How to Get Current Month Using Date() Function in PHP
-
Understanding the
Date()
Function - Formatting Options for Date()
- Practical Use Cases for Current Month
- Conclusion
- FAQ
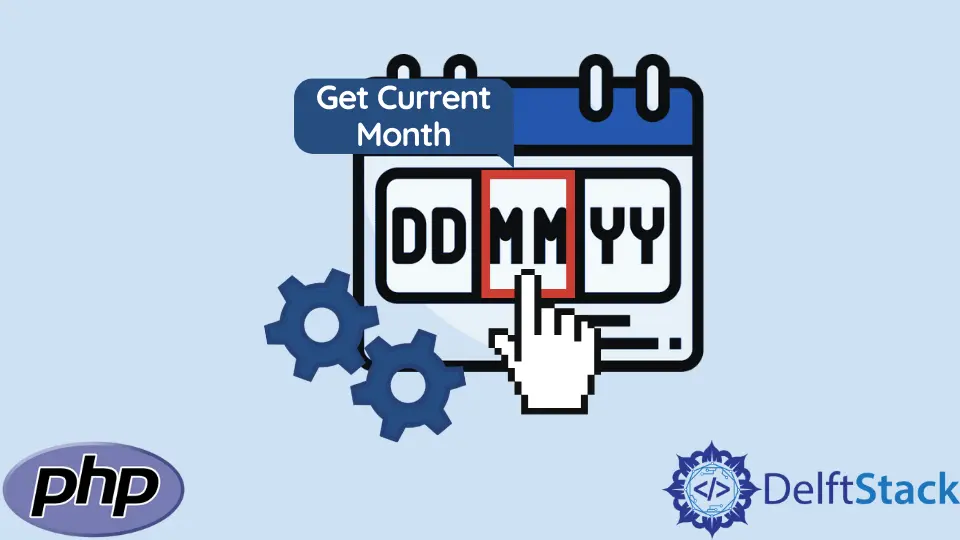
In PHP, working with dates is a common requirement for many developers. Whether you’re building a calendar application, a blog, or simply need to display the current month, the date()
function is your go-to tool. This function allows you to format dates in various ways, and retrieving the current month is straightforward.
In this tutorial, we will explore how to effectively get the current month using the date()
function in PHP. We will break down the process step by step, ensuring you have a clear understanding of how to implement this in your own projects. Let’s dive in!
Understanding the Date()
Function
The date()
function in PHP is a powerful tool for formatting dates and times. It takes two parameters: the format string and an optional timestamp. If no timestamp is provided, it defaults to the current time. To get the current month, you simply need to use the appropriate format character in the function.
Getting the Current Month
To retrieve the current month, you can use the format character m
or F
. The m
character returns the month in a two-digit format (01 to 12), while F
returns the full textual representation of the month (e.g., January, February, etc.). Here’s how you can implement this in your PHP code:
$currentMonthNumeric = date('m');
$currentMonthTextual = date('F');
echo "Current Month (Numeric): " . $currentMonthNumeric;
echo "\nCurrent Month (Textual): " . $currentMonthTextual;
Output:
Current Month (Numeric): 10
Current Month (Textual): October
In this example, we first retrieve the current month in numeric format and store it in the variable $currentMonthNumeric
. We then retrieve the full month name and store it in $currentMonthTextual
. Finally, we display both values using the echo
statement. This simple yet effective approach allows you to present the current month in whichever format suits your needs.
Formatting Options for Date()
When using the date()
function, you have a variety of formatting options at your disposal. Depending on your requirements, you might want to customize how the month is displayed. Here are some common formatting options you can use:
- Numeric Month: As mentioned, use
m
for a two-digit representation. - Full Month Name: Use
F
to get the full name of the month. - Abbreviated Month Name: Use
M
for a three-letter abbreviation (e.g., Jan, Feb).
Here’s an example that demonstrates these formatting options:
$monthNumeric = date('m');
$monthFullName = date('F');
$monthAbbreviation = date('M');
echo "Numeric Month: " . $monthNumeric;
echo "\nFull Month Name: " . $monthFullName;
echo "\nAbbreviated Month: " . $monthAbbreviation;
Output:
Numeric Month: 10
Full Month Name: October
Abbreviated Month: Oct
In this code, we retrieve the month in three different formats and display them. This flexibility allows developers to choose the most appropriate format for their application. Whether you need a simple numeric representation or a more descriptive name, the date()
function has you covered.
Practical Use Cases for Current Month
Understanding how to get the current month can be beneficial in various scenarios. Here are a few practical use cases:
- Dynamic Content Display: If you’re building a website that showcases monthly promotions or events, using the current month dynamically ensures your content is always relevant.
- Data Filtering: When querying databases, filtering records based on the current month can help in generating reports or analytics.
- User Notifications: For applications that send reminders or notifications, knowing the current month allows you to schedule these actions effectively.
Consider this example where you might want to display a message based on the current month:
$currentMonth = date('F');
if ($currentMonth == 'December') {
echo "Happy Holidays!";
} else {
echo "Welcome to " . $currentMonth;
}
Output:
Welcome to October
In this example, we check if the current month is December and display a special message if it is. Otherwise, we welcome the user to the current month. This simple logic can enhance user engagement by providing timely messages.
Conclusion
In this tutorial, we explored how to get the current month using the date()
function in PHP. We covered various formatting options and practical use cases that demonstrate the importance of retrieving the current month dynamically. Whether you’re developing a web application or a simple script, understanding how to work with dates can significantly enhance your project’s functionality. Remember to experiment with the date()
function and see how it can fit into your coding needs.
FAQ
-
How do I get the current year in PHP?
You can use thedate('Y')
function to get the current year in a four-digit format. -
Can I get the current month in different languages?
PHP’sdate()
function does not support localization directly. You can create an array of month names in different languages and retrieve the appropriate month based on the current month number. -
What if I need the month from a specific date?
You can use thestrtotime()
function to convert a date string into a timestamp and then usedate()
to format it. For example,date('m', strtotime('2023-10-01'))
will return ‘10’. -
Is the
date()
function timezone sensitive?
Yes, thedate()
function uses the server’s default timezone. You can set a specific timezone usingdate_default_timezone_set('Timezone')
before calling the function. -
Can I format a date in PHP other than the current date?
Absolutely! You can pass a specific timestamp as the second parameter to thedate()
function to format any date.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn