How to Convert a Number to Month Name in PHP
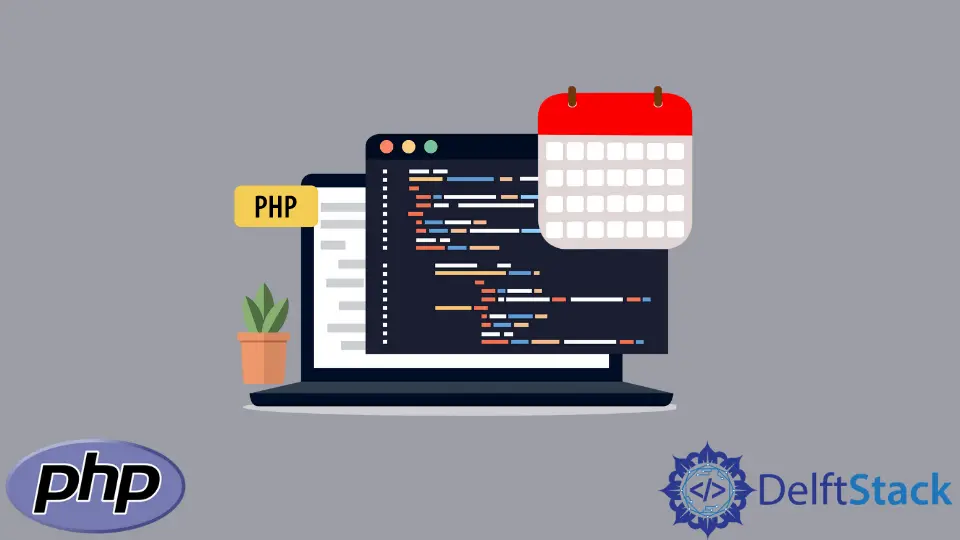
Converting a number to a month name in PHP is a common task that developers often encounter. Whether you’re working with user input or processing timestamps, knowing how to display the month name can enhance the user experience.
In this tutorial, we’ll explore two primary methods for achieving this: using the DateTime object and the date() function. We’ll provide clear examples and explanations to help you understand how to implement these techniques effectively. By the end of this article, you’ll be equipped with the skills to convert numbers to month names seamlessly in your PHP applications.
Method 1: Using the DateTime Object
The DateTime object in PHP is a powerful class that provides a rich set of methods for date and time manipulation. To convert a number to a month name using this object, you can create a DateTime instance and format it accordingly. Here’s how you can do it:
$monthNumber = 5; // Example month number
$date = DateTime::createFromFormat('!m', $monthNumber);
$monthName = $date->format('F');
echo $monthName;
Output:
May
In this example, we first define the month number we want to convert. The createFromFormat
method is used with the format string !m
, which specifies that we are providing a month in numeric form. The exclamation mark ensures that the month is treated as a standalone value. After creating the DateTime object, we use the format
method with the format character F
to retrieve the full textual representation of the month. This method is particularly useful because it allows you to easily manipulate and format dates in various ways beyond just retrieving month names.
Method 2: Using the date() Function
Another straightforward method to convert a number to a month name in PHP is by using the built-in date() function. This function allows you to format a timestamp into a readable date format, including month names. Here’s an example of how to use it:
$monthNumber = 3; // Example month number
$timestamp = mktime(0, 0, 0, $monthNumber, 1, 2000);
$monthName = date('F', $timestamp);
echo $monthName;
Output:
March
In this code, we start by defining the month number we want to convert. We then use the mktime
function to create a timestamp for the first day of that month in the year 2000. This is necessary because the date() function requires a valid timestamp to format. Finally, we call the date() function with the format string F
, which returns the full month name corresponding to the timestamp we created. This method is simple and effective, especially when you need to convert month numbers frequently in your PHP applications.
Conclusion
Converting a number to a month name in PHP can be easily accomplished using either the DateTime object or the date() function. Both methods provide a straightforward way to enhance your applications’ date handling capabilities. By implementing these techniques, you can ensure that your users receive clear and formatted date information, improving their overall experience. Whether you choose to use the DateTime class for its extensive capabilities or the simplicity of the date() function, you now have the knowledge to handle month name conversions confidently in your PHP projects.
FAQ
-
How do I convert a month number to a short month name in PHP?
You can use the same methods as above but change the format character toM
instead ofF
in the format function. -
Can I convert a month name back to a number in PHP?
Yes, you can use the array of month names to map back to numbers or use the DateTime object to parse the month name. -
What happens if I provide an invalid month number?
If you provide an invalid month number (like 0 or 13), the DateTime object will return false, so you should always validate input. -
Is there a performance difference between using DateTime and date() function?
Generally, the performance difference is negligible for most applications, but DateTime offers more features for complex date manipulations. -
Can I change the language of the month names in PHP?
Yes, you can use thesetlocale
function to change the locale, which will affect how month names are displayed.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn