PHP UTF-8 Conversion
-
Use
utf8_encode()
andutf8_decode()
to Encode and Decode Strings in PHP -
Use
iconv()
to Convert a String to UTF-8
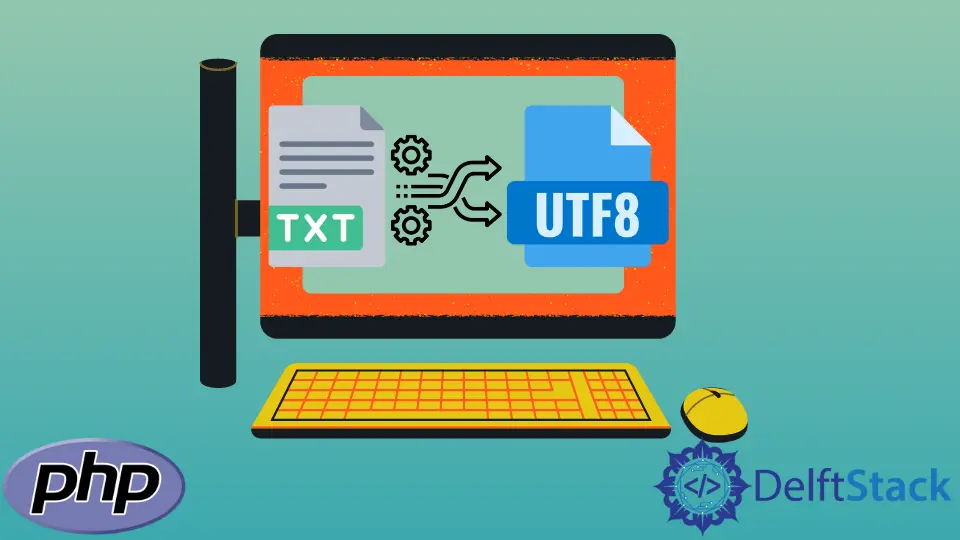
The UTF-8
is a way to encode Unicode characters, each character in between one to four bytes.
It is used to handle the special character or characters from languages other than English.
PHP has different ways to convert text into UTF-8
.
Use utf8_encode()
and utf8_decode()
to Encode and Decode Strings in PHP
Both utf8_encode()
and utf8_decode()
are built-in functions in PHP.
It is used to encode and decode ISO-8859-1
, and other types of strings to UTF-8
, both of these function takes a string as a parameter.
See the example below:
<?php
$demo="\xE0\xE9\xED"; //ISO-8859-1 String àéí
echo "UTF-8 Encoded String: ";
echo utf8_encode($demo) ."<br>";
echo "UTF-8 Decoded String: ";
echo utf8_decode(utf8_encode($demo)) ."<br>";
echo "UTF-8 Encoded String from the decoded: ";
echo utf8_encode(utf8_decode(utf8_encode($demo))) ."<br>";
?>
The code above encodes an ISO-8859-1
string to UTF
and then decodes the output again. The input string you see is with ISO-8859-1
encoding.
Output:
UTF-8 Encoded String: àéí
UTF-8 Decoded String: ���
UTF-8 Encoded String from the decoded: àéí
The utf8_decode()
converts a string with ISO-8859-1
characters encoded with UTF-8
to single-byte ISO-8859-1
.
When reading an ISO-8859-1
encoded text as UTF-8
, you will often see that question mark.
Use iconv()
to Convert a String to UTF-8
iconv()
is another built-in PHP function used to convert string from one Unicode.
It takes three parameters, one is the string’s Unicode, the second is the Unicode you want to convert, and the third is the string itself.
See the example below:
<?php
$demo="\xE0\xE9\xED"; //ISO-8859-1 String àéí
echo "The UTF-8 String is: ";
echo iconv("ISO-8859-1", "UTF-8", $demo)."<br>";
//mb_detect_encoding() is a function used to detect encoding of the given text.
echo "The UTF-8 String with auto detection is: ";
echo iconv(mb_detect_encoding($demo, mb_detect_order(), true), "UTF-8", $demo);
?>
The code above takes three parameters and converts the text to UTF-8
.
Output:
The UTF-8 String is: àéí
The UTF-8 String with auto detection is: àéí
PHP also offers other functions like recode_string()
or mb_convert_encoding()
, which works similarly to iconv
; they convert a string to the requested Unicode.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook