PHP Heredoc Syntax
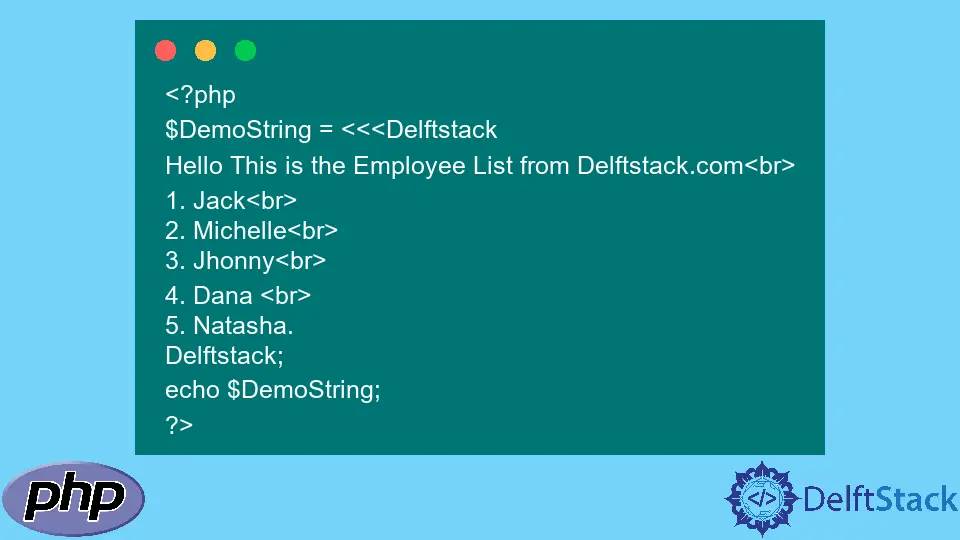
The heredoc syntax is used to declare strings in PHP. This tutorial describes and demonstrates the use of heredoc syntax in PHP.
PHP Heredoc Syntax
In PHP, mostly the programmers use two methods to declare strings, one with ' '
single quotes and the other with ""
double quotes, as demonstrated below.
$DemoString = 'Demo String';
$DemoString = "Demo String";
The heredoc syntax is another way to declare strings in PHP. The heredoc syntax takes at least three lines of code, and it uses the <<<
operator at the beginning to declare the string variable.
The syntax for this method is:
$DemoString = <<< identifier
// string
// string
// string
identifier;
The identifier
is used at the beginning and end of the syntax. You can use any word instead of an identifier
here.
Here are some important points when working with heredoc syntax.
- The heredoc variable must have at least three lines that begin with
<<<
and an identifier and ends with the same identifier. - The HTML tags can also be used in the heredoc syntax, which will be implemented as HTML elements.
- Never try to add functions and conditions in heredoc syntax. This will generate errors.
- To display other variables in the heredoc syntax, you can use curly braces
{}
.
Let’s try an example.
<?php
$DemoString = <<<Delftstack
Hello This is the Employee List from Delftstack.com<br>
1. Jack<br>
2. Michelle<br>
3. Jhonny<br>
4. Dana <br>
5. Natasha.
Delftstack;
echo $DemoString;
?>
The code above uses the heredoc syntax with the identifier Delftstack
. See output:
Hello This is the Employee List from Delftstack.com
1. Jack
2. Michelle
3. Jhonny
4. Dana
5. Natasha.
Here is another example with HTML tags and other variables.
<?php
$SiteName = "Delftstack";
$Message = "This is the best tutorials site for programmers";
$Print = <<<heredocDelftstack
<div >
<div >
<h1>{$SiteName}</h1>
<p>{$Message}</p>
</div>
</div>
heredocDelftstack;
echo $Print;
?>
The code above will show the data in HTML form. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook