How to Escape Quotation in PHP
Minahil Noor
Feb 02, 2024
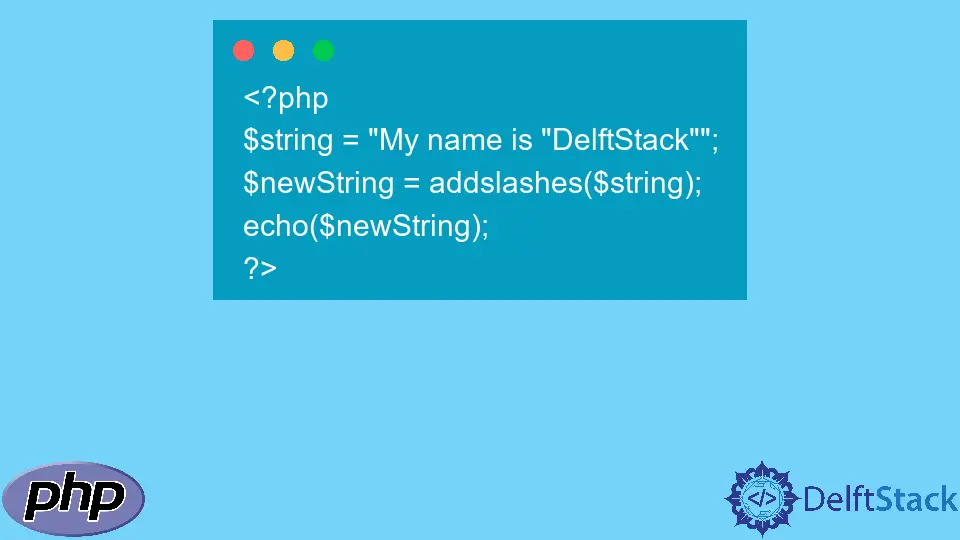
This article will introduce different methods to escape quotation in PHP.
Use the Backslash to Escape Quotation in PHP
Whenever we use quotation marks inside a string, the PHP compiler shows an error. To avoid this error, we will use backslash \
to escape it. The correct syntax to use backslash is as follows.
$mystring = "\"\"";
The output will be ""
.
The program below shows the way by which we can use the backslash \
to escape quotation marks in PHP.
<?php
echo("My name is \"DelftStack\".");
Output:
My name is "DelftStack".