How to Document Root in PHP
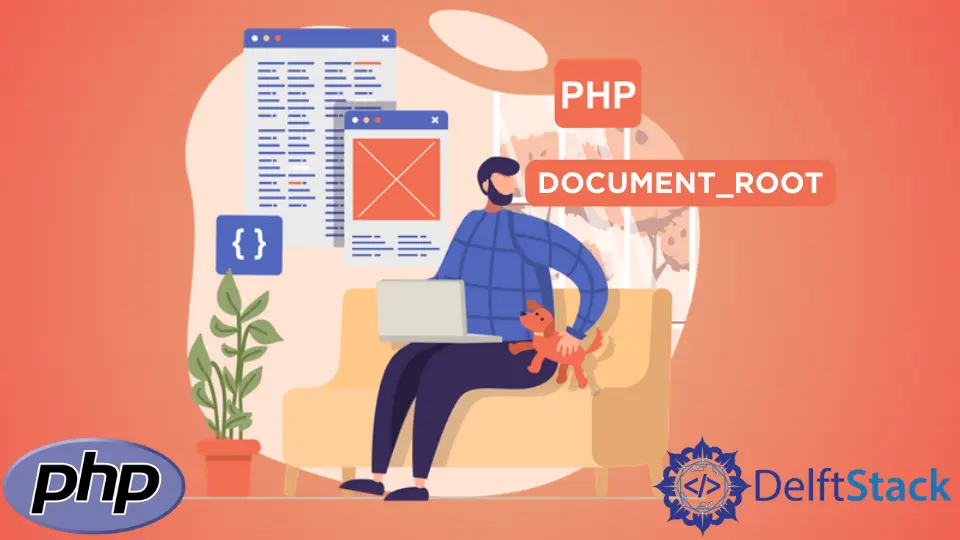
Your PHP script may need the root directory name in which the script is currently executing. This article will introduce how to get the directory name of the current script inside the project.
$_SERVER
in PHP
$_SERVER
is an array that contains information about headers, paths, and script locations. The webserver creates all this information. You can use an array to get details of a specific location, like PHP_SELF
, SCRIPT_NAME
, DOCUMENT_ROOT
, etc.
DOCUMENT_ROOT
in PHP
It’s not advisable to set the static path for any file because the change of root path will lead to a failure if the path inside the script is not changed. $_SERVER
contains information about the document root directory under which the current script is executing. It is accessible via the variable DOCUMENT_ROOT
, as defined in the server’s configuration file. This is the path where your application code is stored.
The main difference between DOCUMENT_ROOT
& /
in HTML file is that it first renders an actual file path & later renders the root of the server URL. So if you want a file path relative to the server, you need to add $_SERVER['DOCUMENT_ROOT']./'helloworld.html'
but if you want it relative to your website, just use /helloworld.html
.
Example code:
<?php
echo $_SERVER['DOCUMENT_ROOT'];
?>
Output:
C:/xampp/www/
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn