How to Check foreach Loop Key Value in PHP
Minahil Noor
Feb 02, 2024
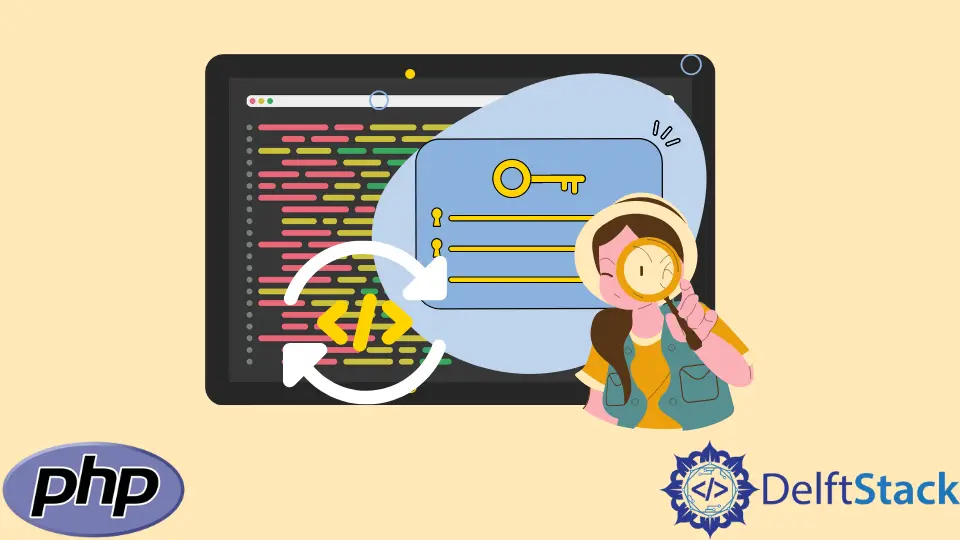
This article will introduce a method to check the foreach
loop key value in PHP.
Use the foreach
Loop to Check the foreach
Loop Key Value in PHP
We can use a foreach
loop to check the foreach
loop key value. The foreach
loop traverses through an array whose keys and elements can be accessed in the loop. The correct syntax to use this loop is as follows.
foreach($arrayName as $variableName){
//PHP code
}
If we have an associative array, we can use this loop in the following way:
foreach($arrayName as $key => $variableName){
//PHP code
}
The detail of its parameters is as follows:
Variable | Detail | |
---|---|---|
$arrayName |
mandatory | This is the array we want to traverse. |
$variableName |
mandatory | It is the variable name for array elements. |
$key |
optional | It is the variable name for keys of the array. |
The foreach
loop stops when it traverses the whole array.
We can use the echo()
function to display the array keys.
The program below shows how we can use foreach
loop to check foreach
loop key value in PHP.
<?php
$result = array("firstname"=>"Olivia", "lastname"=>"Mason", "marks"=>85, "attendance"=>100);
echo("The value of keys of the array are:\n");
foreach ($result as $key => $value)
{
echo($key);
echo("\n");
}
?>
We have looped through the array and displayed its keys.
Output:
The value of keys of the array are:
firstname
lastname
marks
attendance