
Whenever the global variable changes, the variable inside the function will change and vice versa. The syntax for passing by reference is:
function FunctionName(&$Parameter){
//
}
Where FunctionName
is the function’s name, and Parameter
is a variable that will be passed by reference. Here is a simple example for passing by reference in PHP.
<?php
function Show_Number(&$Demo){
$Demo++;
}
$Demo=7;
echo "Value of Demo variable before the function call :: ";
echo $Demo;
echo "<br>";
echo "Value of Demo variable after the function call :: ";
Show_Number($Demo);
echo $Demo;
?>
The code above passes the variable Demo
by reference in the function Show_Number
. See output:
Value of Demo variable before the function call :: 7
Value of Demo variable after the function call :: 8
Let’s try another example to pass by reference with and without the ampersand symbol. See example:
<?php
// Assigning the new value to some $Demo1 variable and then printing it
echo "PHP pass by reference concept :: ";
echo "<hr>";
function PrintDemo1( &$Demo1 ) {
$Demo1 = "New Value \n";
// Print $Demo1 variable
print( $Demo1 );
echo "<br>";
}
// Drivers code
$Demo1 = "Old Value \n";
PrintDemo1( $Demo1 );
print( $Demo1 );
echo "<br><br><br>";
echo "PHP pass by reference concept but exempted ampersand symbol :: ";
echo "<hr>";
function PrintDemo2( $Demo2 ) {
$Demo2 = "New Value \n";
// Print $Demo2 variable
print( $Demo2 );
echo "<br>";
}
// Drivers code
$Demo2 = "Old Value \n";
PrintDemo2( $Demo2 );
print( $Demo2 );
echo "<br>";
?>
The code above creates two functions that are used to change the values of variables. When the variable is passed by reference with the ampersand symbol &
, the function is called simultaneously and changes the variable’s value.
Similarly, when passed by reference without the ampersand symbol &
, it will need to call the function to change the variable’s value. See output:
PHP pass by reference concept ::
New Value
New Value
PHP pass by reference concept but exempted ampersand symbol ::
New Value
Old Value
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook