File Download With CURL in PHP
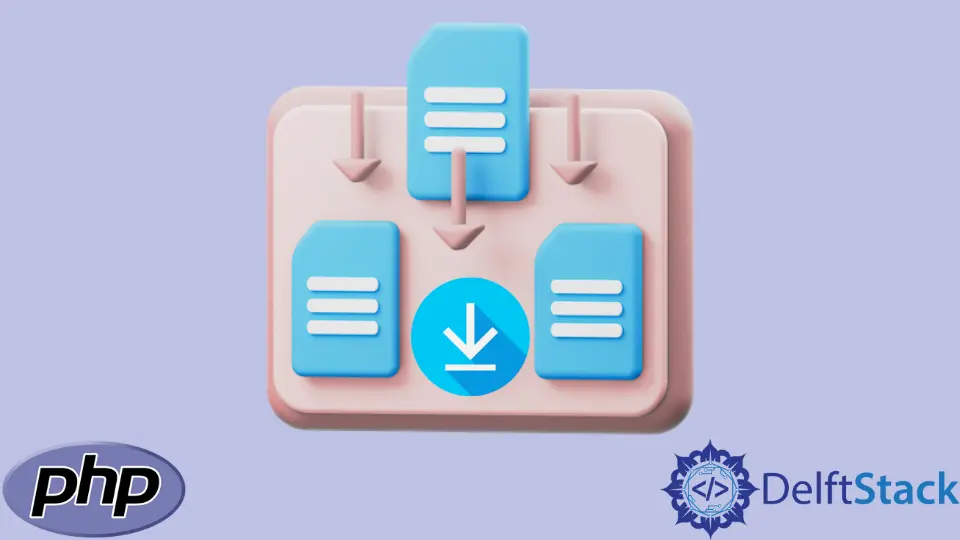
The -output
or -o
command is used to download files with cURL in PHP.
We can download a file with cURL by giving the URL to the file. cURL library is used for many purposes in PHP.
Use the cURL
to Download a File From a Given URL in PHP
First of all, make sure cURL is enabled in your PHP. You can check it by running phpinfo()
and enabling it from the php.ini
file.
We are trying to download the files from the local server to the local server itself.
<?php
// File download information
set_time_limit(0); // if the file is large set the timeout.
$to_download = "http://localhost/delftstack.jpg"; // the target url from which the file will be downloaded
$downloaded = "newDelftstack.jpg"; // downloaded file url
// File Handling
$new_file = fopen($downloaded, "w") or die("cannot open" . $downloaded);
// Setting the curl operations
$cd = curl_init();
curl_setopt($cd, CURLOPT_URL, $to_download);
curl_setopt($cd, CURLOPT_FILE, $new_file);
curl_setopt($cd, CURLOPT_TIMEOUT, 30); // timeout is 30 seconds, to download the large files you may need to increase the timeout limit.
// Running curl to download file
curl_exec($cd);
if (curl_errno($cd)) {
echo "the cURL error is : " . curl_error($cd);
} else {
$status = curl_getinfo($cd);
echo $status["http_code"] == 200 ? "The File is Downloaded" : "The error code is : " . $status["http_code"] ;
// the http status 200 means everything is going well. the error codes can be 401, 403 or 404.
}
// close and finalize the operations.
curl_close($cd);
fclose($new_file);
?>
Output:
The output shows that the code can download a file from a given URL. To download the large files, we can increase the timeout limit.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook