PHP Script in CSS Files
- Understanding the Basics of PHP and CSS
- Method 1: Inline CSS with PHP
- Method 2: Dynamic CSS Files
- Method 3: Using PHP to Include CSS Files
- Conclusion
- FAQ
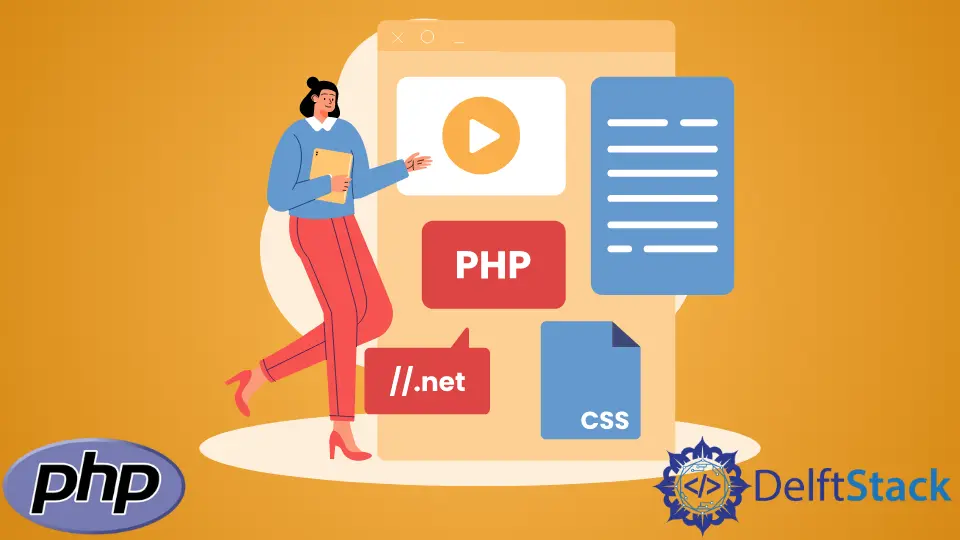
In the world of web development, integrating PHP with CSS can significantly enhance the styling and functionality of your websites.
This tutorial aims to demonstrate how to effectively use CSS stylesheets within PHP scripts. By leveraging PHP’s dynamic capabilities, you can create styles that adapt based on user input, database content, or other variables. Whether you are a seasoned developer or just starting, understanding how to combine these two powerful languages can elevate your projects. Join me as we explore different methods to implement CSS stylesheets in PHP scripts, ensuring your web pages are not only functional but also visually appealing.
Understanding the Basics of PHP and CSS
Before diving into the practical aspects of using PHP with CSS, it’s essential to grasp the fundamentals of both languages. PHP, a server-side scripting language, is designed to create dynamic web pages. It processes data on the server before sending it to the client, allowing for personalized experiences. On the other hand, CSS (Cascading Style Sheets) is used to style HTML elements, controlling layout, colors, fonts, and more. The combination of PHP and CSS allows developers to create responsive designs that can change based on various conditions.
Method 1: Inline CSS with PHP
One of the simplest ways to integrate CSS into PHP is by using inline styles. This method involves embedding CSS directly within PHP echo statements. Here’s a quick example:
<?php
$color = "blue";
echo "<div style='color: $color;'>This text is styled using PHP!</div>";
?>
Output:
This text is styled using PHP!
In this example, the variable $color
is defined in PHP and then used to set the text color of a <div>
element. This approach is straightforward but can become cumbersome for larger projects where styles are reused frequently. Inline styles are best suited for quick, one-off styling changes rather than comprehensive designs.
Method 2: Dynamic CSS Files
Another effective method is to create dynamic CSS files using PHP. This allows you to generate CSS rules based on variables or conditions. Here’s how you can achieve this:
<?php
header("Content-type: text/css; charset: UTF-8");
$background_color = "lightgray";
$text_color = "darkblue";
?>
body {
background-color: <?php echo $background_color; ?>;
color: <?php echo $text_color; ?>;
}
Output:
body {
background-color: lightgray;
color: darkblue;
}
In this example, the PHP script generates a CSS file. The header
function specifies the content type as CSS, while the CSS rules are dynamically generated based on PHP variables. This method is particularly useful for creating themes or styles that change based on user preferences or other conditions. By separating CSS from PHP logic, you maintain a cleaner codebase and improve maintainability.
Method 3: Using PHP to Include CSS Files
You can also include external CSS files in your PHP scripts. This method is beneficial for larger projects where styles are organized into separate files. Here’s an example of how to include a CSS file conditionally:
<?php
$theme = "dark"; // or "light"
if ($theme == "dark") {
echo '<link rel="stylesheet" type="text/css" href="dark-theme.css">';
} else {
echo '<link rel="stylesheet" type="text/css" href="light-theme.css">';
}
?>
Output:
<link rel="stylesheet" type="text/css" href="dark-theme.css">
In this code, PHP checks the value of the $theme
variable and includes the appropriate CSS file. This approach allows for easy switching between styles and is particularly useful for applications that offer user-selectable themes. By managing stylesheets in this way, you can enhance user experience without cluttering your PHP code.
Conclusion
Integrating CSS with PHP opens up a world of possibilities for web developers. Whether you choose to use inline styles, dynamic CSS files, or conditionally include stylesheets, each method has its advantages. Inline styles are quick and easy for minor adjustments, while dynamic CSS files allow for more extensive customization based on variables. Including external CSS files offers a clean and organized approach, especially for larger projects. By mastering these techniques, you can create visually stunning and highly functional web applications that engage users and enhance their experience.
FAQ
-
Can I use PHP variables in my CSS files?
Yes, you can use PHP to generate CSS dynamically by including PHP code within your stylesheets. -
Is it better to use inline CSS or external stylesheets?
For maintainability and organization, external stylesheets are generally preferred over inline CSS, especially for larger projects. -
How do I conditionally load different CSS files in PHP?
You can use PHP’s conditional statements to check certain criteria and include the appropriate CSS file based on those conditions. -
Can I use PHP to apply styles based on user preferences?
Absolutely! You can store user preferences in sessions or databases and use PHP to apply those styles dynamically. -
Are there performance implications when using PHP for CSS?
Generating CSS with PHP can add some server load, but if managed properly, it can enhance user experience without significant performance drawbacks.
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn