Magic Quotes in PHP
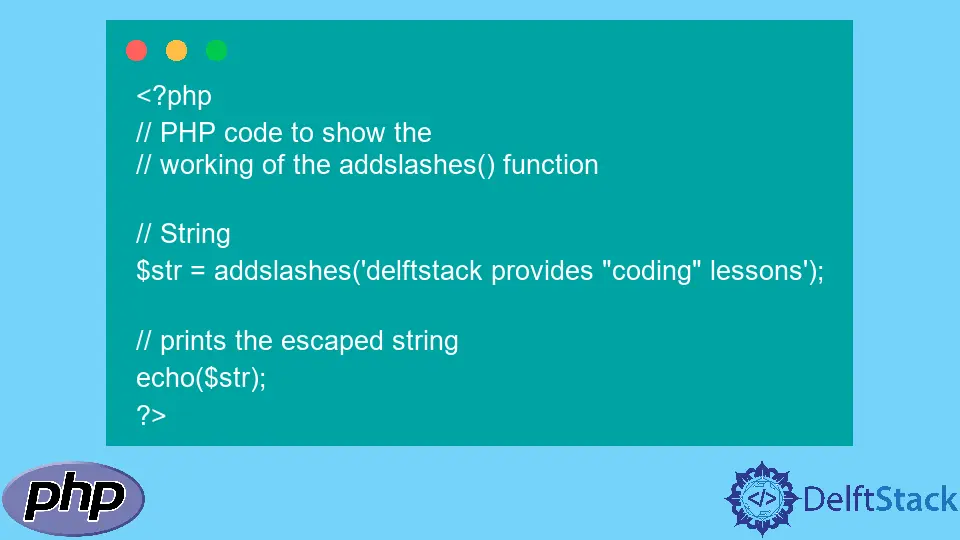
This article will discuss the addslashes()
function in PHP. It returns strings with backslashes before predefined characters.
Predefined characters include:
- Single quote
'
- Double quote
''
- Backslash
\
- Null
Magic Quotes in PHP
We can practically use this function when preparing strings for storage in databases and queries.
Before magic quotes in PHP were depreciated, the magic_quotes_gpc
was on by default. It used to run the addslashes()
function on $_GET/$_POST/$_COOKIES/$_REQUEST
by default.
We always check for already escaped strings. Running the addslashes()
function on such a string leads to double escaping.
Use the get_magic_quotes_gpc()
to verify if a string has been escaped.
Syntax:
addslashes(string)
The code below shows how the addslashes()
function works.
<?php
// PHP code to show the
// working of the addslashes() function
// String
$str = addslashes('delftstack provides "coding" lessons');
// prints the escaped string
echo($str);
?>
Output:
delftstack provides \"coding\" lessons
Example 2:
<?php
$str = "My name's Chris.";
echo $str . " This is not secure in a database query.<br>";
echo addslashes($str) . " This is secure in a database query.";
?>
Output:
My name's Chris. This is not secure in a database query.
My name\'s Chris. This is secure in a database query.
It is important to note that the addslashes()
function is different from the addcslashes()
function.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn